15) Write instructions that first clear bits 0 and 1 in AL. Then, if the destination operand is equal to zero, the code should jump to label L3. Otherwise, it should jump to label L4.
15) Write instructions that first clear bits 0 and 1 in AL. Then, if the destination operand is equal to zero, the code should jump to label L3. Otherwise, it should jump to label L4.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question

Transcribed Image Text:**Question 15: Conditional Bit Manipulation and Jump in Assembly**
Write instructions that first clear bits 0 and 1 in the AL register. Then, if the destination operand is equal to zero, the code should jump to label L3. Otherwise, it should jump to label L4.
---
**Explanation:**
1. **Clearing Bits 0 and 1 in AL:**
- This can be achieved using the bitwise AND operation with a mask where bits 0 and 1 are 0.
2. **Conditional Jump:**
- After clearing the bits, check if the resulting value in AL is zero.
- If AL is zero, jump to label L3.
- Otherwise, jump to label L4.
**Example Code (Assembly Language):**
```asm
; Clear bits 0 and 1 in AL
AND AL, 11111100b ; Mask where bits 0 and 1 are 0, others are 1
; Compare AL with zero
CMP AL, 0
; Conditional jumps
JE L3 ; Jump to label L3 if AL is zero (equal)
JMP L4 ; Otherwise, jump to label L4 (since JE not taken)
```
**Explanation of the Code:**
1. `AND AL, 11111100b`:
- The `AND` operation uses the mask `11111100b` (binary notation) to clear the last two bits of the AL register while keeping the other bits unchanged.
2. `CMP AL, 0`:
- This instruction compares the value in the AL register with zero.
3. `JE L3`:
- The `JE` (Jump if Equal) instruction will jump to label `L3` if the comparison result is zero.
4. `JMP L4`:
- The `JMP` instruction will unconditionally jump to label `L4`. It is executed if the previous comparison (`CMP AL, 0`) is not zero.
This sequence ensures that bits 0 and 1 in AL are cleared first, and then it conditionally jumps to the appropriate label based on the value in AL.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
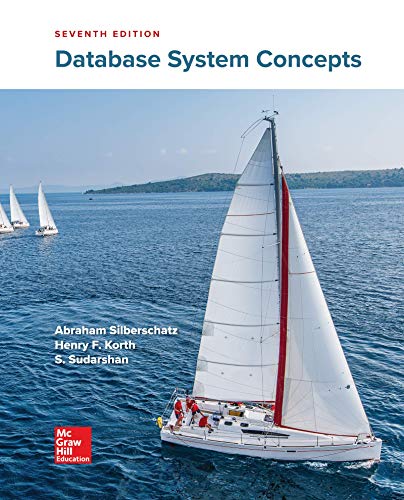
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
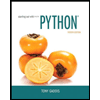
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
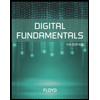
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
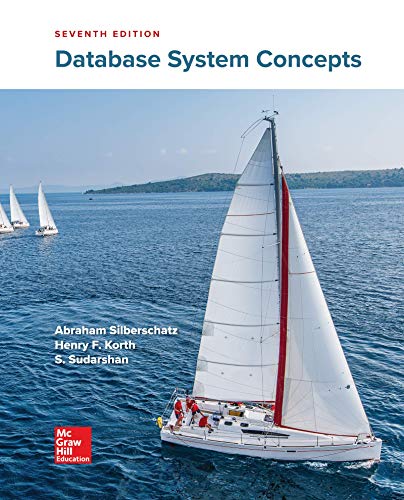
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
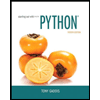
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
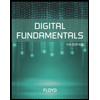
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
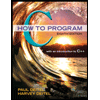
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
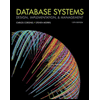
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
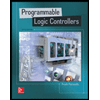
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education