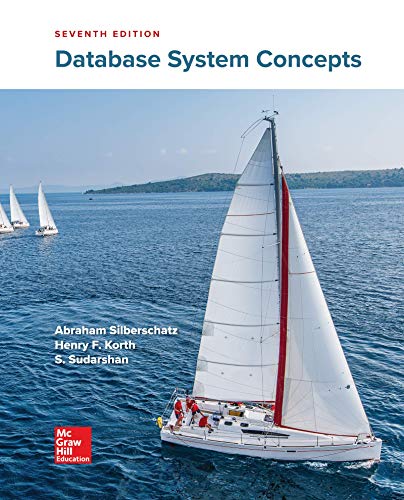
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![9.7
(The Account class) Design a class named Account that contains:
A private int data field named id for the account (default 0).
I A private double data field named balance for the account (default 0).
A private double data field named annua]InterestRate that stores the
current interest rate (default 0). Assume all accounts have the same interest
rate.
A private Date data field named dateCreated that stores the date when the
account was created.
A no-arg constructor that creates a default account.
A constructor that creates an account with the specified id and initial balance.
1 Theaccessorandmutatormethodsfor id,balance, andannualInterestRate.
The accessor method for dateCreated.
I A method named getMonthlyInterestRate() that returns the monthly
interest rate.
A method named getMonthlyInterest() that returns the monthly interest.
A method named withdraw that withdraws a specified amount from the
account.
I A method named deposit that deposits a specified amount to the account.
Draw the UML diagram for the class and then implement the class. (Hint: The
method getMonthlyInterest() is to return monthly interest, not the interest rate.
Monthly interest is balance * monthlyInterestRate. monthlyInterestRate
is annualInterestRate / 12. Note that annualInterestRate is a percentage,
e.g., like 4.5%. You need to divide it by 100.)
Write a test program that creates an Account object with an account ID of 1122,
a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw
method to withdraw $2,500, use the deposit method to deposit $3,000, and print
the balance, the monthly interest, and the date when this account was created.
VideoNote
The Fan class](https://content.bartleby.com/qna-images/question/8dbcbf0b-9123-4a1c-9796-89fa34227664/ece99dfe-8295-47fb-b30f-2d2ffcd96215/ofrdygn_thumbnail.jpeg)
Transcribed Image Text:9.7
(The Account class) Design a class named Account that contains:
A private int data field named id for the account (default 0).
I A private double data field named balance for the account (default 0).
A private double data field named annua]InterestRate that stores the
current interest rate (default 0). Assume all accounts have the same interest
rate.
A private Date data field named dateCreated that stores the date when the
account was created.
A no-arg constructor that creates a default account.
A constructor that creates an account with the specified id and initial balance.
1 Theaccessorandmutatormethodsfor id,balance, andannualInterestRate.
The accessor method for dateCreated.
I A method named getMonthlyInterestRate() that returns the monthly
interest rate.
A method named getMonthlyInterest() that returns the monthly interest.
A method named withdraw that withdraws a specified amount from the
account.
I A method named deposit that deposits a specified amount to the account.
Draw the UML diagram for the class and then implement the class. (Hint: The
method getMonthlyInterest() is to return monthly interest, not the interest rate.
Monthly interest is balance * monthlyInterestRate. monthlyInterestRate
is annualInterestRate / 12. Note that annualInterestRate is a percentage,
e.g., like 4.5%. You need to divide it by 100.)
Write a test program that creates an Account object with an account ID of 1122,
a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw
method to withdraw $2,500, use the deposit method to deposit $3,000, and print
the balance, the monthly interest, and the date when this account was created.
