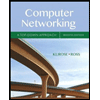
- Add the following operation to the Class StackClass: void reverseStack(StackClass<T> otherStack);
This operation copies the elements of a stack in reverse order onto another stack.
Consider the following statements:
StackClass<T> stack1;
StackClass<T> stack2;
The statement
stack1.reverseStack(stack2);
copies the elements of stack1 onto stack2 in the reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed and stack1 is unchanged.
Write the definition of the method to implement the operation reverseStack. Also write a program to test the method reverseStack.
In StackClass.java, please finish method - public void reverseStack(StackClass<T> otherStack) and testing main program – Problem53.java.
Note: java programming, please use given outline code

Step by stepSolved in 2 steps

- don't use others answers java 1. Write a generic static method that takes a Stack of any type element as a parameter, pops each element from the stack, and prints it. It should have a type parameter that represents the Stack’s element type.arrow_forwardStack ADT Implement a program in C++ that has the following three parts and each does the following: 1. Implements in C++, the specification of a Stack ADT with all the Main and Helper behaviors (save the file as StackADT.h) 2. Implements in C++, all the necessary methods (behavior) of the StackADT (save the file as StackADT.cpp) 3. Implements in C++, a main function (the client or application program) that creates and uses Stack objects (save the file as StackMain.cpp). This main function should be able to do the following: a. It should be able to read a given text file as input, b. It should be able to get each input value from the input file (one word at a time) and store it (push) on the StackADT object until it stores all the words from the input file. c. It should be able to retrieve an item from the stack and 1) display it on the screen, as well as 2) write it to an output file, and then delete (pop) that item from the stack, and repeat this process until it retrieves all the…arrow_forwardModify the Java class for the abstract stack type shown belowto use a linked list representation and test it with the same code thatappears in this chapter. class StackClass {private int [] stackRef;private int maxLen,topIndex;public StackClass() { // A constructorstackRef = new int [100];maxLen = 99;topIndex = -1;}public void push(int number) {if (topIndex == maxLen)System.out.println("Error in push–stack is full");else stackRef[++topIndex] = number;}public void pop() {if (empty())System.out.println("Error in pop–stack is empty"); else --topIndex;}public int top() {if (empty()) {System.out.println("Error in top–stack is empty");return 9999;}elsereturn (stackRef[topIndex]);}public boolean empty() {return (topIndex == -1);}}An example class that uses StackClass follows:public class TstStack {public static void main(String[] args) {StackClass myStack = new StackClass();myStack.push(42);myStack.push(29);System.out.println("29 is: " + myStack.top());myStack.pop();System.out.println("42 is:…arrow_forward
- Java Implement Stack using Deque (doubly linked list) You must create an array and the user can insert elements into this array and can only access or remove the newly inserted element from the array. The array is executed using a doubly linked list. The following Project should have these classes: 1. Class Book: The main Node for the deque array where it should have the following attributes besides (next, prev nodes): a) Book Id b) Book Name c) Book Author 2. Class Booklists: Where all the main operations are done. You need to apply these following operations: 1) AddBook() [push(0) : The method Inserts the book object into deque Stack (form the last). 2) RemoveBook() [pop()] : This method extracts an object from the last of the Deque stack and it removes it. If such object does not exist, the method returns null.(from the last) 3) isEmpty() : Return True if deque stack is Empty else return False. 4) DisplayAlIBooks() : Print all the books in the deque stack. 5) getlistsize(): Return…arrow_forwardAssume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forwardAnswer in 5 mins else downvotearrow_forward
- in c++ language pleasearrow_forwardsolution for subpart 3arrow_forwardProject Overview: This project is for testing the use and understanding of stacks. In this assignment, you will be writing a program that reads in a stream of text and tests for mismatched delimiters. First, you will create a stack class that stores, in each node, a character (char), a line number (int) and a character count (int). This can either be based on a dynamic stack or a static stack from the book, modified according to these requirements. I suggest using a stack of structs that have the char, line number and character count as members, but you can do this separately if you wish.Second, your program should start reading in lines of text from the user. This reading in of lines of text (using getline) should only end when it receives the line “DONE”.While the text is being read, you will use this stack to test for matching “blocks”. That is, the text coming in will have the delimiters to bracket information into blocks, the braces {}, parentheses (), and brackets [ ]. A string…arrow_forward
- java /* Practice Stacks and ourVector Write a java program that creates a stack of integers. Fill the stack with 30 random numbers between -300 and +300. A)- With the help of one ourVector Object and an additional stack, reorganize the numbers in the stack so that numbers smaller than -100 go to the bottom of the stack, numbers between -100 and +100 in the middle and numbers larger than +100 in the top (order does not matter) B)- (a little harder) Solve the same problem using only one ourVector object for help C)- (harder) Solve the same problem using only one additional stack as a helper */ public class HWStacks { public static void main(String[] args) { // TODO Auto-generated method stub } }arrow_forwardOCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction -Command mul refers to integer multiplication -Command div refers to integer division -Command rem refers to the remainder of integer division -Command neg is to calculate the negation of an integer -Command swap interchanges the top two elements in the stack, meaning that the…arrow_forwardsolution for subpart 4arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
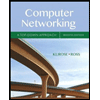
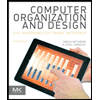
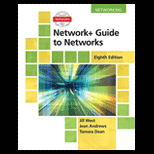
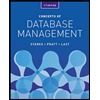
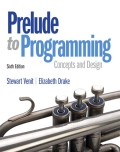
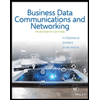