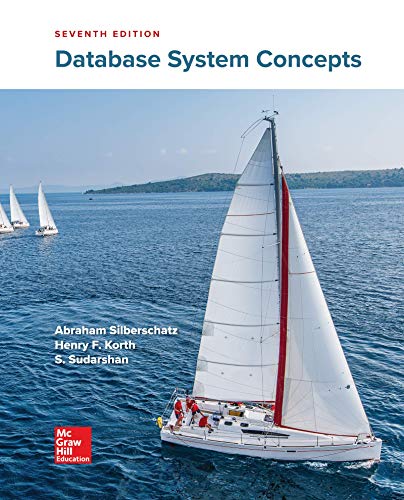
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
4.7.1: Writing a recursive math function.
Write code to complete raise_to_power(). Note: This example is for practicing recursion; a non-recursive function, or using the built-in function math.pow(), would be more common.
Sample output with inputs: 4 24^2 = 16
Sample output with inputs: 4 24^2 = 16
def raise_to_power(base_val, exponent_val):
if exponent_val == 0:
result_val = 1
else:
result_val = base_val * ''' Your solution goes here '''
return result_val
user_base = int(input())
user_exponent = int(input())
print('%d^%d = %d' % (user_base, user_exponent,
raise_to_power(user_base, user_exponent)))
need solution to result_val =base_val* ???????
![entry level cyber
* Home
Gradebook
zy Section 4.7 - CYB X
A python - LAB: Sor X
b Answered: 4.7.1:
* [Solved] How do
G 5.16 LAB: Cryptog X
+
i learn.zybooks.com/zybook/CYB_135_54915392/chapter/4/section/7
= zyBooks My library > CYB/135: Object-Oriented Security Scripting home > 4.7: Recursive math functions
E zyBooks catalog
? Help/FAQ
Kenneth Schultz -
• More on the GCD algorithm from wikipedia.org
CHALLENGE
АCTIVITY
4.7.1: Writing a recursive math function.
Write code to complete raise_to_power(). Note: This example is for practicing recursion; a non-recursive function, or using the built-
in function math.pow(), would be more common.
Sample output with inputs: 4 2
4^2 = 16
346682.2019644.qx3zgy7
1 def raise_to_power (base_val, exponent_val):
if exponent_val == 0:
result_val = 1
else:
result_val = base_val *
1 test
passed
2
4
5
Your solution goes here''
All tests
6
passed
7
return result_val
8
9 user_base = int(input())
10 user_exponent = int(input())
11
12 print('%d^%d = %d' % (user_base, user_exponent,
raise_to_power(user_base, user_exponent)))
13
Run
View your last submission v
3:40 AM
O Type here to search
77°F Cloudy
9/18/2021](https://content.bartleby.com/qna-images/question/7ef9d07b-5934-4723-aca6-faf08a622511/a8b7c86f-bd9a-4cfa-a471-f72340fb898f/jc6n5p_thumbnail.png)
Transcribed Image Text:entry level cyber
* Home
Gradebook
zy Section 4.7 - CYB X
A python - LAB: Sor X
b Answered: 4.7.1:
* [Solved] How do
G 5.16 LAB: Cryptog X
+
i learn.zybooks.com/zybook/CYB_135_54915392/chapter/4/section/7
= zyBooks My library > CYB/135: Object-Oriented Security Scripting home > 4.7: Recursive math functions
E zyBooks catalog
? Help/FAQ
Kenneth Schultz -
• More on the GCD algorithm from wikipedia.org
CHALLENGE
АCTIVITY
4.7.1: Writing a recursive math function.
Write code to complete raise_to_power(). Note: This example is for practicing recursion; a non-recursive function, or using the built-
in function math.pow(), would be more common.
Sample output with inputs: 4 2
4^2 = 16
346682.2019644.qx3zgy7
1 def raise_to_power (base_val, exponent_val):
if exponent_val == 0:
result_val = 1
else:
result_val = base_val *
1 test
passed
2
4
5
Your solution goes here''
All tests
6
passed
7
return result_val
8
9 user_base = int(input())
10 user_exponent = int(input())
11
12 print('%d^%d = %d' % (user_base, user_exponent,
raise_to_power(user_base, user_exponent)))
13
Run
View your last submission v
3:40 AM
O Type here to search
77°F Cloudy
9/18/2021
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- A1.1.6 (a) create the recursive function BinaryGCd (a,b) based on the following pseudo code # recursive pseudo-code def BinaryGCF (a,b): arrange order so ab if a=0: if a and b are even: else if a is even: else if b is even: else: done, b is GCF GCF (a,b) = 2*GCF (a/2, b/2) GCF (a,b) = GCF (a/2, b) GCF (a,b) GCF (a,b) In [ ]: def BinaryGCF (a,b): GCF (a,b/2) GCF (a,b-a) (b) demonstrate that it gives the same results as pfGCD (a,b) and EuclidGCD (a, b) from A1.5. "enter the recursive code below" for a,b in [(660,350), (99,53), (30,84)]: # demonstrate all GCD functions give the same result 13arrow_forward11.10 LAB: All permutations of names Write a program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia #include #include #include main.cpp: using namespace std; // TODO: Write method to create and output all permutations of the list of names. void AllPermutations(const vector &permList, const vector &nameList) { } int main(int argc, char* argv[]) { vector nameList; vector permList; string name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. return 0; }arrow_forward6.9 LAB: All permutations of names Write a program that lists all ways people can line up for a photo (all permutations of a list of Strings). The program will read a list of one word names (until -1), and use a recursive method to create and output all possible orderings of those names, one ordering per line. When the input is: Julia Lucas Mia -1 then the output is (must match the below ordering): Julia Lucas Mia Julia Mia Lucas Lucas Julia Mia Lucas Mia Julia Mia Julia Lucas Mia Lucas Julia import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names.public static void allPermutations(ArrayList<String> permList, ArrayList<String> nameList) {} public static void main(String[] args) {Scanner scnr = new Scanner(System.in);ArrayList<String> nameList = new ArrayList<String>();ArrayList<String> permList = new ArrayList<String>();String name;//…arrow_forward