VideoNote
The Fan class
Expert Solution

arrow_forward
Step 1
import java.util.Date; | |
public class Account { | |
// Data fields | |
private int id; | |
private double balance; | |
private static double annualInterestRate; | |
private Date dateCreated; | |
// Constructors | |
/** Creates a default account */ | |
Account() { | |
this(0, 0); | |
} | |
/** Creates an account with the specified id and initial balance */ | |
Account(int id, double balance) { | |
this.id = id; | |
this.balance = balance; | |
annualInterestRate = 0; | |
dateCreated = new Date(); | |
} | |
// Mutator methods | |
/** Set id */ | |
public void setId(int id) { | |
this.id = id; | |
} | |
/** Set balance */ | |
public void setBalance(double balance) { | |
this.balance = balance; | |
} | |
/** Set annualInterestRate */ | |
public void setAnnualInterestRate(double annualInterestRate) { | |
this.annualInterestRate = annualInterestRate; | |
} | |
// Accessor methods | |
/** Return id */ | |
public int getId() { | |
return id; | |
} | |
/** Return balance */ | |
public double getBalance() { | |
return balance; | |
} | |
/** Return annualInterestRate */ | |
public double getAnnualInterestRate() { | |
return annualInterestRate; | |
} | |
/** Return dateCreated */ | |
public String getDateCreated() { | |
return dateCreated.toString(); | |
} | |
/** Return monthly interest rate */ | |
public double getMonthlyInterestRate() { | |
return annualInterestRate / 12; | |
} | |
// Methods | |
/** Return monthly interest */ | |
public double getMonthlyInterest() { | |
return balance * (getMonthlyInterestRate() / 100); | |
} | |
/** Decrease balance by amount */ | |
public void withdraw(double amount) { | |
balance -= amount; | |
} | |
/** Increase balance by amount */ | |
public void deposit(double amount) { | |
balance += amount; | |
} | |
/** Return a String decription of Account class */ | |
public String toString() { | |
return "\nAccount ID: " + id + "\nDate created: " + getDateCreated() | |
+ "\nBalance: $" + String.format("%.2f", balance) + | |
"\nMonthly interest: $" + String.format("%.2f", getMonthlyInterest()); | |
} | |
} |
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- May I please have your assistance with this lab: 2.15 LAB: Artwork label ( modules) Part of the code is correct. I am only needing the code to be correct for the following errors: This is the current code & errors at bottom of code: # Artist.py class Artist: # below is the comstructor to initializez artist infomration # constructor initialize the artist's name to "None" and the years of birth and death to 0 def __init__(self, name='None', birth_year=0, death_year=0): self.name = name self.birth_year = birth_year self.death_year = death_year def print_info(self): if self.birth_year >= 0 and self.death_year >= 0: print(f'Artist: {self.name} ({self.birth_year} to {self.death_year})') elif self.birth_year >= 0: print(f'Artist: {self.name} ({self.birth_year} - present)') else: print(f'Artist: {self.name} (unkown)') # Artwork.py class Artwork:…arrow_forwardQ5) Write, compiles, and test a class that displays the following pattern on the screen: X X X XXXXX X X X X X X X XXXX Save the class as Q5 X XXXX X X X X X X Xarrow_forward13arrow_forward
- 25. The size in Bytes of an empty class is 04 01 8arrow_forward5. Multiple Choice Questions: • A non-default destructor is needed_ 1. always (for correct operation) optionally 2. 3. only if a developer wants to be more explicit than the default 4. when there will be more than one instance of a class 5. when class data members are dynamically allocated • Consider the following code: int x = 15; // located in memory at 0x1236 int* intPtr; // located in memory at 0x1240 intPtr = &x; cout << *intPtr; What is its output? 1. an error 2. 15 3. a random number 4. 0x1236 5. 0x1240 • The following function prototype is for the UberObject & operator-(UberObject & rhs); 1. copy assignment 2. default constructor 3. move constructor 4. move assignment 5. copy constructorarrow_forwardT/F 3) If class AParentClass has a public instance data x, and AChildClass is a derived class of AParentClass, thenAChildClass can access x but can not redefine x to be a different type.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
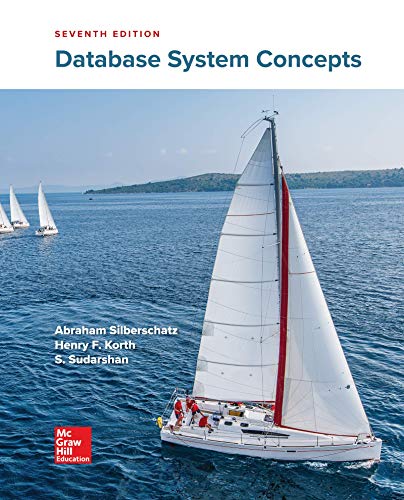
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
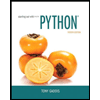
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
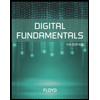
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
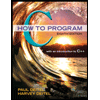
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
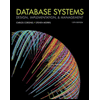
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
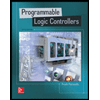
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education