1i - Second Smallest Take an unknown number, larger than two, of positive integers as input. Assume that the first number is always smaller than the second, all numbers are unique and the input consists of at least three integers. Print the second smallest integer. Example: Please enter some numbers: 10 12 2 5 15 The second smallest number is: 5 Put your program in second_smallest.py. Use line.split(" ") to split the numbers in the input. 1j - Bubblesort Bubble sort is a sorting algorithm: when given a list, for example [4,2,6,8], it will sort the list to become sorted, [2,4,6,8]. Bubble sort works by walking through the list, comparing adjacent elements, and swapping them if they are in the wrong order. The process of walking through the list is repeated until we have traversed the entire list and no elements were swapped (this means that all adjacent elements are in the right order, and hence the list is sorted). Example run, let's see how this works on the numbers "5 1 4 2 8": First pass ( 5 1 4 2 8 ) → ( 1 5 4 2 8 ) Here, the algorithm compares the first two elements, and swaps since 5 > 1. ( 1 5 4 2 8 ) → ( 1 4 5 2 8 ) Swap since 5 > 4 ( 1 4 5 2 8 ) → ( 1 4 2 5 8 ) Swap since 5 > 2 ( 1 4 2 5 8 ) → ( 1 4 2 5 8 ) Now, since these elements are already in order (8 > 5), the algorithm does not swap them. Second pass ( 1 4 2 5 8 ) → ( 1 4 2 5 8 ) ( 1 4 2 5 8 ) → ( 1 2 4 5 8 ), Swap since 4 > 2 ( 1 2 4 5 8 ) → ( 1 2 4 5 8 ) ( 1 2 4 5 8 ) → ( 1 2 4 5 8 ) Third pass Now, the array is already sorted, but the algorithm does not know if it is completed. The algorithm needs one additional whole pass without any swap to know it is sorted. Implement the above algorithm. Example: Please enter some numbers: 123 45 78 6 5 48 38 98 [5, 6, 38, 45, 48, 78, 98, 123]
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
- Please do this in Python
Programming and provide screenshot - The output should be exact same like in the examples.
- If the output in the example is in 2 decimals, then it should be like that.
1i - Second Smallest
Take an unknown number, larger than two, of positive integers as input. Assume that the first
number is always smaller than the second, all numbers are unique and the
input consists of at least three integers. Print the second smallest integer.
Example:
Please enter some numbers: 10 12 2 5 15
The second smallest number is: 5
Put your program in second_smallest.py. Use line.split(" ") to split the numbers in the input.
1j - Bubblesort
Bubble sort is a sorting
Example run, let's see how this works on the numbers "5 1 4 2 8":
First pass
( 5 1 4 2 8 ) → ( 1 5 4 2 8 ) Here, the algorithm compares the first two elements, and swaps since 5 > 1.
( 1 5 4 2 8 ) → ( 1 4 5 2 8 ) Swap since 5 > 4
( 1 4 5 2 8 ) → ( 1 4 2 5 8 ) Swap since 5 > 2
( 1 4 2 5 8 ) → ( 1 4 2 5 8 ) Now, since these elements are already in order (8 > 5), the algorithm does not swap them.
Second pass
( 1 4 2 5 8 ) → ( 1 4 2 5 8 )
( 1 4 2 5 8 ) → ( 1 2 4 5 8 ), Swap since 4 > 2
( 1 2 4 5 8 ) → ( 1 2 4 5 8 )
( 1 2 4 5 8 ) → ( 1 2 4 5 8 )
Third pass
Now, the array is already sorted, but the algorithm does not know if it is completed. The algorithm needs one additional whole pass without any swap to know it is sorted.
Implement the above algorithm.
Example:
Please enter some numbers: 123 45 78 6 5 48 38 98
[5, 6, 38, 45, 48, 78, 98, 123]
Please put your program in bubble_sort.py
Bubble sort is actually a quite inefficient way of sorting: it takes a lot of steps compared to other algorithms such as mergesort.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

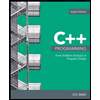
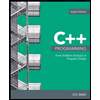