3.6.3 Snake Movements Whenever the Player makes a valid movement, the Snake will also move for one grid on the map. However, the Snake's movement is randomized. You have to use the random number generator function for the Snake to decide 1 out of 8 possible directions. If the Snake is at the edge of the map OR near the Walls, then some movements will be restricted. (Please be careful here. Moving the Snake outside the map might crash your program due to unauthorized out of bound memory access) * * * **** Figure 3: The Snake can move to 1 out of 8 directions. * *** Figure 4: If the Snake is at the edge/border of the map OR near the Walls, then the choice of movements will be reduced. Please ensure that the Snake does not move out of bound of the map. UCP Assignment Page 7 of 14 Semester 2, 2024 However, if the Player moves to a grid on which it is exactly ONE grid away from the Snake, then the Snake will just move to eat the Player without any random movement. P- (a) Player moves closer to the Snake. (b) Snake moves to eat the Player. Figure 5: The Snake will move in the direction of the Player only if the Player is one grid away from the Snake. (after Player movement) 15 20 36 0 0 0 10 3000 10000000000000 0 0002010000011111000 0 0 0 2 0 1 0 0 0 0 0 1 1 1 1 1 0 0 0 0 1100010000010001000 100000100000105000000 0001110000010001000 0 0 0 1 1 1 0 0 0 0 0 1 0 0 0 1 0 0 0 10000000000011111000 10000000000000000000 0 10000000000000000000 0 10000000001000000000 0 00000000010000111000 0000000111000000000 0 10000011100000000000 100000000000000000 40 Ө * P 0 * @ O 00000 *00 0 0 0 $ 000 00000 ** 0 000 000 000 0 Press w to move up Press s to move down Press a to move left Press d to move right Press u to undo
3.6.3 Snake Movements Whenever the Player makes a valid movement, the Snake will also move for one grid on the map. However, the Snake's movement is randomized. You have to use the random number generator function for the Snake to decide 1 out of 8 possible directions. If the Snake is at the edge of the map OR near the Walls, then some movements will be restricted. (Please be careful here. Moving the Snake outside the map might crash your program due to unauthorized out of bound memory access) * * * **** Figure 3: The Snake can move to 1 out of 8 directions. * *** Figure 4: If the Snake is at the edge/border of the map OR near the Walls, then the choice of movements will be reduced. Please ensure that the Snake does not move out of bound of the map. UCP Assignment Page 7 of 14 Semester 2, 2024 However, if the Player moves to a grid on which it is exactly ONE grid away from the Snake, then the Snake will just move to eat the Player without any random movement. P- (a) Player moves closer to the Snake. (b) Snake moves to eat the Player. Figure 5: The Snake will move in the direction of the Player only if the Player is one grid away from the Snake. (after Player movement) 15 20 36 0 0 0 10 3000 10000000000000 0 0002010000011111000 0 0 0 2 0 1 0 0 0 0 0 1 1 1 1 1 0 0 0 0 1100010000010001000 100000100000105000000 0001110000010001000 0 0 0 1 1 1 0 0 0 0 0 1 0 0 0 1 0 0 0 10000000000011111000 10000000000000000000 0 10000000000000000000 0 10000000001000000000 0 00000000010000111000 0000000111000000000 0 10000011100000000000 100000000000000000 40 Ө * P 0 * @ O 00000 *00 0 0 0 $ 000 00000 ** 0 000 000 000 0 Press w to move up Press s to move down Press a to move left Press d to move right Press u to undo
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
CAN YOU HELP ME WITH MAKING THE SNAKE MOVEMENT LOGIC MORE REFINED INSTEAD USING RANDOM DIRECTIONS I WANT IT TO USE A LOGIC TO CHASE THE PLAYER AND ALSO CAN YOU MAKE IT LOOK LIKE THE OUTPUT OF THIS PICTURE?
