Please draw a UML class diagram for the following code: public class SudokuSolver { public static boolean solveSudoku(int[][] board) { int N = board.length; int[] emptyCell = findEmptyCell(board); if (emptyCell == null) { return true; } int row = emptyCell[0]; int col = emptyCell[1]; for (int num = 1; num <= 9; num++) { if (isValidMove(board, row, col, num)) { board[row][col] = num; if (solveSudoku(board)) { return true; } board[row][col] = 0; } } return false; } public static int[] findEmptyCell(int[][] board) { int N = board.length; for (int row = 0; row < N; row++) { for (int col = 0; col < N; col++) { if (board[row][col] == 0) { return new int[]{row, col}; } } } return null; } public static boolean isValidMove(int[][] board, int row, int col, int num) { return isValidRow(board, row, num) && isValidCol(board, col, num) && isValidRegion(board, row - row % 3, col - col % 3, num); } public static boolean isValidRow(int[][] board, int row, int num) { for (int col = 0; col < board.length; col++) { if (board[row][col] == num) { return false; } } return true; } public static boolean isValidCol(int[][] board, int col, int num) { for (int row = 0; row < board.length; row++) { if (board[row][col] == num) { return false; } } return true; } public static boolean isValidRegion(int[][] board, int startRow, int startCol, int num) { for (int row = 0; row < 3; row++) { for (int col = 0; col < 3; col++) { if (board[row + startRow][col + startCol] == num) { return false; } } } return true; } public static void printBoard(int[][] board) { int N = board.length; for (int row = 0; row < N; row++) { for (int col = 0; col < N; col++) { System.out.print(board[row][col] + " "); } System.out.println(); } } public static void main(String[] args) { int[][] puzzle = { {0, 0, 3, 0, 0, 8, 0, 2, 0}, {6, 0, 0, 3, 0, 0, 0, 0, 9}, {0, 9, 0, 7, 0, 0, 1, 0, 4}, {9, 0, 0, 2, 0, 0, 5, 4, 3}, {0, 8, 0, 4, 0, 1, 0, 0, 0}, {4, 6, 2, 0, 0, 7, 0, 0, 1}, {1, 0, 6, 0, 0, 3, 0, 9, 0}, {8, 0, 0, 0, 0, 9, 3, 0, 6}, {0, 3, 0, 6, 0, 0, 7, 0, 0} }; if (solveSudoku(puzzle)) { printBoard(puzzle); } else { System.out.println("No solution exists."); } } }
Please draw a UML class diagram for the following code:
public class SudokuSolver {
public static boolean solveSudoku(int[][] board) {
int N = board.length;
int[] emptyCell = findEmptyCell(board);
if (emptyCell == null) {
return true;
}
int row = emptyCell[0];
int col = emptyCell[1];
for (int num = 1; num <= 9; num++) {
if (isValidMove(board, row, col, num)) {
board[row][col] = num;
if (solveSudoku(board)) {
return true;
}
board[row][col] = 0;
}
}
return false;
}
public static int[] findEmptyCell(int[][] board) {
int N = board.length;
for (int row = 0; row < N; row++) {
for (int col = 0; col < N; col++) {
if (board[row][col] == 0) {
return new int[]{row, col};
}
}
}
return null;
}
public static boolean isValidMove(int[][] board, int row, int col, int num) {
return isValidRow(board, row, num) &&
isValidCol(board, col, num) &&
isValidRegion(board, row - row % 3, col - col % 3, num);
}
public static boolean isValidRow(int[][] board, int row, int num) {
for (int col = 0; col < board.length; col++) {
if (board[row][col] == num) {
return false;
}
}
return true;
}
public static boolean isValidCol(int[][] board, int col, int num) {
for (int row = 0; row < board.length; row++) {
if (board[row][col] == num) {
return false;
}
}
return true;
}
public static boolean isValidRegion(int[][] board, int startRow, int startCol, int num) {
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 3; col++) {
if (board[row + startRow][col + startCol] == num) {
return false;
}
}
}
return true;
}
public static void printBoard(int[][] board) {
int N = board.length;
for (int row = 0; row < N; row++) {
for (int col = 0; col < N; col++) {
System.out.print(board[row][col] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] puzzle = {
{0, 0, 3, 0, 0, 8, 0, 2, 0},
{6, 0, 0, 3, 0, 0, 0, 0, 9},
{0, 9, 0, 7, 0, 0, 1, 0, 4},
{9, 0, 0, 2, 0, 0, 5, 4, 3},
{0, 8, 0, 4, 0, 1, 0, 0, 0},
{4, 6, 2, 0, 0, 7, 0, 0, 1},
{1, 0, 6, 0, 0, 3, 0, 9, 0},
{8, 0, 0, 0, 0, 9, 3, 0, 6},
{0, 3, 0, 6, 0, 0, 7, 0, 0}
};
if (solveSudoku(puzzle)) {
printBoard(puzzle);
} else {
System.out.println("No solution exists.");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

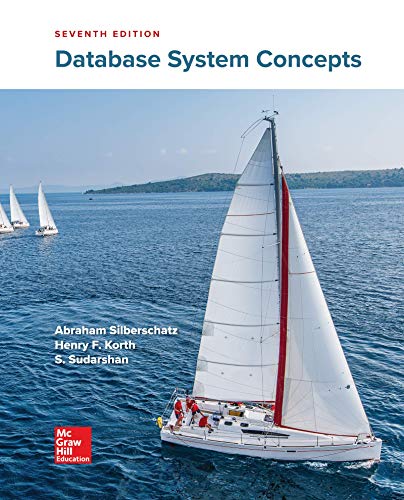
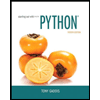
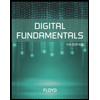
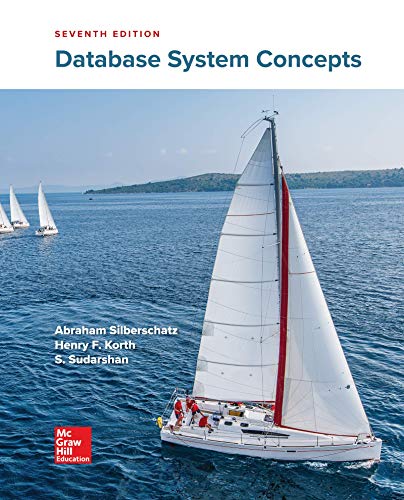
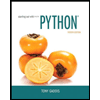
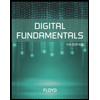
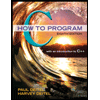
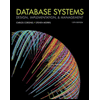
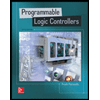