A deque (pronounced “deck”) is a list-based collection that allows additions and removals to take place at both ends. A deque supports the operations addFront(x), removeFront( ), addRear(x), removeRear( ), size( ), and empty( ). Write a class that implements a deque that stores strings using a doubly linked list below. Demonstrate your class with a graphical user interface that allows users to manipulate the deque by typing appropriate commands in a JTextField component, and see the current state of the deque displayed in a JTextArea component. Consult the documentation for the JTextArea class for methods you can use to display each item in the deque on its own line. ########################################################################################################################## import java.util.Objects; public class DoublyLinkedList { Node head; DoublyLinkedList() { head = new Node(null, null, null); } /** Appending the first item in the list **/ public Node addFirst(E item) { Node n = new Node(head.next, head, item); if (head.next != null) head.next.previous = n; head.next = n; return n; } /** Appending the last item in the list **/ public Node add(E item) { Node end = getNode(size() - 1); Node next = new Node(null, end, item); end.next = next; return next; } /**getting node at index given**/ private Node getNode(int index) { if (index > size()) throw new IndexOutOfBoundsException(); Node node = head; for (int i = 0; i <= index; ++i) node = node.next; return node; } /**##########################**/ /** Required clear function: remove all elements from the list. **/ public void clear(){ head = new Node(null, null, null); } /** Required get function: return the element at position index in the list **/ public E get(int index) { Node node = getNode(index); return (node != null) ? node.value : null; } /** Required set function: replace the element at the specified position with the specified element and return the previous element **/ public E set(int index, E element) { Node node = getNode(index); E ret = node.value; node.value = element; return ret; } /**#############**/ /** returns size of the list**/ public int size() { int count = 0; Node node = head.next; while (node != null) { ++count; node = node.next; } return count; } /** checks if list is empty i.e. if size is 0**/ public boolean isEmpty() { return (size() > 0) ? false : true; } /** prints list **/ public String toString() { StringBuilder sb = new StringBuilder("{"); Node next = head.next; while (next != null) { sb.append(next.value + ", "); next = next.next; } String ret = sb.toString(); if (ret.length() > 1) ret = ret.substring(0, ret.length() - 2); return (ret + "}"); } /** Generic Node class for linked list **/ class Node { E value; Node next; Node previous; Node() {} Node(Node next, Node previous, E value) { this.next = next; this.previous = previous; this.value = value; } public String toString() { return "" + value + ""; } } public static void main(String[] args) { DoublyLinkedList list = new DoublyLinkedList(); list.add(1.0); list.add(1.2); list.add(1.4); list.add(2.9); list.addFirst(0.4); list.addFirst(0.1); System.out.println("Printing initial list:"); System.out.println(list); /**testing get function**/ System.out.println("Using get(0) to get element at index 0 => "+list.get(0)); System.out.println("Using get(1) to get element at index 1 => "+list.get(1)); System.out.println("Using get(2) to get element at index 2 => "+list.get(2)); System.out.println("Using get(3) to get element at index 3 => "+list.get(3)); System.out.println("Printing list again:"); System.out.println(list); /**testing set function**/ System.out.println("Using set(0,-7.0) to set element at index 0. The previous element at 0th index => "+list.set(0,-7.0)); System.out.println("Printing modified list again:"); System.out.println(list); System.out.println("Clearing list :"); /**testing clear function**/ list.clear(); System.out.println(list); } }
A deque (pronounced “deck”) is a list-based collection that allows additions and removals to take place at both ends. A deque supports the operations addFront(x), removeFront( ), addRear(x), removeRear( ), size( ), and empty( ). Write a class that implements a deque that stores strings using a doubly linked list below. Demonstrate your class with a graphical user interface that allows users to manipulate the deque by typing appropriate commands in a JTextField component, and see the current state of the deque displayed in a JTextArea component. Consult the documentation for the JTextArea class for methods you can use to display each item in the deque on its own line.
