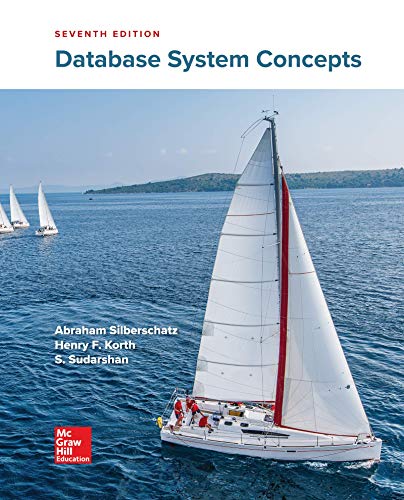
In coral language: Write a function DrivingCost with parameters drivenMiles, milesPerGallon, and dollarsPerGallon, that returns the dollar cost to drive those miles. All items are of type float.
Ex: If the function is called with 50 20.0 3.1599, the function returns 7.89975.
Define that function in a program whose inputs are the car's miles/gallon and the gas dollars/gallon (both floats). Output the gas cost for 10 miles, 50 miles, and 400 miles, by calling your DrivingCost function three times.
Ex: If the input is 20.0 3.1599, the output is:
1.57995 7.89975 63.198
Note: Small expression differences can yield small floating-point output differences due to computer rounding. Ex: (a + b)/3.0 is the same as a/3.0 + b/3.0 but output may differ slightly. Because our system tests programs by comparing output, please obey the following when writing your expression for this problem. In the DrivingCost function, use the variables in the following order to calculate the cost: drivenMiles, milesPerGallon, dollarsPerGallon.
need all subsections and sections answered.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

How do you ensure the output is only 2 decimal places to the right
How do you ensure the output is only 2 decimal places to the right
- Write a Python program that plays the rock-paper-scissors game, that following happens: Rock breaks scissors Paper covers rock Scissors cut paper There is a tie (both players picked the same thing) Fulfillments: Your program should have at least 2 functions, including a main() function (no global variables or global code other than a call to main within an if-statement) You should use at least one multi-way if-statement (using "elif"). You should use at least one logical "and" or "or" in your program You should always output who won (unless there's a tie). Every function (except main) needs to have a comment in a triple-quoted string at the beginning, briefly explaining what it does. Have a repeat function that asks the user if they want to exit the game (ask the user whether he/she wants to continue the game.)arrow_forwardA pedometer treats walking 2,000 steps as walking 1 miles. Define a function name StepsToMiles that takes an integer as a parameter, representing the numbers of steps, and returns a float that represents the number of miles walked. Then, write a main program that reads the number of steps as an input, calls function StepsToMiles() with the inputs as an argument, and outputs the miles walked.arrow_forwardIn the U.S. fuel efficiency of cars is specified in miles per gallon (mpg). In Europe it is often expressed in liters per 100 km. Write a MATLAB user- defined function that converts fuel efficiency from mpg to liters per 100 km. For the function name and arguments, use Lkm=mpgToLpkm (mpg). The input argument mpg is the efficiency in mi/gl, and the output argument Lkm is the efficiency in liters per 100 km (rounded to the nearest hundredth). Use the function in the Command Window to: (a) Determine the fuel efficiency in liters per 100 km of a car whose fuel effi- ciency is 21 mi/gal. (b) Determine the fuel efficiency in liters per 100 km of a car whose fuel effi- ciency is 36 mi/gal.arrow_forward
- write a program in C++, that should demonstrate a value returning function. Ask the user for their name and the type of ice cream they want. 1 for a bowl, 2 for a cone, and 3 for a take home ½ gallon container. Also ask how many toppings they want for their ice cream. Then call getIceCreamTotal() and pass it the type of ice cream and how many toppings they want. Show the total it returns to the user with their name. getIceCreamTotal() should find the total for the ice cream and return it. Bowls are 2 dollars, cones are 1.5 dollars, and 5 dollars to take it home in a big container. The toppings are .75 each. Find the price plus the cost of the toppings and return that total.arrow_forwardWrite a function tuplinator (number) that takes a number argument and returns a tuple consisting of the number, the number squared, and the number cubed. See below for examples. For example: Test Result print(tuplinator (2)) (2, 4, 8) print(tuplinator (3)) (3, 9, 27) Answer: (penalty regime: 0, 10, ... %) 1arrow_forwardGiven the function of def happyBirthday(name, age = 10): print("Happy Birthday", name, "I hear your", age, "today") What is the output with a function call of: happyBirthday(age = 7, "Sarah") Happy Birthday Sarah I hear your 10 today Happy Birthday Sarah I hear your 7 today Happy Birthday 7 I hear your Sarah today Error Message Parameters are __________ Variables defined in a function header. Variables or constants used in a function call. Both variable defined in a function header, and a local variable for the function Local variables to a function. from graphics import * def drawDoor(x1, y1, x2, y2): '''draws a door with opposite corners at x1, y1 and x2, y2''' door = Rectangle(Point(x1, y1), Point(x2, y2)) door.setFill('red') door.draw(win) knob = Circle(Point(x2 - 10, (y1 + y2)//2), 3) knob.setFill('yellow') knob.draw(win) True or False: the function call is…arrow_forward
