13.5 LAB: Zip code and population (class templates) Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): * vector> zipCodeState: ZIP code - state abbreviation pairs * vector> abbrevState: state abbreviation - state name pairs * vector> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 I put the TODO in bold which are the ones I need help with. main.cpp: #include #include #include #include #include "StatePair.h" using namespace std; int main() { ifstream inFS; // File input stream int zip; int population; string abbrev; string state; unsigned int i; // ZIP code - state abbrev. pairs vector> zipCodeState; // state abbrev. - state name pairs vector> abbrevState; // state name - population pairs vector> statePopulation; // Fill the three ArrayLists // Try to open zip_code_state.txt file inFS.open("zip_code_state.txt"); if (!inFS.is_open()) { cout << "Could not open file zip_code_state.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; inFS >> zip; if (!inFS.fail()) { temp.SetKey(zip); } inFS >> abbrev; if (!inFS.fail()) { temp.SetValue(abbrev); } zipCodeState.push_back(temp); } inFS.close(); // Try to open abbreviation_state.txt file inFS.open("abbreviation_state.txt"); if (!inFS.is_open()) { cout << "Could not open file abbreviation_state.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; inFS >> abbrev; if (!inFS.fail()) { temp.SetKey(abbrev); } getline(inFS, state); //flushes endline getline(inFS, state); state = state.substr(0, state.size()-1); if (!inFS.fail()) { temp.SetValue(state); } abbrevState.push_back(temp); } inFS.close(); // Try to open state_population.txt file inFS.open("state_population.txt"); if (!inFS.is_open()) { cout << "Could not open file state_population.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; getline(inFS, state); state = state.substr(0, state.size()-1); if (!inFS.fail()) { temp.SetKey(state); } inFS >> population; if (!inFS.fail()) { temp.SetValue(population); } getline(inFS, state); //flushes endline statePopulation.push_back(temp); } inFS.close(); cin >> zip; for (i = 0; i < zipCodeState.size(); ++i) { // TODO: Using ZIP code, find state abbreviation } for (i = 0; i < abbrevState.size(); ++i) { // TODO: Using state abbreviation, find state name } for (i = 0; i < statePopulation.size(); ++i) { // TODO: Using state name, find population. Print pair info. } } StatePair.h: #ifndef STATEPAIR #define STATEPAIR template class StatePair { // TODO: Define constructors StatePair(); StatePair(T1 userKey, T2 userValue); // TODO: Define mutators, and accessors for StatePair // TODO: Define PrintInfo() method }; #endif
13.5 LAB: Zip code and population (class templates) Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): * vector> zipCodeState: ZIP code - state abbreviation pairs * vector> abbrevState: state abbreviation - state name pairs * vector> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 I put the TODO in bold which are the ones I need help with. main.cpp: #include #include #include #include #include "StatePair.h" using namespace std; int main() { ifstream inFS; // File input stream int zip; int population; string abbrev; string state; unsigned int i; // ZIP code - state abbrev. pairs vector> zipCodeState; // state abbrev. - state name pairs vector> abbrevState; // state name - population pairs vector> statePopulation; // Fill the three ArrayLists // Try to open zip_code_state.txt file inFS.open("zip_code_state.txt"); if (!inFS.is_open()) { cout << "Could not open file zip_code_state.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; inFS >> zip; if (!inFS.fail()) { temp.SetKey(zip); } inFS >> abbrev; if (!inFS.fail()) { temp.SetValue(abbrev); } zipCodeState.push_back(temp); } inFS.close(); // Try to open abbreviation_state.txt file inFS.open("abbreviation_state.txt"); if (!inFS.is_open()) { cout << "Could not open file abbreviation_state.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; inFS >> abbrev; if (!inFS.fail()) { temp.SetKey(abbrev); } getline(inFS, state); //flushes endline getline(inFS, state); state = state.substr(0, state.size()-1); if (!inFS.fail()) { temp.SetValue(state); } abbrevState.push_back(temp); } inFS.close(); // Try to open state_population.txt file inFS.open("state_population.txt"); if (!inFS.is_open()) { cout << "Could not open file state_population.txt." << endl; return 1; // 1 indicates error } while (!inFS.eof()) { StatePair temp; getline(inFS, state); state = state.substr(0, state.size()-1); if (!inFS.fail()) { temp.SetKey(state); } inFS >> population; if (!inFS.fail()) { temp.SetValue(population); } getline(inFS, state); //flushes endline statePopulation.push_back(temp); } inFS.close(); cin >> zip; for (i = 0; i < zipCodeState.size(); ++i) { // TODO: Using ZIP code, find state abbreviation } for (i = 0; i < abbrevState.size(); ++i) { // TODO: Using state abbreviation, find state name } for (i = 0; i < statePopulation.size(); ++i) { // TODO: Using state name, find population. Print pair info. } } StatePair.h: #ifndef STATEPAIR #define STATEPAIR template class StatePair { // TODO: Define constructors StatePair(); StatePair(T1 userKey, T2 userValue); // TODO: Define mutators, and accessors for StatePair // TODO: Define PrintInfo() method }; #endif
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 5PE
Related questions
Question
13.5 LAB: Zip code and population (class templates)
Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main():
* vector> zipCodeState: ZIP code - state abbreviation pairs
* vector> abbrevState: state abbreviation - state name pairs
* vector> statePopulation: state name - population pairs
Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair.
