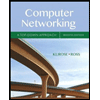
WHY DOES MY CODE APPEARS TO HAVE ERROR? PLEASE CHECK MY CODING (USING R PROGRAMMING)
#For Cuboid
cuboid <- function(l, w, h)
calcArea <- function(l, w, h)
{
Area <- 2*((l*w) +(l*h) +(w*h))
return(Area)
}
calcvol <- function(l, w, h)
{
vol <- l*w*h
return(vol)
}
#For cube
cube <- function(a)
calcArea <- function(a)
{
Area <- 6*a*a
return(Area)
}
calcvol <- function(a)
{
vol <- a*a*a
return(vol)
}
#For circular cylinder
cir_cylinder <- function(r, h)
calcArea <- function(r, h)
{
Area <- 2*3.14*r*(r+h)
return(Area)
}
calcvol <- function(r, h)
{
vol <- 3.14*r*r*h
return(vol)
}
#For circular cone
cir_cone <- function(s, h, r)
calcArea <- function(s, h, r)
{
Area <- 3.14*r*(r+s)
return(Area)
}
calcvol <- function(s, h, r)
{
vol <- ((3.14*r*r*h) /3)
return (vol)
}
#For Sphere
sphere <- function(r)
calcArea <- function(r)
{
Area <- 4*3.14*r*r
return(Area)
}
calcvol <- function(r)
{
vol <- (4/3) *3.14*r*r*r
return(vol)
}
#For hemisphere
hemi_sphere <- function(r)
calcArea<- function(r)
{
Area <- 3*3.14*r*r
return(Area)
}
calcvol <- function(r)
{
vol <- (2/3) *3.14*r*r*r
return(vol)
}
if(interactive()){
l<-as.numeric(readline(prompt="Enter the length (l) of the cuboid "))
w<-as.numeric(readline(prompt="Enter the width (w) of the cuboid "))
h<-as.numeric(readline(prompt="Enter the height (h) of the cuboid "))
print(paste("The area of the cuboid is ", calcArea(l, w, h)))
print(paste("The volume of the cuboid is ", calcvol(l, w, h)))
}
if(interactive()){
a<-as.numeric(readline(prompt="Enter the length (a) of the cube "))
print(paste("The area of the cube is ", calcArea(a)))
print(paste("The volume of the cube is ", calcvol(a)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the length (r) of the circular cylinder "))
h<-as.numeric(readline(prompt="Enter the height (h) of the circular cylinder "))
print(paste("The area of the circular cylinder is ", calcArea(r,h)))
print(paste("The volume of the circular cylinder is ", calcvol(r,h)))
}
if(interactive()){
s<-as.numeric(readline(prompt="Enter the length (s) of the circular cone "))
h<-as.numeric(readline(prompt="Enter the height (h) of the circular cone "))
r<-as.numeric(readline(prompt="Enter the radius (r) of the circular cone "))
print(paste("The area of the circular cone is ", calcArea(s, h, r)))
print(paste("The volume of the circular cone is ", calcvol(s, h, r)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the radius (r) of the sphere "))
print(paste("The area of the sphere is ", calcArea(r)))
print(paste("The volume of the sphere is ", calcvol(r)))
}
if(interactive()){
r<-as.numeric(readline(prompt="Enter the radius (r) of the hemi_sphere "))
print(paste("The area of the hemi_sphere is ", calcArea(r)))
print(paste("The volume of the hemi_sphere is ", calcvol(r)))
}
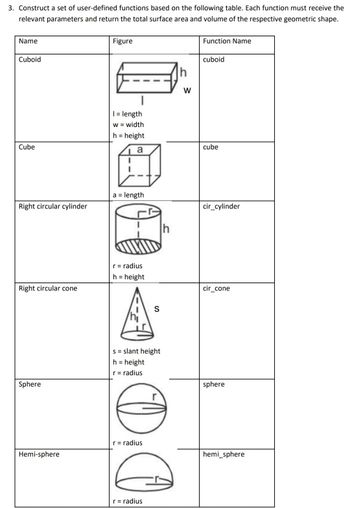
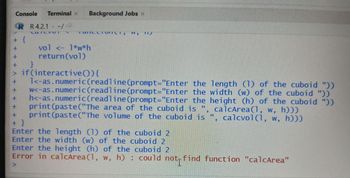

Step by stepSolved in 4 steps with 3 images

- C++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forwardIn c++ please help me answer this question I will give you a good rating :) implement the three versions of the addupto20() function: iterative, recursive, and divide-and-conquer approach test these functions with a few inputs from your main() Iterative solution * check if there exsits two numbers from vector data that add up to 20 e.g., if data=[2,5,3,15], the function returns true, as data [0] +data [3]==20 e.g., if data=[3,4,0,8], the function return false precondition: vector data has been initialized postcondition: if there