/* * AHPA #8: The C ATM Machine * * Two people, "a" and "b", have checking and savings accounts. * * Create a 2x2 array to hold their amounts: a – checking: $500, savings: * $1,000; b – checking: $750, savings: $325. * * Have the ATM machine ask the user their name. * * Ask the user if they want to withdraw from their checking or their * savings account. * * Use a switch statement to process different types of accounts. * * Create software that will provide an ATM user with the proper change * for any dollar amount up to $200. ( C PROGRAMMING LANGUAGE) * * Example: Run the code for $19, $55, and $200. * */ #include #include #include #include #include int main(void) { return 0; } (PLEASE WITH COMMENT AND OUTPUT SHOULD BE SHOWN IN PICTURE BELOW)
/*
* AHPA #8: The C ATM Machine
*
* Two people, "a" and "b", have checking and savings accounts.
*
* Create a 2x2 array to hold their amounts: a – checking: $500, savings:
* $1,000; b – checking: $750, savings: $325.
*
* Have the ATM machine ask the user their name.
*
* Ask the user if they want to withdraw from their checking or their
* savings account.
*
* Use a switch statement to process different types of accounts.
*
* Create software that will provide an ATM user with the proper change
* for any dollar amount up to $200. ( C
*
* Example: Run the code for $19, $55, and $200.
*
*/
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <time.h>
int main(void) {
return 0;
}
(PLEASE WITH COMMENT AND OUTPUT SHOULD BE SHOWN IN PICTURE BELOW)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

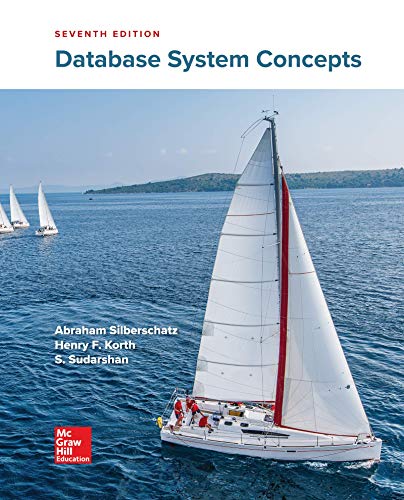
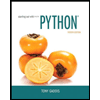
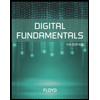
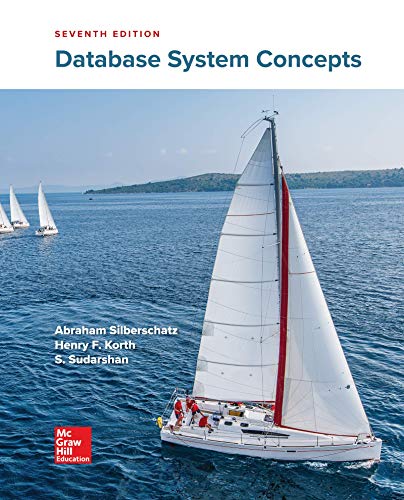
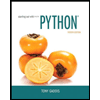
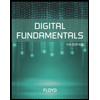
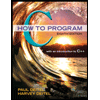
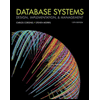
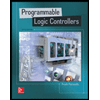