Ex: If the input is:
21044
the output is:
Maryland: 6079602
I put the TODO in bold which are the ones I need help with.
main.cpp:
#include
#include
#include
#include
#include "StatePair.h"
using namespace std;
int main() {
ifstream inFS; // File input stream
int zip;
int population;
string abbrev;
string state;
unsigned int i;
// ZIP code - state abbrev. pairs
vector> zipCodeState;
// state abbrev. - state name pairs
vector> abbrevState;
// state name - population pairs
vector> statePopulation;
// Fill the three ArrayLists
// Try to open zip_code_state.txt file
inFS.open("zip_code_state.txt");
if (!inFS.is_open()) {
cout << "Could not open file zip_code_state.txt." << endl;
return 1; // 1 indicates error
}
while (!inFS.eof()) {
StatePair temp;
inFS >> zip;
if (!inFS.fail()) {
temp.SetKey(zip);
}
inFS >> abbrev;
if (!inFS.fail()) {
temp.SetValue(abbrev);
}
zipCodeState.push_back(temp);
}
inFS.close();
// Try to open abbreviation_state.txt file
inFS.open("abbreviation_state.txt");
if (!inFS.is_open()) {
cout << "Could not open file abbreviation_state.txt." << endl;
return 1; // 1 indicates error
}
while (!inFS.eof()) {
StatePair temp;
inFS >> abbrev;
if (!inFS.fail()) {
temp.SetKey(abbrev);
}
getline(inFS, state); //flushes endline
getline(inFS, state);
state = state.substr(0, state.size()-1);
if (!inFS.fail()) {
temp.SetValue(state);
}
abbrevState.push_back(temp);
}
inFS.close();
// Try to open state_population.txt file
inFS.open("state_population.txt");
if (!inFS.is_open()) {
cout << "Could not open file state_population.txt." << endl;
return 1; // 1 indicates error
}
while (!inFS.eof()) {
StatePair temp;
getline(inFS, state);
state = state.substr(0, state.size()-1);
if (!inFS.fail()) {
temp.SetKey(state);
}
inFS >> population;
if (!inFS.fail()) {
temp.SetValue(population);
}
getline(inFS, state); //flushes endline
statePopulation.push_back(temp);
}
inFS.close();
cin >> zip;
for (i = 0; i < zipCodeState.size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
}
for (i = 0; i < abbrevState.size(); ++i) {
// TODO: Using state abbreviation, find state name
}
for (i = 0; i < statePopulation.size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
}
StatePair.h:
#ifndef STATEPAIR
#define STATEPAIR
template
class StatePair {
// TODO: Define constructors
StatePair();
StatePair(T1 userKey, T2 userValue);
// TODO: Define mutators, and accessors for StatePair
// TODO: Define PrintInfo() method
};
#endif

Transcribed Image Text:6482.1116448
LAB
13.5.1: LAB: Zip code and population (class templates)
АCTIVITY
Current file: StatePair.h -
1 #ifndef STATEPAIR
2 #define STATEPAIR
3
4 template<typename T1, typename T2>
5 class StatePair {
7 // TODO: Define constructors
StatePair();
StatePair(T1 userKey, T2 userValue);
8
10
11 // TODO: Define mutators, and accessors for StatePair
12
13 // TODO: Define PrintInfo() method
14 };
15
16 #endif
foro out

Transcribed Image Text:AB
АCTIVITY
13.5.1: LAB: Zip code and population (class templates)
Current file: main.cpp
91
92
cin >> zip;
93
for (i 0; i < zipCodeState.size(); ++i) {
// TODO: Using ZIP code, find state abbreviation
94
95
96
97
98
for (i - 0; i < abbrevState.size(); ++i) { I
// TODO: Using state abbreviation, find state name
99
100
101
102
103
for (i - 0; i < statePopulation.size(); ++i) {
// TODO: Using state name, find population. Print pair info.
}
104
105
106
107
108 }
Run your program as often as you'd like, before submitt
input values in the first box, then click Run program and
Develop mode
Submit mode
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
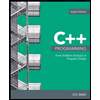
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
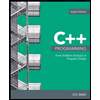
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning