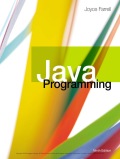
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
- A private int data field named accountNum for the account (default 0).
- A private string data field named accountName for the account (default "none").
- A private double data field named balance for the account (default 0).
- A private int data field named numTransactions that will count the number of deposit and withdraw transactions made to an account.
- A private Date data field named dateCreated that stores the date when the account was created.
- A no-arg constructor that creates a default account.
- A constructor that creates an account with the specified account number and name.
- The accessor and mutator methods for account number, name.
- The accessor method for balance and dateCreated.
- A method named withdraw that withdraws a specified amount from the account.
- A method named deposit that deposits a specified amount to the account.
- A method named displayAccountInfo that displays all the information in the Account object.
- A method named combine that takes another Account object as an argument and adds up balances and number of transactions to current Account object.
package assignment2;
public class Test {
public static void main(String[] args) {
Account account = new Account(1122, "my checking account");
account.deposit(3000);
account.withdraw(2500);
account.deposit(800);
account.withdraw(200);
account.displayAccountInfo();
if (account.getBalance() != 1100) {
System.out.println("Something went wrong! " + account.getAccountName() + " should have 1100 CAD balance.");
}
System.out.println();
Account accountSavings = new Account(1133, "my savings account");
accountSavings.deposit(10000);
accountSavings.deposit(2000);
accountSavings.displayAccountInfo();
System.out.println();
account.combine(accountSavings);
account.setAccountNum(1190);
account.setAccountName("my all money at the bank");
account.displayAccountInfo();
}
}
"Test.java" and below output is expected:
Account num: 1122
Account name: my checking account
Balance: 1100.0 CAD
4 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account num: 1133
Account name: my savings account
Balance: 12000.0 CAD
2 transactions since created at Thu Jul 06 12:55:21 PDT 2023
Account num: 1190
Account name: my all money at the bank
Balance: 13100.0 CAD
6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account name: my checking account
Balance: 1100.0 CAD
4 transactions since created at Thu Jul 06 12:55:20 PDT 2023
Account num: 1133
Account name: my savings account
Balance: 12000.0 CAD
2 transactions since created at Thu Jul 06 12:55:21 PDT 2023
Account num: 1190
Account name: my all money at the bank
Balance: 13100.0 CAD
6 transactions since created at Thu Jul 06 12:55:20 PDT 2023
USE PDT time, makes sure the code is right
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The Inventory class contains a Private variable named strId. The variable is associated with the Public ItemId property. An application instantiates an Inventory object and assigns it to a variable named onHand. Which of the following can be used by the application to assign the string "XG45 to the strId variable? a. onHand.ItemId = "XG45" b. ItemId.strId = "XG45" c. onHand.strId = "XG45" d. ItemId.strId = "XG45"arrow_forwardCreate an application named SalesTransactionDemo that declares several SalesTransaction objects and displays their values and their sum. The SalesTransaction class contains fields for a salesperson name, sales amount, and commission and a readonly field that stores the commission rate. Include three constructors for the class. One constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value times commission rate. The second constructor accepts a name and sales amount, but sets the commission rate to 0. The third constructor accepts a name and sets all the other fields to 0. An overloaded + operator adds the sales values for two SalesTransaction objects.arrow_forwardAssume that you have created a class named TermPaper that contains a character field named letterGrade. You also have created a property for the field. Which of the following cannot be true? The property name is letterCrade. The property is read-only. The property contains a set accessor that does not allow a grade lower than 'C '. The property does not contain a get accessor.arrow_forward