are two numbers from list add up to 20, return true; otherwise, return false */ bool AddupTo20 (const vector‹int> & data){ } Come up with a recursive solution to the problem, following the hints given below: * check if there exsits two numbers from vector data[first...right] add up to 20 e.g., if data=[2,5,3,15], first=0, last=3, the function returns true, as data [0] +data [3]==20 e.g., if data=[2,5,3,15], first=2, last=3, the function returns…arrow_forwardChange the following in rstudio a<- c(1,2,3, "", 4) #to --->> a[1] 1 2 3 NA 4arrow_forward
- Consider the following C++ code segment: int d = 20; int f(int b) { static int c = 0; c *= b; return c; } int main() { int a; cin >> a; cout << f(a) * d << endl; }For each variable a, b, c, d: identify all type bindings and storage bindings for each binding, determine when the binding occurs identify the scope and lifetimearrow_forwardModify the function joinLine so that it right align the line to lengthlineLen by adding the appropriate number of space at the beginning ofthe line. (in Haskell) code: joinLine :: Line -> String joinLine [] = [] joinLine (w:ws) = w ++ " " ++ (joinLine ws)arrow_forwardData Structures and Algorithms in C/C++ a) Implement the addLargeNumbers function with the following prototype: void addLargeNumbers(const char *pNum1, const char *pNum2); This function should output the result of adding the two numbers passed in as strings. Here is an example call to this function with the expected output: /* Sample call to addLargeNumbers */ addLargeNumbers("592", "3784"); /* Expected output */ 4376 b) Implement a test program that demonstrates adding at least three pairs of large numbers (numbers larger than can be represented by a long). c) (1 point) Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant.arrow_forward
- 8.arrow_forwardOZ PROGRAMMING LANGUAGE Develop a function {MapTuple T F} that returns a tuple that has the same width and label as the tuple T with its fields mapped by the function F. For example, local fun {Sq X} X*X end in {MapTuple a(1 2 3) Sq} end should return a(1 4 9). Hint: A tuple is constructed with {MakeTuple L N}, where L is the label and N is the width of the tuple.arrow_forwardConsider the following structure definition. Write a C/C++ function that takes an array of struct student with its size and applies a curve system like if the student scored below 50, 1dd 10 points, if it is above 50, add 2 points. The lowest possible mark is 0 and the highest possible mark is 10. struct student{ int ID; int score; void applycurve (struct student a[ ], int size)arrow_forward
- I need this function to be defined using Haskell language. Hailstone sequences are produced by repeatedly applying the following mapping to numbers: an even number n maps to n/2 and an odd number m maps to 3m+1.The function hailmap applies this transformation to a single integer.hailmap :: Integer -> Integerhailmap n = undefined Note: when the function is called using an integer the answer will be given based on the number being odd or even. -- ghci> hailmap 7-- 22-- ghci> hailmap 8-- 4 Note: Use div rather than / for division, to avoid the need for conversion between numeric types.-- ghci> 15 `div` 2-- 7arrow_forwardin carrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
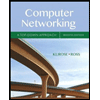
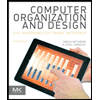
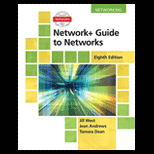
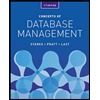
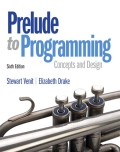
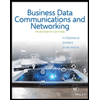