GAME.C:
#include "game.h"
#include
// Reads the map from the file and initializes the game state
void readMap(const char *filename, GameState *game) {
FILE*file=fopen(filename, "r");
if (!file) {
perror("Error opening map file");
exit(EXIT_FAILURE);
}
intnumRows, numCols, numWalls;
fscanf(file, "%d%d", &numRows, &numCols);
fscanf(file, "%d", &numWalls);
for (int i =0; i < MAX_ROWS; i++) {
for (int j =0; j < MAX_COLS; j++) {
int manhattanDistance =abs(game->playerPosition.x- i) +abs(game->playerPosition.y- j);
if (manhattanDistance <= visionRadius) {
if (i ==game->playerPosition.x&& j ==game->playerPosition.y) {
printf("P");
} else if (i == game->snakePosition.x && j == game->snakePosition.y) {
printf("~");
} else {
switch (game->map[i][j]) {
case0: printf("."); break; // Empty space
case1: printf("O"); break; // Wall
case2: printf("@"); break; // Lantern
case5: printf("$"); break; // Treasure
default: printf("?"); break;
}
}
} else {
printf(" "); // Print empty space for areas outside of vision
}
}
printf("\n");
}
#else
// Standard mode: print everything
for (inti=0; iplayerPosition.x&&j==game->playerPosition.y) {
printf("P");
} else if (i == game->snakePosition.x && j == game->snakePosition.y) {
printf("~");
} else {
switch (game->map[i][j]) {
case0: printf(" "); break;
case1: printf("O"); break;
case2: printf("@"); break;
case5: printf("$"); break;
default: printf("?"); break;
}
}
}
printf("\n");
}
#endif
}
// Handles player input and undo functionality
void handleInput(GameState *game, Node **undoStack, int *isGameOver) {
charinput=getchar();
intmoveMade=0;
switch (input) {
case'w': case'a': case's': case'd':
pushState(game, undoStack); // Save the current state for undo
movePlayer(input, game); // Move the player
moveSnake(game); // Move the snake after player moves
moveMade=1;
break;
case'u':
undoLastMove(game, undoStack);
moveMade=1;
break;
default:
printf("Invalid input! Use 'w', 'a', 's', 'd' for movement or 'u' for undo.\n");
break;
}
// Check for winning or losing conditions
if (moveMade) {
if (game->playerPosition.x==game->treasurePosition.x&&game->playerPosition.y==game->treasurePosition.y) {
system("clear");
printf("You win! You found the treasure!\n");
*isGameOver=1;
}
if (game->playerPosition.x==game->snakePosition.x&&game->playerPosition.y==game->snakePosition.y) {
system("clear");
printf("You lose! The snake caught you!\n");
*isGameOver=1;
}
}
}
// Moves the player according to the direction input ('w', 'a', 's', 'd')
void movePlayer(char direction, GameState *game) {
Position*player=&game->playerPosition;
intvalidMove=0;
switch (direction) {
case'w': if (player->x>0&&game->map[player->x-1][player->y] !=1) { player->x--; validMove=1; } break;
case's': if (player->xmap[player->x+1][player->y] !=1) { player->x++; validMove=1; } break;
case'a': if (player->y>0&&game->map[player->x][player->y-1] !=1) { player->y--; validMove=1; } break;
case'd': if (player->ymap[player->x][player->y+1] !=1) { player->y++; validMove=1; } break;
}
// Check if player has picked up the lantern
if (validMove&&player->x==game->lanternPosition.x&&player->y==game->lanternPosition.y) {
game->hasLantern=1;
game->map[player->x][player->y] =0; // Remove lantern from map
}
}
// Moves the snake randomly in 8 possible directions
void moveSnake(GameState *game) {
Position*snake=&game->snakePosition;
intdirection=randomDirection(); // Generates a random direction (0-7)
switch (direction) {
case0: if (snake->x>0&&game->map[snake->x-1][snake->y] !=1) snake->x--; break; // up
case1: if (snake->xmap[snake->x+1][snake->y] !=1) snake->x++; break; // down
case2: if (snake->y>0&&game->map[snake->x][snake->y-1] !=1) snake->y--; break; // left
case3: if (snake->ymap[snake->x][snake->y+1] !=1) snake->y++; break; // right
case4: if (snake->x>0&&snake->y>0&&game->map[snake->x-1][snake->y-1] !=1) { snake->x--; snake->y--; } break; // up-left
case5: if (snake->x>0&&snake->ymap[snake->x-1][snake->y+1] !=1) { snake->x--; snake->y++; } break; // up-right
case6: if (snake->xy>0&&game->map[snake->x+1][snake->y-1] !=1) { snake->x++; snake->y--; } break; // down-left
case7: if (snake->xymap[snake->x+1][snake->y+1] !=1) { snake->x++; snake->y++; } break; // down-right
}
}

Transcribed Image Text:3.6.3 Snake Movements
Whenever the Player makes a valid movement, the Snake will also move for one grid on the
map. However, the Snake's movement is randomized. You have to use the random number
generator function for the Snake to decide 1 out of 8 possible directions. If the Snake is at the
edge of the map OR near the Walls, then some movements will be restricted. (Please be careful
here. Moving the Snake outside the map might crash your program due to unauthorized out
of bound memory access)
*
*
*
****
Figure 3: The Snake can move to 1 out of 8 directions.