- Write a function DrivingCost with input parameters drivenMiles, milesPerGallon, and dollarsPerGallon, that returns the dollar cost to drive those miles. All items are of type double. If the function is called with 50 20.0 3.1599, the function returns 7.89975. Define that function in a program whose inputs are the car's miles/gallon and the gas dollars/gallon (both doubles). Output the gas cost for 10 miles, 50 miles, and 400 miles, by calling your DrivingCost function three times. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: printf("%0.21f", yourValue); Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call a function: double DrivingCost(double drivenMiles, double milesPerGallon, double dollarsPerGallon) 324758.2040686.qx3zqy7 LAB 5.18.1: LAB: Driving cost - functions 0/ 10 ACTIVITY main.c Load default template.. 1 #include 2 3 /* Define your function here */ 4 5 int main(void) {…arrow_forwardThis is a c programming question. Please solve with functions. Question The function that returns letter grade according to midterm and final grade: The function turn_to_letter decides on the letter grade of the student according to the table below from the visa and final grades sent into it and returns it, write it together with a main function which the turn_to_letter function is called and prints the letter equivalent on the screen. -Success score = 40% of midterm + 60% of final, -F for the success score below 50, D for between 50 and 59, C for between 60 and 69, B for between 70 and 79, A for 80 and above.arrow_forwardWrite a function called orbit that takes three inputs and returns three outputs. Our function is related to a planet that is in orbit around a star. Your function should take information about your planet’s orbit and tell us how long that orbit will take. Your three inputs should be: - The major axis radius, major. - The minor axis radius, minor. - The planet’s orbital velocity, velo, in meters per second. Your outputs should be the length of one year on that planet, in days, using three approximations for the perimeter of an ellipse.A circular approximation, circTime. - Using Ramanujan’s1 first approximation, ram1Time. - Using Ramanujan’s second approximation, ram2Timearrow_forward
- Write a function convertQuartersToDollars that has the noOfQuarters, noOfDollars, and remainingCents as formal parameters of data type int. The noOfQuarters is the number of quarters to be used to calculate noOfDollars, the number of dollars that the total number of quarters can be converted to, and remainingCents , the remaining cents. The noOfDollars and remainingCents are returned from the function,arrow_forwardPlease give me correct solution.arrow_forwardWrite a function driving_cost() with input parameters miles_per_gallon, dollars_per_gallon, and miles_driven, that returns the dollar cost to drive those miles. All items are of type float. The function called with arguments (20.0, 3.1599, 50.0) returns 7.89975. Define that function in a program whose inputs are the car's miles per gallon and the price of gas in dollars per gallon (both float). Output the gas cost for 10 miles, 50 miles, and 400 miles, by calling your driving_cost() function three times. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print (f {your_value:.2f}') Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call a function: def driving_cost (miles_per_gallon, dollars_per_gallon, miles_driven) 461710.3116374.qx3zqy7 LAB ACTIVITY 7.9.1: LAB: Driving costs - functions L23456 Define your function here. 4# Type your code here. main.py 0/10 Load default template...arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
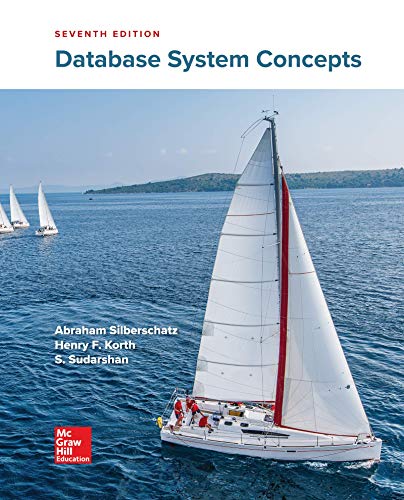
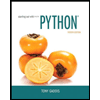
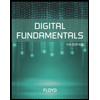
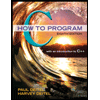
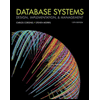
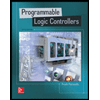