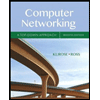
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
self.tail = new_node
else:
new_node.next = current_node.next
current_node.next = new_node
def remove_after(self, current_node):
if (current_node == None) and (self.head != None):
succeeding_node = self.head.next
self.head = succeeding_node
if succeeding_node == None: # Remove last item
self.tail = None
elif current_node.next != None:
succeeding_node = current_node.next.next
current_node.next = succeeding_node
if succeeding_node == None: # Remove tail
self.tail = current_node
def display(self):
node = self.head
if (node == None):
print('Empty!')
while node != None:
print(node.data, end=' ')
node = node.next
def remove_smallest_node(self):
#*** Your code goes here!***
# NOTE: this function will print out the information about the smallest node
# before removing it
def Generate_List_of_LinkedLists(n):
LLL = []
#*** Your code goes here!***
# NOTE: this function will read the input from the user for each of the lists and
# generate the list
return LLL
if __name__ == '__main__':
#*** Your code goes here!***
![19.19 LAB: Linear Search
In this lab you are asked to complete the provided code so that it generates a list of singly-linked-lists (note: this will be a python list, where
each element of the list is a singly linked list). Your code should do the following:
To generate a list of singly linked lists, your code should accept the number of linked lists as an input
• Then, for each linked list, it should accept integers as nodes till the user enters -1
It should next display all the linked lists in the lists entered by the user
Next, it should accept a number - this is an index position for the list (a linked list)
• For the linked list in the index position supplied by the user, your code should find the node with the smallest value and delete it
o Your code should display "Empty!", if there is no nodes left in the linked list after removing the smallest node!
Finally, it should print the modified linked list
For example, when the input is:
3
7
-1
3
4
7
9.
-1
1
The output should be:
linked list [ 0 ]:
1 3 5 7
linked list [ 1 ]:
3 4 7 2 9
the smallest node is:
2
List 1 after removing the smallest node:
3 4 79
Note Make sure to check for the following possible user's mistakes
• if the user inputs a negative number as the number of linked lists in the list, your code should display: "Wrong input! try again:"; then,
let the user to input another number
Same for the Empty linked lists](https://content.bartleby.com/qna-images/question/fe5af5d5-f811-48fd-8e6e-3830a7fa1b94/061e07ba-cc1b-485e-a937-1b59096f121e/lys5ysg_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- You may have found it somewhat tedious and unpleasant to use the debugger and visualizer to verify the correctness of your addFirst and addLast methods. There is also the problem that such manual verification becomes stale as soon as you change your code. Imagine that you made some minor but uncertain change to addLast]. To verify that you didn't break anything you'd have to go back and do that whole process again. Yuck. What we really want are some automated tests. But unfortunately there's no easy way to verify correctness of addFirst and addLast] if those are the only two methods we've implemented. That is, there's currently no way to iterate over our list and get bad its values and see that they are correct. That's where the toList method comes in. When called, this method returns a List representation of the Deque. For example, if the Deque has had addLast (5) addLast (9) addLast (10), then addFirst (3) called on it, then the result of toList() should be a List with 3 at the…arrow_forwardfrom PlaneQueue import PlaneQueue from PlaneNode import PlaneNode if __name__ == "__main__": plane_queue = PlaneQueue() # TODO: Read in arriving flight codes and whether a flight has landed. # Print the queue after every push() or pop() operation. If the user # entered "landed", print which flight has landed. Continue until -1 # is read.arrow_forwardPython please. Starter code: from player import Playerdef does_player_1_win(p1_hand, p2_hand):#given both player-hands, does player 1 win? -- return True or Falsedef main():# Create players# Have the players play repeatedly until one wins enough timesmain() #you might need something hereclass Player: # player for Rock, Paper, Scissors# ADD constructor, init PRIVATE attributes# ADD method to get randomly-generated "hand" for player# ADD method to increment number of wins for player# ADD method to reset wins to 0# ADD getters & setters for attributesarrow_forward
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardPython code: ##############################class Node: def __init__(self,value): self.left = None self.right = None self.val = value ###############################class BinarySearchTree: def __init__(self): self.root = None def print_tree(node): if node == None: return print_tree(node.left) print_tree(node.right) print(node.val) ################################################## Task 1: get_nodes_in_range function################################################# def get_nodes_in_range(node,min,max): # # your code goes here # pass # fix this if __name__ == '__main__': BST = BinarySearchTree() BST.root = Node(10) BST.root.left = Node(5) BST.root.right = Node(15) BST.root.left.left = Node(2) BST.root.left.right = Node(8) BST.root.right.left = Node(12) BST.root.right.right = Node(20) BST.root.right.right.right = Node(25) print(get_nodes_in_range(BST.root, 6, 20))arrow_forwardLLNode: public class LLNode<T> { protected T info; protectedLLNode<T>link; public LLNode(Tinfo){ this.info=info; link=null; } publicvoidsetInfo(Tinfo){ this.info=info; } publicTgetInfo(){ return info; } publicvoidsetLink(LLNode<T>link){ this.link=link; } publicLLNode<T>getLink(){ return link; } @Override public String toString(){ return "LLNode [info="+info+", link="+link+"]"; } } MyLLNode: public class MyLLNode { LLNode<String>head; public MyLLNode(){ this.head=null; } public MyLLNode(String first){ this.head=newLLNode<String>(first); } //------------------------------------------------------------- public void addAtEnd(String newstr){ LLNode<String>node=head; LLNode<String>newNode=newLLNode<String>(newstr); if(this.head==null){ this.head=newNode; } else{ while(node.getLink()!=null){ node=node.getLink(); } node.setLink(newNode); } } //------------------------------------------------------------- public void addAtFront(String…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
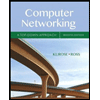
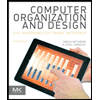
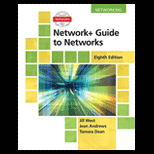
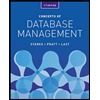
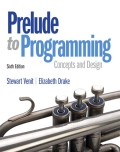
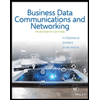