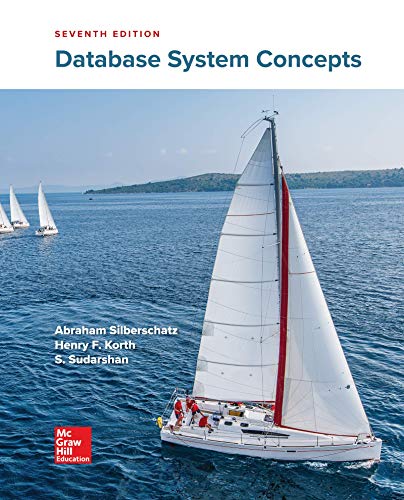
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
The output is actually missing one hi -2 at the end before mark - 1
![**Section 5.20 - CIS 161: Introduction to C Programming - LAB: Word Frequencies**
### Objective:
The aim of this lab is to write a C program that reads a series of words and outputs the frequency of each word.
### Instructions:
- Develop your program and test it as much as you need.
- Submit the final version before the deadline.
### Code Details:
A skeleton code is provided with the following functionality:
- Read input string.
- Count and store frequencies of each word.
- Print the words and their respective frequencies.
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 50
#define MAX_WORD_LENGTH 50
int main() {
char words[MAX_WORDS][MAX_WORD_LENGTH];
int frequencies[MAX_WORDS];
int numWords = 0;
char input[200];
fgets(input, 200, stdin);
// Split the input string into words
char *token = strtok(input, " \n");
while (token != NULL) {
int found = 0;
for (int i = 0; i < numWords; i++) {
if (strcmp(words[i], token) == 0) {
frequencies[i]++;
found = 1;
break;
}
}
if (!found) {
strcpy(words[numWords], token);
frequencies[numWords] = 1;
numWords++;
}
token = strtok(NULL, " \n");
}
// Output the words and frequencies
for (int i = 0; i < numWords; i++) {
if (frequencies[i] != 0) {
printf("%s - %d\n", words[i], frequencies[i]);
}
}
return 0;
}
```
### User Interface:
- **Develop mode:** Allows you to write and test your code.
- **Submit mode:** Submit the final version of your code for grading.
### Input and Output:
1. **Enter Program Input (optional):**
- Example: `5 hey hi Mark hi mark`
2. **Run Program:**
- Click to execute the code and observe the program's output.
3. **Program Output Displayed Here:**
- The area where the result of running the program will be displayed.
### Example:
#### Input:
```
5 hey hi Mark hi mark
```](https://content.bartleby.com/qna-images/question/3e035166-c990-4dd0-a7c5-b2c139b07c7d/5f282657-c447-41a2-b9e6-88b34d64eb2f/sxq0gol_thumbnail.png)
Transcribed Image Text:**Section 5.20 - CIS 161: Introduction to C Programming - LAB: Word Frequencies**
### Objective:
The aim of this lab is to write a C program that reads a series of words and outputs the frequency of each word.
### Instructions:
- Develop your program and test it as much as you need.
- Submit the final version before the deadline.
### Code Details:
A skeleton code is provided with the following functionality:
- Read input string.
- Count and store frequencies of each word.
- Print the words and their respective frequencies.
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 50
#define MAX_WORD_LENGTH 50
int main() {
char words[MAX_WORDS][MAX_WORD_LENGTH];
int frequencies[MAX_WORDS];
int numWords = 0;
char input[200];
fgets(input, 200, stdin);
// Split the input string into words
char *token = strtok(input, " \n");
while (token != NULL) {
int found = 0;
for (int i = 0; i < numWords; i++) {
if (strcmp(words[i], token) == 0) {
frequencies[i]++;
found = 1;
break;
}
}
if (!found) {
strcpy(words[numWords], token);
frequencies[numWords] = 1;
numWords++;
}
token = strtok(NULL, " \n");
}
// Output the words and frequencies
for (int i = 0; i < numWords; i++) {
if (frequencies[i] != 0) {
printf("%s - %d\n", words[i], frequencies[i]);
}
}
return 0;
}
```
### User Interface:
- **Develop mode:** Allows you to write and test your code.
- **Submit mode:** Submit the final version of your code for grading.
### Input and Output:
1. **Enter Program Input (optional):**
- Example: `5 hey hi Mark hi mark`
2. **Run Program:**
- Click to execute the code and observe the program's output.
3. **Program Output Displayed Here:**
- The area where the result of running the program will be displayed.
### Example:
#### Input:
```
5 hey hi Mark hi mark
```
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C₂ S = 0 A B₂ b. B = 0000 C. ( FA S₁₂ = 1111 S=0 A = 1001 B = 0111 d. S=1 A = 0000 B 1100 e... S=1 A = 0011 B = 1010 Az Input values for the adder-subtractor circuit above are given below. In each case, indicate the values of the outputs C4, S3, S₂, S₁, and So- a. ( S = 1 A = 0000 B = 1111 9₂ C4 S3 = S₂ = S₁ = So = C4= S₂ = S₂ = S₁ = So= C4= S3 S₂ = S₁ = So= B₂ C4 = S3 = S₂ = S₁ = So= C₁ = S3 = S₂ = S₁ = So = A₂ FA B₁ A₁ D FA St Ao FA Soarrow_forwardC++ code for the output belowarrow_forwardi need the answer quicklyarrow_forward
- A digit is to be showed on the 7-segment display. Select all the C flow-control constructs that can be used to determine the suitable bit-pattern? Select one or more: □ a. if-else b. switch-case Oc for d. do-while e. whilearrow_forwardIf the input A = 10101010, input B = 10101010 Can you please help me create/ draw a full subtractor circuit with (input A , B , Bin and output Sum, Bout ) to get the correct outcome please.arrow_forwardHow do you ensure the output is only 2 decimal places to the rightarrow_forward
- How do I generate a 4-bit DIGITAL sine wave in Python and scale the y-axis from 0000 to 0111? The y-axis has to start at 0000 and end at 0111. The amplitude of the sine-wave is 0111. Please do not post if the math in the code is wrong.arrow_forwardplsarrow_forwardConvert the infix expression to postfix: a+b/c+darrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
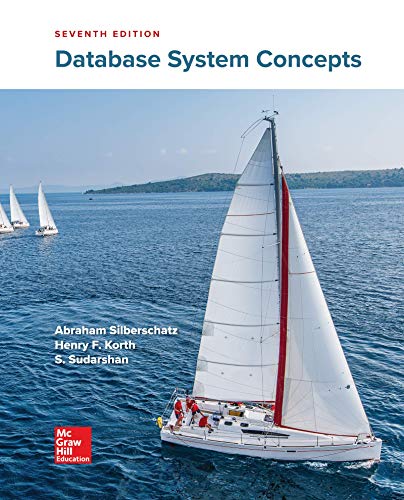
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
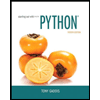
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
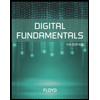
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
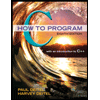
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
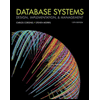
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
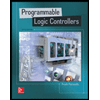
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education