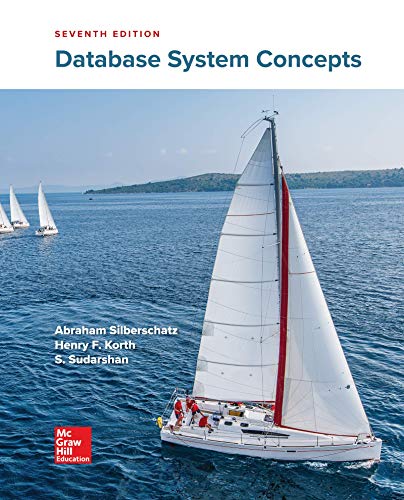
Program this in java:
a. I input an array of integers: int[] arr = { 4, 100, 2, 50, 7, -45, -23, 84, 51, 120, 98, -72 }.
b. Declare an integer variable that named "total".
c. create a loop.
d.1. The loop control variable value to be declared is 0. This will be represented by the variable "i" and it represents the index of the inputted array.
e.2. The loop has a condition that if the current loop control variable value is greater than or equal to the size of the array minus the first item of the array, the loop will break.
f.3. After the body or function is executed, the loop control variable is incremented by 2.
g.4. The body of the loop has a conditional statement. if arr[i] is even, add it to total, else minus it to the total.
g. After the loop breaks or finishes, display the value of the total.

Step by stepSolved in 3 steps with 1 images

- This is needed in JAVAarrow_forwardLanguage is C++ Lab10B: Binary Bubbles. Binary search is a very fast searching algorithm, however it requires a set of numbers to be sorted first. For this lab, create an array full of 11 integers which the user will generate. Like in the previous lab, assume that the values will be between -100 and +100. Then, using the sorting algorithm called BubbleSort, put the array in the correct order (from lowest to highest number). After this, please printthe array to the screen. Finally, search the array for the target value using Binary Search.The BinarySearch code will implement the algorithm described in the lecture slides. During this, you should print out a few key values which help Binary Search function. For example, this algorithm focuses on a low, mid, and high which correspond to the indices in the array the algorithm is currently considering and searching. Printing these values during the search process will help with debugging and fixing any issues. • BubbleSort sorts the array…arrow_forwardIn C Programming Write a main function that declares an array of 100 ints. Fill the array with random values between 1 and 100. Calculate the average of the values in the array. Output the average.arrow_forward
- I want this work be done in C# Visual studio. Given the following: private void btnRun_Click(object sender, EventArgs e){//Your code goes here } Code the statement(s) that will create a integer array of 5 elements and initialize the array with the following values in one line of code 10, 15, 20, 25, and 30. Then using a for loop alter the values in each element of the array by adding 5 to it. So after the for loop runs the values of the array should be 15, 20, 25, 30, and 35.arrow_forwardC++arrow_forwardin C programming Write a main function that declares an array of 100 doubles.In a for loop, assign each of the doubles a random number between 0.50 and 50.00. Here’s how.array[i] = (double) (rand() % 100 + 1) / 2.0;Output the elements of the array in 10 columns that are each 6 spaces wide. Each row in the output will have 10 values. The doubles will be printed with 2 places of accuracy past the decimal.The output of this one-dimensional array requires a single loop with an if statement inside. Even though the 100 numbers are going to be presented as a table of numbers, they are still just a list in memory.arrow_forward
- In C#, how would you compile a program that declares a 5 element double array that uses a loop to read values for each element from the user. The program should then use an additional loop to compute and output the sum of the elements in the array.arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardc++ language using just one for looparrow_forward
- In this project you will generate a poker hand containing five cards randomly selected from a deck of cards. The names of the cards are stored in a text string will be converted into an array. The array will be randomly sorted to "shuffle" the deck. Each time the user clicks a Deal button, the last five cards of the array will be removed, reducing the size of the deck size. When the size of the deck drops to zero, a new randomly sorted deck will be generated. A preview of the completed project with a randomly generated hand is shown in Figure 7-50.arrow_forwardHello, Stuck on this question. All programming is in Python. 2.) Design a program that generates a 7-digit lottery number. The program should have an INTEGER array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array. All programming is in Python.arrow_forwardCreate a Console application that does the following:This program must use a single array to store integers and find the maximum value.1. Create an array and initialize it internally with the values 5, 8, 9, 4, and 2.2. Use a loop to iterate through the array and determine the maximum value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
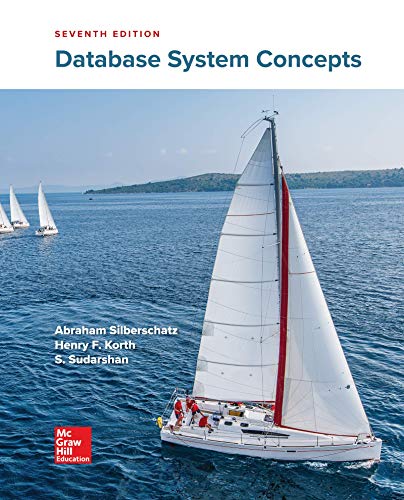
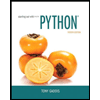
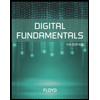
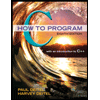
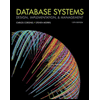
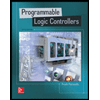