- 4.6.1: Recursive function: Writing the base case. Add an if branch to complete double_pennies()'s base case.Sample output with inputs: 1 10Number of pennies after 10 days: 1024 Note: If the submitted code has an infinite loop, the system will stop running the code after a few seconds, and report "Program end never reached." The system doesn't print the test case that caused the reported message # Returns number of pennies if pennies are doubled num_days timesdef double_pennies(num_pennies, num_days):total_pennies = 0 ''' Your solution goes here ''' else:total_pennies = double_pennies((num_pennies * 2), (num_days - 1)) return total_pennies # Program computes pennies if you have 1 penny today,# 2 pennies after one day, 4 after two days, and so onstarting_pennies = int(input())user_days = int(input()) print('Number of pennies after', user_days, 'days: ', end="")print(double_pennies(starting_pennies, user_days))arrow_forwardTake Test: Final Exam Theory 202 A Question Completion Status: 1. 2 4. 6. 9. 10 11 12 13 21 QUESTION 17 Determine the output generated by the partial C code given. #include #include void main() { int a=9, b=4,c=0; c= pow(sqrt(b)+sqrt(a),2); printf("%d, %d and %d", a, b, c ); For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac).arrow_forwardQ1.1. Write a NumPy program to get the following array by using loops Output: array([[ 1, 2, 3, 4, 5, 6, 7, 8, 9], [2, 4, 6, 8, 10, 12, 14, 16, 18], [3, 6, 9, 12, 15, 18, 21, 24, 27], [4, 8, 12, 16, 20, 24, 28, 32, 36], [5, 10, 15, 20, 25, 30, 35, 40, 45], [6, 12, 18, 24, 30, 36, 42, 48, 54], [7, 14, 21, 28, 35, 42, 49, 56, 63], [8, 16, 24, 32, 40, 48, 56, 64, 72], [9, 18, 27, 36, 45, 54, 63, 72, 81]]) Q1.2. Replace all odd numbers in array with -1. Q1.3. By using numpy functions and prog array, get the result. prog = np.array([1,2,3,4,6]). Result= array([1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 3, 3, 3, 4, 4, 4, 4, 4, 4, 4, 4, 6, 6, 6, 6, 6, 6, 6, 6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6, 1, 2, 3, 4, 6]) Q1.4. Write a NumPy program to create random 2 array and size of arrays should be 1x15. In your array there should be integer numbers from 0 to 9 and by using numpy function, find common numbers in your array. Q1.5. Write a NumPy program to generate 50…arrow_forward
- python This is what i have:arrow_forwardCHALLENGE ACTIVITY 11.1.1: Calling a recursive function. Write a statement that calls the recursive function backwards_alphabet() with input starting_letter.Sample output with input: 'f' f e d c b a USE PYTHON FOR THIS!arrow_forwardR7.30Assuming the following declarations, find the mistakes in the statements below. struct A { int m1[2]; int m2; } struct B { A a1; A* a2; int m3; } A a; B b[2]; a.b[0].a2 = a; b.b[1].m2 = 42; c.b[2].a2 = new A; d.b[0].a1.m1 = 42; e.b[1].a2->m2 = 42;arrow_forward
- 5.6 Matarrow_forward2.4: Floating-point ACTIVITY 2.4.2. Unient types. I calify point. zyBooks catalog 556802.4192934.qx3zqy7 Jump to level 1 The reciprocal of velocity_input is 1 / velocity_input. The following program intends to read a floating-point value from input, compute the reciprocal of the value, and output the reciprocal, but the code contains an error. Find and fix the error. Ex: If the input is 0.480, then the output is: The reciprocal of velocity = 10.480 = 2.083 1 # Modify the following code 2 velocity_input = input() 3 velocity_recip = 1 / velocity_input 4 5 print(f'The reciprocal of velocity = 1 / {velocity_input: .3f} = {velocity_recip: .3f}') Iarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
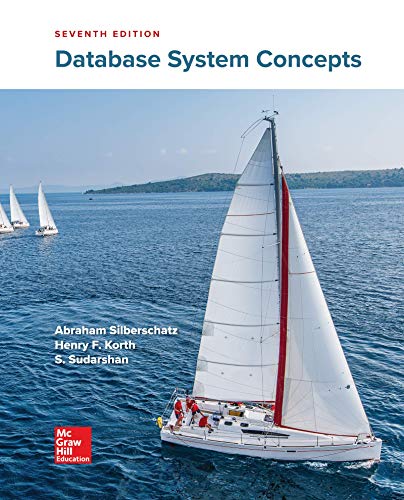
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
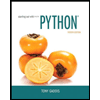
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
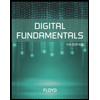
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
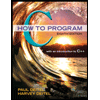
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
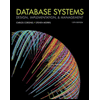
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
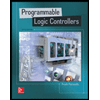
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education