2. Team Class a. Variables i. ii. iii. iv. V. b. Methods i. ii. iii. iv. vi. Trainer -> String Gym ->String vii. Members ->arrayList of type pokemon saveFile ->a constant string that is the filename of "teamData.record" Accessor and Mutator methods for all variables setTeam ->sets all variables for a team 2 Constructors addMember 1. Add a pokemon to the members arraylist 2. readInput for such pokemon saveData 1. Writes all data from this class to the binary file saveFile loadData 1. Loads data from saveFile 2. Set the team using the setTeam method writeOutput 1. Prints all data for a team including printing all data for each pokemon in the members arraylist 2. Before printing pokemon data, ensure that you use Collections.sort(members) on the members arrayList. You can look up how this method works, but it should use the compare To that we set up in the pokemon class and sort the pokemon into alphabetical order before printing.
CREATE ANOTHER CLASS FOR TEAM
The CODE FOR POKEMON:
package com.company;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.util .*;
public class Pokemaon implements Comparable, Serializable, Fightable {
String name;
int level;
int health;
int attack;
String type;
//2 Constructors - 1 Default, 1 sets all variables
public Pokemaon(String name, int level, int health, int attack, String type) {
super();
this.name = name;
this.level = level;
this.health = health;
this.attack = attack;
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getLevel() {
return level;
}
public void setLevel(int level) {
this.level = level;
}
public int getHealth() {
return health;
}
public void setHealth(int health) {
this.health = health;
}
public int getAttack() {
return attack;
}
public void setAttack(int attack) {
this.attack = attack;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public String toString() {
return "Pokemaon [name=" + name + ", level=" + level + ", health=" + health + ", attack=" + attack + ", type="
+ type + "]";
}
@Override
public int compareTo(Object o) {
System.out.println("compareTo() method......");
return 0;
}
@Override
public void dealDamage() {
System.out.println("dealDamage() method......");
}
public static void main(String[] args) throws IOException, ClassNotFoundException {
Scanner sc1 = new Scanner(System.in);
Scanner sc2 = new Scanner(System.in);
System.out.println("Please enter the name..");
String name = sc1.nextLine();
System.out.println("Please enter the level..");
int lev = sc2.nextInt();
System.out.println("Please enter the health..");
int hel = sc2.nextInt();
System.out.println("Please enter the type..");
String type = sc1.nextLine();
System.out.println("Please enter the attack..");
int att = sc2.nextInt();
Pokemaon a = new Pokemaon(name, lev, hel, att, type);
FileOutputStream fos = new FileOutputStream("C:\\Users\\HP\\OneDrive\\Desktop\\xyz.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(a);
System.out.println("Data read successfully....");
}
}
.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

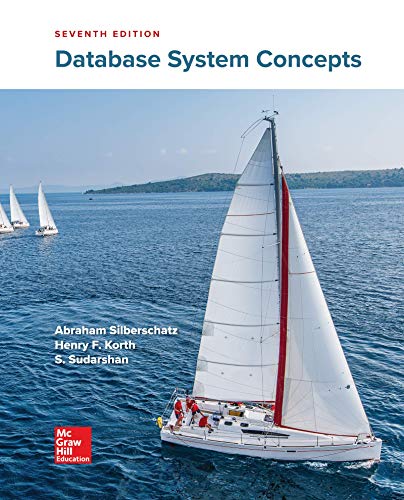
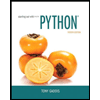
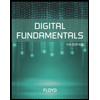
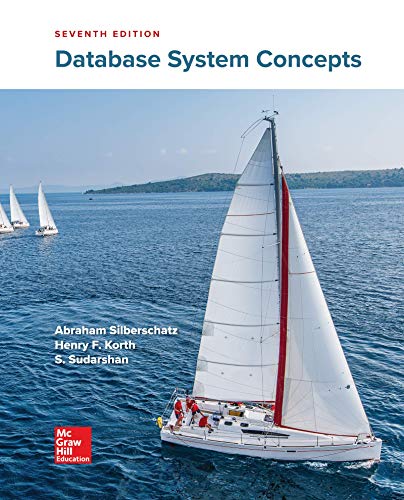
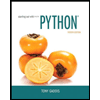
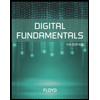
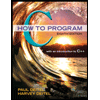
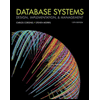
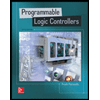