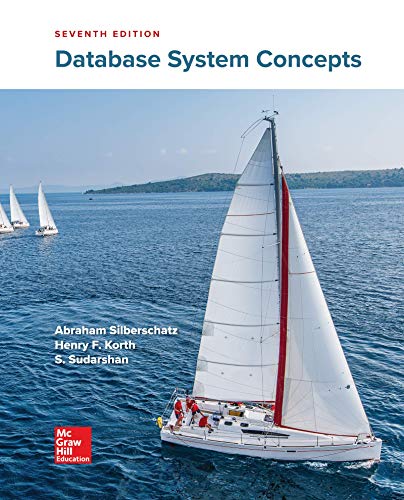
Concept explainers
A binary search tree is a data structure that consists of JavaScript objects called "nodes". A tree always has a root node which holds its own integer value property and can have up to two child nodes (or leaf nodes), a left and right property. A leaf node holds a value attribute and, likewise, a left and right attribute each potentially pointing to another node in the binary tree. Think of it as a javascript object with potentially more sub-objects referenced by the left and right attributes. There are certain rules that apply to a binary tree: A node's left leaf node has a value that is <= than to its own value A node's right leaf node has a value that is => its own value. In other words: let node = { value: left: right: = this object's value> } You will be writing a function called isPresent that takes two arguments: the root object and a value (number), and returns a boolean true if the value is present in the tree or false if it is not present.
function isPresent(root, value) {
// your code here
// return boolean
}
Example test case:
Inputs:
root => { "value": 5, "left": { "value": 3, "left": { "value": 2, "left": null, "right": null }, "right": { "value": 4, "left": null, "right": null } }, "right": { "value": 7, "left": { "value": 6, "left": null, "right": null }, "right": { "value": 8, "left": null, "right": null } } }
value => 6
Output: true
Reasoning: 6 is the value of a leaf node in the binary tree ..
"left": { "value": 6, "left": null, "right": null }, ..

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- unique please Your task for this assignment is to identify a spanning tree in one connected undirected weighted graph using C++. Implement a spanning tree algorithm using C++. A spanning tree is a subset of the edges of a connected undirected weighted graph that connects all the vertices together, without any cycles. The program is interactive. Graph edges with respective weights (i.e., v1 v2 w) are entered at the command line and results are displayed on the console. Each input transaction represents an undirected edge of a connected weighted graph. The edge consists of two unequal non-negative integers in the range 0 to 9 representing graph vertices that the edge connects. Each edge has an assigned weight. The edge weight is a positive integer in the range 1 to 99. The three integers on each input transaction are separated by space. An input transaction containing the string “end-of-file” signals the end of the graph edge input. After the edge information is read, the process…arrow_forwardJavaarrow_forwardJava code that eliminates the binary search's node with the lowest value. returns a reference to its element from a tree. If this tree is empty, it raises an EmptyCollectionException. If the tree is empty, returning a reference to the node with the fewest values produces an EmptyCollectionException.arrow_forward
- 1. Draw the recursion tree generated when calling hanoi (3, 1, 3). The first parameter is numDisks, the second is the number of the fromPeg, and the last is the toPeg. Each node in the tree should include the function name and three parameters described above. hanoi (3, 1, 3) is the root node in the drawing.arrow_forwardDATA STRUCTURES AND ALGORITHMSarrow_forwardWrite a Java program to Construct a 2-3 tree with at last 10 key values, Search the particular key value present in the 2-3 tree or not and finally Delete any one leaf node which has single key value.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
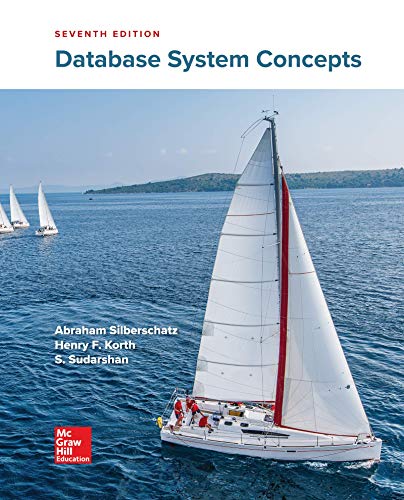
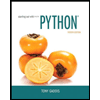
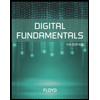
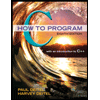
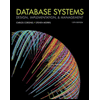
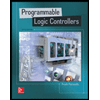