- Each of the following files in the Chapter.08 folder of your downloadable student files has syntax and/or logical errors. In each case, determine the problem, and fix the program. After you correct the errors, save each file using the same filename preceded with Fixed. For example, DebugEight1.cs will become FixedDebugEight1.cs. a. DebugEight1.cs b. DebugEight2.cs c. DebugEight3.cs d. DebugEight4.csarrow_forwardIn this exercise, you modify the Grade Calculator application from this chapter’s Apply lesson. Use Windows to make a copy of the Grade Solution folder. Rename the copy Grade Solution-Intermediate. Open the Grade Solution.sln file contained in the Grade Solution-Intermediate folder. Open the CourseGrade.vb file. The DetermineGrade method should accept an integer that represents the total number of points that can be earned in the course. (Currently, the total number of points is 200: 100 points per test.) For an A grade, the student must earn at least 90% of the total points. For a B, C, and D grade, the student must earn at least 80%, 70%, and 60%, respectively. If the student earns less than 60% of the total points, the grade is F. Make the appropriate modifications to the DetermineGrade method and then save the solution. Unlock the controls on the form. Add a label control and a text box to the form. Change the label control’s Text property to “&Maximum points:” (without the quotation marks). Change the text box’s name to txtMax. Lock the controls and then reset the tab order. Open the form’s Code Editor window. The txtMax control should accept only numbers and the Backspace key. Code the appropriate procedure. The grade should be cleared when the user makes a change to the contents of the txtMax control. Code the appropriate procedure. Modify the frmMain_Load procedure so that each list box displays numbers from 0 through 200. Locate the btnDisplay_Click procedure. If the txtMax control does not contain a value, display an appropriate message. The maximum number allowed in the txtMax control should be 400; if the control contains a number that is more than 400, display an appropriate message. The statement that calculates the grade should pass the maximum number of points to the studentGrade object’s DetermineGrade method. Make the necessary modifications to the procedure. Save the solution and then start and test the application.arrow_forwardThe purpose of this exercise is to demonstrate the importance of testing an application thoroughly. Open the FixIt Solution.sln file contained in the VB2017\Chap04\FixIt Solution folder. The application displays a shipping charge that is based on the total price entered by the user, as shown in Figure 4-64. Start the application and then test it by clicking the Display shipping button. Notice that the Shipping charge box contains $13, which is not correct. Now, test the application using the following total prices: 100, 501, 1500, 500.75, 30, 1000.33, and 2000. Here too, notice that the application does not always display the correct shipping charge. (More specifically, the shipping charge for two of the seven total prices is incorrect.) Open the Code Editor window and correct the errors in the code. Save the solution and then start and test the application.arrow_forward
- Create an application class named LetterDemo that instantiates objects of two classes named Letter and CertifiedLetter and that demonstrates all their methods. The classes are used by a company to keep track of letters they mail to clients. The Letter class includes auto-implemented properties for the name of the recipient and the date mailed. Also, include a ToString() method that overrides the Object classs ToString() method and returns a string that contains the name of the class (using CetType and the Letters data field values. Create a child class named CertifiedLetter that includes an auto-implemented property that holds a tracking number for the letter.arrow_forwardWhich of the following is the name of the Inventory class’s default constructor? Inventory InventoryConstructor Default Newarrow_forwardWhich of the following statements is false? a. A class can contain only one constructor. b. An example of a behavior is the SetTime method in a Time class. c. An object created from a class is referred to as an instance of the class. d. An instance of a class is considered an object.arrow_forward
- The operator used to create objects is = += new createarrow_forwardAfter you have dragged a Button onto a Form in the IDE, you can double-click it to __________ delete it view its properties create a method that executes when a user clicks the Button increase its sizearrow_forwardWrite a GUI program named TestsInteractiveGUI that allows a user to enter scores for five tests he has taken. Display the average of the test scores to two decimal places.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE L
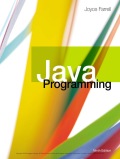
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
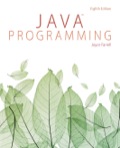
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
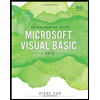
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
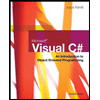
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
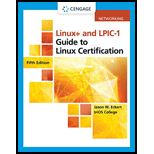
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L