A retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent and the county sales tax rate is 2 percent. Write a program that asks the user to enter the total sales for the month. The application should calculate and display the following: The amount of county sales tax The amount of state sales tax The total sales tax (county plus state) Step 3: Complete the pseudocode by writing the missing lines. (Reference: Defining and Calling a Module, page 78-81). Also, when writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module. (Reference: Passing Arguments by Value and by Reference, page 97 – 103). Module main () //Declare local variables Declare Real totalSales ______________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ //Function calls Call inputData(totalSales) Call calcCounty(totalSales, countyTax) ______________________________________________________ ______________________________________________________ ______________________________________________________ End Module //this module takes in the required user input Module inputData(Real Ref totalSales) Display “Enter the total sales for the month.” Input totalSales End Module //this module calculates county tax //totalSales can be a value parameter because it is not //changed in the module. //countyTax must be a reference parameter because it is //changed in the module Module calcCounty(Real totalSales, Real Ref countyTax) countyTax = totalSales * .02 End Module //this module calculates state tax Module ____________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ End Module //this module calculates total tax Module ____________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ End Module //this module prints the total, county, and state tax Module ____________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ ______________________________________________________ End Module
A retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent and the county sales tax rate is 2 percent. Write a program that asks the user to enter the total sales for the month. The application should calculate and display the following:
- The amount of county sales tax
- The amount of state sales tax
- The total sales tax (county plus state)
Step 3: Complete the pseudocode by writing the missing lines. (Reference: Defining and Calling a Module, page 78-81). Also, when writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module. (Reference: Passing Arguments by Value and by Reference, page 97 – 103).
Module main ()
//Declare local variables
Declare Real totalSales
______________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
//Function calls
Call inputData(totalSales)
Call calcCounty(totalSales, countyTax)
______________________________________________________
______________________________________________________
______________________________________________________
End Module
//this module takes in the required user input
Module inputData(Real Ref totalSales)
Display “Enter the total sales for the month.”
Input totalSales
End Module
//this module calculates county tax
//totalSales can be a value parameter because it is not
//changed in the module.
//countyTax must be a reference parameter because it is
//changed in the module
Module calcCounty(Real totalSales, Real Ref countyTax)
countyTax = totalSales * .02
End Module
//this module calculates state tax
Module ____________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
End Module
//this module calculates total tax
Module ____________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
End Module
//this module prints the total, county, and state tax
Module ____________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
______________________________________________________
End Module

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

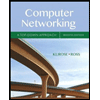
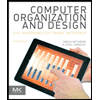
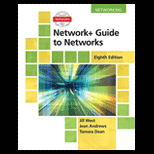
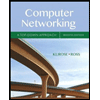
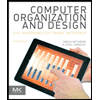
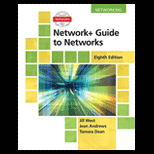
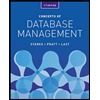
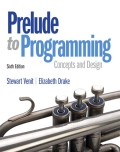
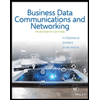