*
***
Figure 4: If the Snake is at the edge/border of the map OR near the Walls, then the choice of
movements will be reduced. Please ensure that the Snake does not move out of bound of the
map.
UCP Assignment
Page 7 of 14
Semester 2, 2024
However, if the Player moves to a grid on which it is exactly ONE grid away from the Snake,
then the Snake will just move to eat the Player without any random movement.
P-
(a) Player moves closer to the Snake.
(b) Snake moves to eat the Player.
Figure 5: The Snake will move in the direction of the Player only if the Player is one grid away
from the Snake. (after Player movement)

Transcribed Image Text:15 20
36
0
0
0
10 3000 10000000000000 0
0002010000011111000
0 0 0 2 0 1 0 0 0 0 0 1 1 1 1 1 0 0 0 0
1100010000010001000
100000100000105000000
0001110000010001000
0 0 0 1 1 1 0 0 0 0 0 1 0 0 0 1 0 0 0
10000000000011111000
10000000000000000000 0
10000000000000000000 0
10000000001000000000 0
00000000010000111000
0000000111000000000 0
10000011100000000000
100000000000000000 40
Ө
* P
0
* @ O
00000
*00
0
0
0 $
000
00000
**
0
000
000
000
0
Press w to move up
Press s to move down
Press a to move left
Press d to move right
Press u to undo
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
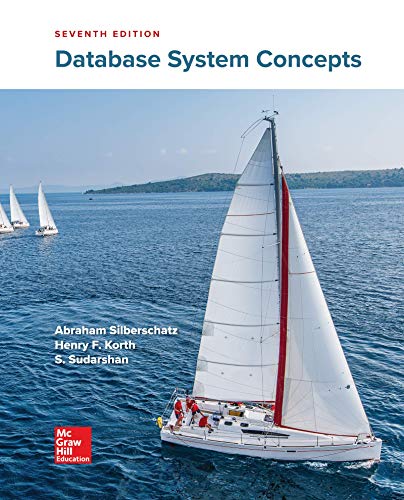
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
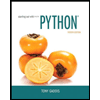
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
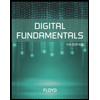
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
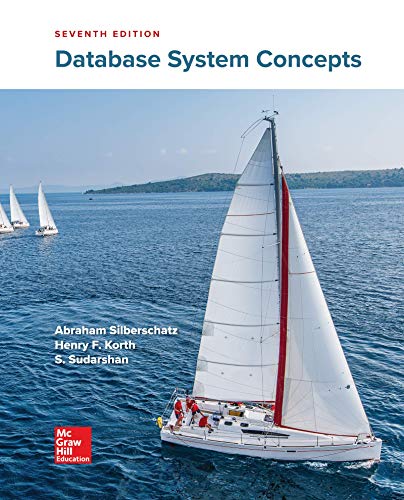
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
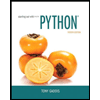
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
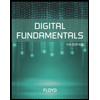
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
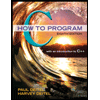
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
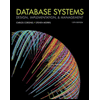
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
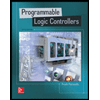
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education