##########################################################################################################################
import java.util.Objects;
public class DoublyLinkedList<E> {
Node head;
DoublyLinkedList() {
head = new Node(null, null, null);
}
/** Appending the first item in the list **/
public Node addFirst(E item) {
Node n = new Node(head.next, head, item);
if (head.next != null)
head.next.previous = n;
head.next = n;
return n;
}
/** Appending the last item in the list **/
public Node add(E item) {
Node end = getNode(size() - 1);
Node next = new Node(null, end, item);
end.next = next;
return next;
}
/**getting node at index given**/
private Node getNode(int index) {
if (index > size())
throw new IndexOutOfBoundsException();
Node node = head;
for (int i = 0; i <= index; ++i)
node = node.next;
return node;
}
/**##########################**/
/** Required clear function: remove all elements from the list. **/
public void clear(){
head = new Node(null, null, null);
}
/** Required get function: return the element at position index in the list **/
public E get(int index) {
Node node = getNode(index);
return (node != null) ? node.value : null;
}
/** Required set function: replace the element at the specified position with the specified element and return the previous element **/
public E set(int index, E element) {
Node node = getNode(index);
E ret = node.value;
node.value = element;
return ret;
}
/**#############**/
/** returns size of the list**/
public int size() {
int count = 0;
Node node = head.next;
while (node != null) {
++count;
node = node.next;
}
return count;
}
/** checks if list is empty i.e. if size is 0**/
public boolean isEmpty() {
return (size() > 0) ? false : true;
}
/** prints list **/
public String toString() {
StringBuilder sb = new StringBuilder("{");
Node next = head.next;
while (next != null) {
sb.append(next.value + ", ");
next = next.next;
}
String ret = sb.toString();
if (ret.length() > 1)
ret = ret.substring(0, ret.length() - 2);
return (ret + "}");
}
/** Generic Node class for linked list **/
class Node {
E value;
Node next;
Node previous;
Node() {}
Node(Node next, Node previous, E value) {
this.next = next;
this.previous = previous;
this.value = value;
}
public String toString() {
return "" + value + "";
}
}
public static void main(String[] args) {
DoublyLinkedList<Double> list = new DoublyLinkedList<Double>();
list.add(1.0);
list.add(1.2);
list.add(1.4);
list.add(2.9);
list.addFirst(0.4);
list.addFirst(0.1);
System.out.println("Printing initial list:");
System.out.println(list);
/**testing get function**/
System.out.println("Using get(0) to get element at index 0 => "+list.get(0));
System.out.println("Using get(1) to get element at index 1 => "+list.get(1));
System.out.println("Using get(2) to get element at index 2 => "+list.get(2));
System.out.println("Using get(3) to get element at index 3 => "+list.get(3));
System.out.println("Printing list again:");
System.out.println(list);
/**testing set function**/
System.out.println("Using set(0,-7.0) to set element at index 0. The previous element at 0th index => "+list.set(0,-7.0));
System.out.println("Printing modified list again:");
System.out.println(list);
System.out.println("Clearing list :");
/**testing clear function**/
list.clear();
System.out.println(list);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

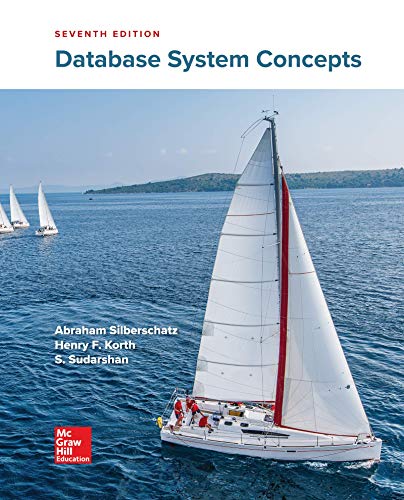
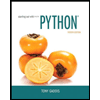
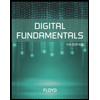
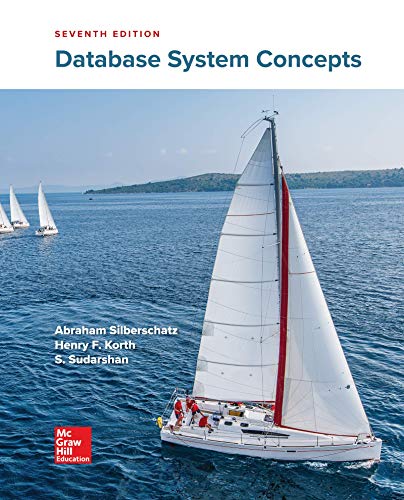
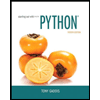
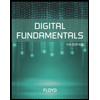
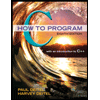
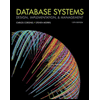
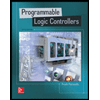