A library wants to manage its collection of books and authors more efficiently. They would like to create a Python program to help with this task. The following requirements need to be implemented: Create an Author class with the attributes: name, dob (date of birth), and nationality. The class should have a __str__ method to represent the author in a user-friendly format. Create a Book class with the following attributes: title, author (an instance of the Author class), publication_date, and price. Also, include a class variable all_books that keeps a list of all book instances created. The Book class should have: A __str__ method to represent the book in a user-friendly format. A __eq__ method to compare two books. Two books are considered the same if they have the same title and author. A __lt__ method to compare two books based on their publication dates. A class method get_all_books that returns a list of all books in the library. A static method most_expensive_book that takes a list of book instances and returns the most expensive one. Finally, create a Library class that manages books and authors. This class should include the following: An attribute collection to store the books in the library. A method add_book to add a new book to the library. If the book already exists in the library (based on the __eq__ method from the Book class), it should not be added again. A method search_books_by_author to find all books written by a particular author. A method most_expensive_book that returns the most expensive book in the library's collection. Make sure to handle potential exceptions gracefully and provide informative error messages to the user. Your program should demonstrate the creation of multiple Author and Book objects, add these books to a Library object, and show usage of the search_books_by_author and most_expensive_book methods. Use the code provided in the first screenshot below to test your implementation. This code should go at the end of your program after you have satisfied all of the requirements above.
A library wants to manage its collection of books and authors more efficiently. They would like to create a Python program to help with this task. The following requirements need to be implemented: Create an Author class with the attributes: name, dob (date of birth), and nationality. The class should have a __str__ method to represent the author in a user-friendly format. Create a Book class with the following attributes: title, author (an instance of the Author class), publication_date, and price. Also, include a class variable all_books that keeps a list of all book instances created. The Book class should have: A __str__ method to represent the book in a user-friendly format. A __eq__ method to compare two books. Two books are considered the same if they have the same title and author. A __lt__ method to compare two books based on their publication dates. A class method get_all_books that returns a list of all books in the library. A static method most_expensive_book that takes a list of book instances and returns the most expensive one. Finally, create a Library class that manages books and authors. This class should include the following: An attribute collection to store the books in the library. A method add_book to add a new book to the library. If the book already exists in the library (based on the __eq__ method from the Book class), it should not be added again. A method search_books_by_author to find all books written by a particular author. A method most_expensive_book that returns the most expensive book in the library's collection. Make sure to handle potential exceptions gracefully and provide informative error messages to the user. Your program should demonstrate the creation of multiple Author and Book objects, add these books to a Library object, and show usage of the search_books_by_author and most_expensive_book methods. Use the code provided in the first screenshot below to test your implementation. This code should go at the end of your program after you have satisfied all of the requirements above.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- A library wants to manage its collection of books and authors more efficiently. They would like to create a Python program to help with this task. The following requirements need to be implemented:
- Create an Author class with the attributes: name, dob (date of birth), and nationality. The class should have a __str__ method to represent the author in a user-friendly format.
- Create a Book class with the following attributes: title, author (an instance of the Author class), publication_date, and price. Also, include a class variable all_books that keeps a list of all book instances created.
The Book class should have:
- A __str__ method to represent the book in a user-friendly format.
- A __eq__ method to compare two books. Two books are considered the same if they have the same title and author.
- A __lt__ method to compare two books based on their publication dates.
- A class method get_all_books that returns a list of all books in the library.
- A static method most_expensive_book that takes a list of book instances and returns the most expensive one.
- Finally, create a Library class that manages books and authors. This class should include the following:
- An attribute collection to store the books in the library.
- A method add_book to add a new book to the library. If the book already exists in the library (based on the __eq__ method from the Book class), it should not be added again.
- A method search_books_by_author to find all books written by a particular author.
- A method most_expensive_book that returns the most expensive book in the library's collection.
- Make sure to handle potential exceptions gracefully and provide informative error messages to the user.
- Your program should demonstrate the creation of multiple Author and Book objects, add these books to a Library object, and show usage of the search_books_by_author and most_expensive_book methods. Use the code provided in the first screenshot below to test your implementation. This code should go at the end of your program after you have satisfied all of the requirements above.

Transcribed Image Text:44 # Test the code
45 tolkien = Author("J.R.R.
46 tolkien.write_book ("The Hobbit", 15)
47 tolkien.write_book("The
48
Tolkien", "British")
Lord of the Rings", 30)
49 rowling = Author("J.K. Rowling", "British")
50 rowling.write_book("Harry Potter and the Philosopher's Stone", 10)
51 rowling.write_book("Harry Potter and the Chamber of Secrets", 10)
52 rowling.write_book("Harry Potter and the Prisoner of Azkaban", 10)
53
54 prolific_author = Author.most_books ()
55 print (f"The author with the most books is {prolific_author.name}
56
f"who wrote {len (prolific_author.books)} books.")
"I
57
58 expensive_book = Book.most expensive_book()
59 print (f"The most expensive book is '{expensive_book.title}' written by
60
f"{expensive_book.author.name}, priced at ${expensive_book.price}.")
II
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
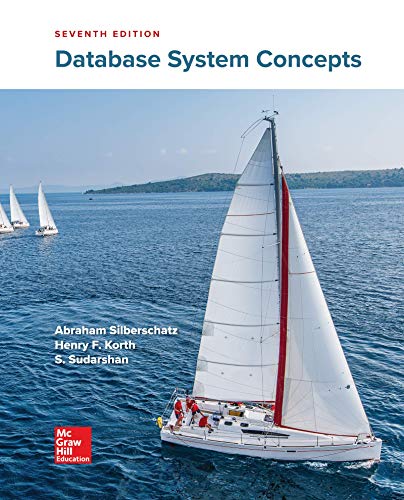
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
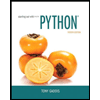
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
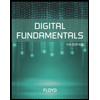
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
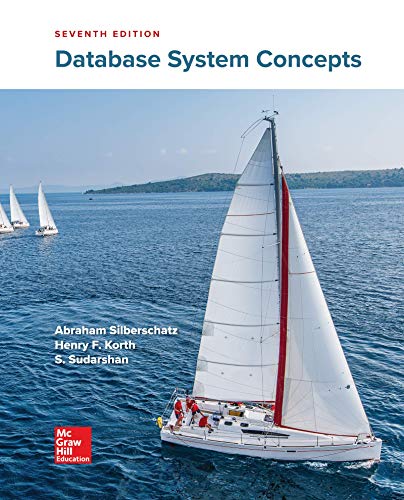
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
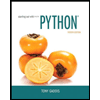
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
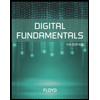
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
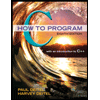
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
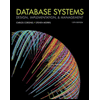
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
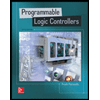
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education