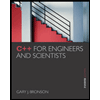
complete the following Horse Class with the guidelines provided below
public class Horse {
// Fields of class Horse
// Constructor of class Horse
/**
* Constructor for objects of class Horse
*/
public Horse(char horseSymbol, String horseName, double horseConfidence) {
}
// Other methods of class Horse
public void fall() {
}
public double getConfidence() {
}
public int getDistanceTravelled() {
}
public String getName() {
}
public char getSymbol() {
}
public void goBackToStart() {
}
public boolean hasFallen() {
}
public void moveForward() {
}
public void setConfidence(double newConfidence) {
}
public void setSymbol(char newSymbol) {
}
}
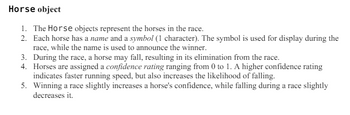
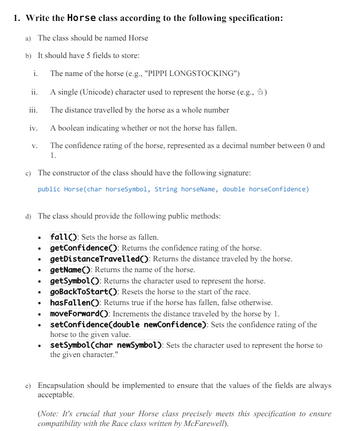

Step by stepSolved in 2 steps

- Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forwardMark the following statements as true or false. The member variables of a class must be of the same type. (1) The member functions of a class must be public. (2) A class can have more than one constructor. (5) A class can have more than one destructor. (5) Both constructors and destructors can have parameters. (5)arrow_forwardA(n) ____________ is one instance or variable of a class.arrow_forward
- Chapter 10 defined the class circleType to implement the basic properties of a circle. (Add the function print to this class to output the radius, area, and circumference of a circle.) Now every cylinder has a base and height, where the base is a circle. Design a class cylinderType that can capture the properties of a cylinder and perform the usual operations on the cylinder. Derive this class from the class circleType designed in Chapter 10. Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Also, write a program to test various operations on a cylinder.arrow_forwardIn previous chapters, you have created programs for the Greenville Idol competition. Now create a Contestant class with the following characteristics: The Contestant class contains public static arrays that hold talent codes and descriptions. Recall that the talent categories are Singing Dancing, Musical instrument, and Other. The class contains an auto-implemented property that holds a contestants name. The class contains fields for a talent code and description. The set accessor for the code assigns a code only if it is valid. Otherwise, it assigns I for Invalid. The talent description is a read-only property that is assigned a value when the code is set. Modify the GreenvilleRevenue program so that it uses the Contestant class and performs the following tasks: The program prompts the user for the number of contestants in this years competition; the number must be between 0 and 30. The program continues to prompt the user until a valid value is entered. The expected revenue is calculated and displayed. The revenue is $25 per contestant. The program prompts the user for names and talent codes for each contestant entered. Along with the prompt for a talent code, display a list of the valid categories. After data entry is complete, the program displays the valid talent categories and then continuously prompts the user for talent codes and displays the names of all contestants in the category. Appropriate messages are displayed if the entered code is not a character or a valid code.arrow_forwardIndicate whether each of the following C# programming language identifiers is legal or illegal. If it is legal, indicate whether it is a conventional identifier for a class. electricBill ElectricBill Electric bill Static void #ssn Ay56we Theater_Tickets 212AreaCode heightInCentimeters Zip23891 Voidarrow_forward
- Some of the characteristics of a book are the title, author(s), publisher, ISBN, price, and year of publication. Design a class bookType that defines the book as an ADT. Each object of the class bookType can hold the following information about a book: title, up to four authors, publisher, ISBN, price, and number of copies in stock. To keep track of the number of authors, add another member variable. Include the member functions to perform the various operations on objects of type bookType. For example, the usual operations that can be performed on the title are to show the title, set the title, and check whether a title is the same as the actual title of the book. Similarly, the typical operations that can be performed on the number of copies in stock are to show the number of copies in stock, set the number of copies in stock, update the number of copies in stock, and return the number of copies in stock. Add similar operations for the publisher, ISBN, book price, and authors. Add the appropriate constructors and a destructor (if one is needed). Write the definitions of the member functions of the class bookType. Write a program that uses the class bookType and tests various operations on the objects of the class bookType. Declare an array of 100 components of type bookType. Some of the operations that you should perform are to search for a book by its title, search by ISBN, and update the number of copies of a book.arrow_forward1. Mark the following statements as true or false. a. An identifier must start with a letter and can be any sequence of characters. (1) b. In C++, there is no difference between a reserved word and a predefined identifier. (1) c. A C++ identifier cannot start with a digit. (1) d. The collating sequence of a character is its preset number in the character data set. (2) e. Only one of the operands of the modulus operator needs to be of type int. (3) f. If ; and ;, then after the statement ; the value of b is erased. (6) g. If the input is 7 and x is a variable of type int, then the statement ; assigns the value 7 to x. (6) h. In an output statement, the newline character may be a part of the string. (10) i. In C++, all variables must be initialized when they are declared. (7) j. In a mixed expression, all the operands are converted to floating-point numbers. (4) k. Suppose . After the statement ; executes, y is 5 and x is 6. (9) i. Suppose . After the statement ; executes, the value of a is still 5 because the value of the expression is not saved in another variable. (9)arrow_forward(Data processing) A bank’s customer records are to be stored in a file and read into a set of arrays so that a customer’s record can be accessed randomly by account number. Create the file by entering five customer records, with each record consisting of an integer account number (starting with account number 1000), a first name (maximum of 10 characters), a last name (maximum of 15 characters), and a double-precision number for the account balance. After the file is created, write a C++ program that requests a user-input account number and displays the corresponding name and account balance from the file.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
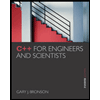
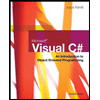
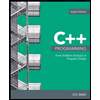
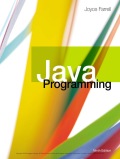
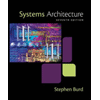