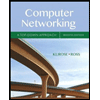
A function takes three (3) strictly positive integer values (less than or equal to 10) corresponding to the sides of a triangle (a, b and c) and tests whether the triangle has two sides which are equal in which case the function outputs a message saying True otherwise False.
Which of the following are correct regarding equivalence partitioning?
b==c, a!=c and a, b and c belong to [1,10] always resulting in True
a==b, a!=c, a, b and c belong to [0,10] resulting in True
b==c, a!=b and a, b and c belong to [1,5] result in True
a>b, b>c and a, b and c belong to [1,10] resulting in True
a==b and a and b belong to [1,10] might be true or false depending on the value of c
a==b, a!=c, is a valid partition always resulting in True

Step by stepSolved in 2 steps

- Write a function to determine whether a string is a permutation of a palindrome given a string. A word or phrase that reads the same both forward and backward is known as a palindrome. Letters are rearranged in a permutation. The palindrome need not contain only words from dictionaries. EXAMPLEInput: Tact CoaOutput: True (permutations: "taco cat'; "atco eta·; etc.)arrow_forwardIn Kotlin, write a higher-order function with an expression body that takes an int n and a function f from int to int and returns the result of calling f(f(n)). For example if you call the function with n = -5 anda function that calculates absolute value, your function will return 5, and if you send the value 2 and a function that calculates the sqaure of an int, the function will return 16. in addition to the function, write the syntax to call it with:a. a function name as the function arguementb. some lambda expression as the function argumentarrow_forwardThe MATLAB function arg supports variable arguments, returns the sum of the number of arguments and all arguments, and has a help function. Write the arg so that the next execution results. >> help argIt supports variable arguments. >> [n,m] = arg(5,6)n = 2m = 11>> [n, m] = arg(3,4,5)n = 3m = 12arrow_forward
- Question 29 Please choose the correct answer.arrow_forwardin c programing Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 The program must define and call the following two functions. Define a function named IntToReverseBinary that takes an int named integerValue as the first parameter and assigns the second parameter, binaryValue, with a string of 1's and 0's representing the integerValue in binary (in reverse order). Define a function named StringReverse that takes an input string as the first parameter and assigns the second parameter, reversedString, with the reverse of inputString. void IntToReverseBinary(int integerValue, char binaryValue[])void StringReverse(char…arrow_forwardWe can assign a value to each character in a word, based on their position in the alphabet (a = 1, b = 2, ..., z = 26). A balanced word is one where the sum of values on the left-hand side of the word equals the sum of values on the right-hand side. For odd length words, the middle character (balance point) is ignored. Write a function that returns true if the word is balanced, and false if it's not. Examples balanced ("zips") → true // "zips" = "zi|ps" = 26+9|16+19 = 35|35 = true balanced ("brake") // "brake" = "br|ke" false = 2+18 | 11+5 = 20 16 = falsearrow_forward
- With the following function f (a, b, c, d), apply Hindley-Milner type checking and identify the type for each argument. f(a, b, c, d) = if b = 1 then else f(a,b,c,d) [] apply if a[1](0) then c(1) else c(d) if a[b](0) then c(b) else f(a, b - 1, c, d) def if apply 1 if apply [] apply if apply applyarrow_forward: Given a string, write a function to check if it is a permutation ofa palindrome. A palindrome is a word or phrase that is the same forwards and backwards. Apermutation is a rearrangement of letters. The palindrome does not need to be limited to justdictionary words.EXAMPLEInput: Tact CoaOutput: True (permutations: "taco cat'; "atco eta·; etc.)arrow_forwardThe correct representation of the function F(A, 8, C) shown in the figure below is: A F Select one: F = m3+ m4 + m5 None of the answers F=m2 + m5 + m6 F= M3 + M4 + M7 F=m1.m3.m6 F= MO.MT.M2.M5.M6 F= I(2, 5,7) F= E(0, 1,7)arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
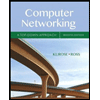
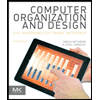
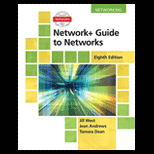
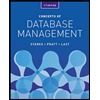
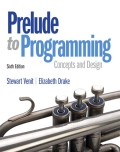
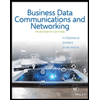