1. Write a program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog; the user input is in color: Please enter an integer between 1,000 and 999,999: 23,456 23456 Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract substrings consisting of the first n - 4 characters and the last three characters. 2. Writing large letters. A large letter H can be produced like this: It can be declared as a string literal like this: *n* final string LETTER_H *n*****n* *n* *%n"; Print the string with System.out.printf. The %n format specifiers cause line breaks in the output. Do the same for the letters E, L, and O. Then write in large letters: H
1. Write a program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog; the user input is in color: Please enter an integer between 1,000 and 999,999: 23,456 23456 Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract substrings consisting of the first n - 4 characters and the last three characters. 2. Writing large letters. A large letter H can be produced like this: It can be declared as a string literal like this: *n* final string LETTER_H *n*****n* *n* *%n"; Print the string with System.out.printf. The %n format specifiers cause line breaks in the output. Do the same for the letters E, L, and O. Then write in large letters: H
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:6. Write a program that reads in an integer and
breaks it into a sequence of individual digits. For
example, the input 16384 is displayed as
16384
You may assume that the input has no more
than five digits and is not negative.
7. Decimal to hex. Write a program that prompts
the user to enter an integer between 0 and 15
and displays its corresponding hex number.
Here are some sample runs (user input in color):
Enter a decimal value (0 to 15): 11
The hex value is B
Enter a decimal value (0 to 15): 5
The hex value is 5
8. Convert number grade to letter. Write a
program that prompts the user to enter a
numeric grade 4, 3, 2, 1, or 0 and displays its
corresponding letter value A, B, C, D, or F.
Here is a sample run (user input in color):
Enter a numeric grade: 3
The letter value for grade 3 is B

Transcribed Image Text:1. Write a program that reads a number between
1,000 and 999,999 from the user, where the
user enters a comma in the input. Then print
the number without a comma. Here is a sample
dialog; the user input is in color:
Please enter an integer between 1,000
and 999,999: 23,456
23456
Hint: Read the input as a string. Measure the
length of the string. Suppose it contains n
characters. Then extract substrings consisting of
the first n - 4 characters and the last three
characters.
2. Writing large letters. A large letter H can be
produced like this:
*
It can be declared as a string literal like this:
final string LETTER_H = "* *%n*
*n*****n* *n* *%n";
Print the string with System.out.printf. The
en format specifiers cause line breaks in the
output. Do the same for the letters E, L, and O.
Then write in large letters:
O
3. Write a program that transforms numbers 1, 2,
3,..., 12 into the corresponding month names
January, February, March, ..., December.
Hint: Make a very long string "January February
March...", in which you add spaces such that
each month name has the same length. Then
use substring to extract the month you want.
4. Printing a grid. Write a program that prints the
following grid to play tic-tac-toe.
+--+--+--+
||||
+--+--+--+
||||
+-+-+-+
+-+-+-+
Of course, you could simply write seven
statements of the form
System.out.println("+--+--+--
+");
You should do it the smart way, though. Declare
string variables to hold two kinds of patterns: a
comb-shaped pattern and the bottom line. Print
the comb three times and the bottom line once.
5. Write a program that prints a Christmas tree, as
shown below. Remember to use escape
sequences.
1
H E L L ^
11
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
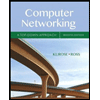
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
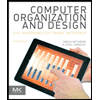
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
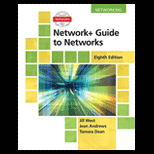
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
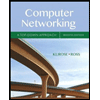
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
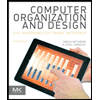
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
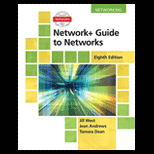
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
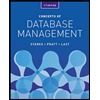
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
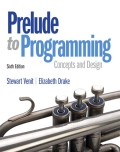
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
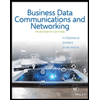
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY