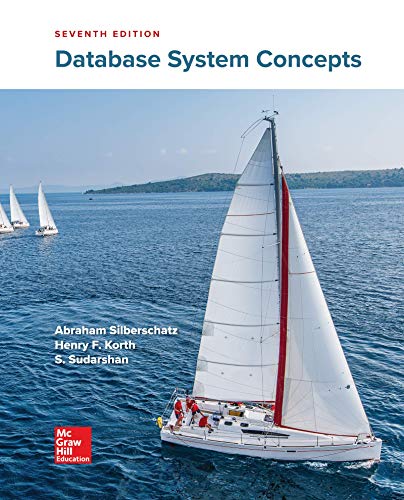
Concept explainers
1. Write a Java application in a project called Alphabet. It should print the uppercase
alphabet, three letters per line. The first, third, fifth, etc. lines should be indented one
space. The final line contains only Y and Z. The output should look exactly as follows.
ABC
DEF
GHI
JKL
MNO
PQR
STU
VWX
YZ
Place a header comment in each program that includes your name, the assignment
the number and a description of what the program does.
2. Write a Java application in a project called Falling. It inputs a distance in meters from
the user and computes the amount of time for the object falling from that distance to hit
the ground and the velocity of the object just before impact. Air resistance is discounted
(assumed to fall in a vacuum).
To compute the time, use the formula:
t = Square Root (2d / g)
where d is the distance in meters and g is the acceleration due to gravity on earth (use
9.807 meters/sec2
). The time is measured in seconds.
To compute the velocity, use the formula:
v = Square Root (2dg)
The velocity is measured in meters per second.
A sample run is shown on the next page. Computed values should be printed with two
digits after the decimal. The spacing and text should follow the example exactly.
Place a header comment in this program that includes your name, the assignment number
and a description of what the program does. Comment each variable indicating what it is
used for in the program.
Problem 2 Sample Run (input shown in bold):
Enter the distance: 100
Distance: 100.0
Time: 4.52 seconds
Velocity: 44.29 meters/second

/*******************************************************************************/
///beginning
public class Main
{
public static void main(String[] args) {
char ch;
int i=1,j=1;
///First blank space
System.out.print(" ");
for( ch = 'A' ; ch <= 'Z' ; ch++ ){
////This will print each character
System.out.print(ch);
if(i==3){
///condition to switch to new line
System.out.println(" ");
if(j%2==0){
//condition to take space in new line
System.out.print(" ");
}
i=0;
j=j+1;
}
i=i+1;
}
}
}
///////////end
Output:
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Java Program 1.Carmens’s Catering provides meals for parties and special events. Write a java program that displays Carmen’s motto. Which is “Carmen’s makes the food that make it a party”. Save the file as CarmensMotto.java. Create a second java program that displays the motto surrounded by border composed of asterisks. Save the file as CarmensMottot2.java.arrow_forwardC# programming question 3: I’m not sure how to do this question. Help of any input, output / comments would be appreciated.arrow_forwardJava Programming: Question 12 Not sure how to do this question. Help of any input and output would be appreciated.arrow_forward
- in phython language Create a package folder named shapes. Inside this package, place two more package folders: 2d and 3d. Inside 2d package, place a module file: circle.py circle.py module has two function definitions: areaCircle(), circCircle(). areaCircle() function takes a parameter: radius, calculates and returns the area of the circle. You may assume PI is 3.14. circCircle() function also takes a parameter: radius, calculates and returns the circumference of the circle. Inside 3d package, place two module files: sphere.py and cylindir.py sphere.py module has two function definitions: areaSphere() and volumeSphere() which both take a parameter for radius, calculates and returns area and volume of a sphere respectively. cylindir.py module has two function definitions: areaCylindir() and volumeCylindir() which both take two parameters: height and radius. They calculate area and volume of a cylindir and return the values respectively. You may find the formulas for those shapes…arrow_forward1. Design a java application that outputs a table with a list of at least 6bstudents together with their first name, last name, score and grade in the following format. First name last name score grade 2 Write a program by entering two integer numbers of your choice and make sure your program works properly. When you are readyvto submit your project enter 24 and 53 as set values and generate 100 random numbers to makecsure all of these numbers are generated within the range of [24.....43] inclusive. Please I need help to write the programs. Thanksarrow_forwardCompatible with visual studios - c# Word SeparatorCreate an application that accepts as input a sentence in which all the words are runtogether but the first character of each word is uppercase. Convert the sentence to astring in which the words are separated by spaces and only the first word starts withan uppercase letter. For example, the string "StopAndSmellTheRoses." would beconverted to "Stop and smell the roses."arrow_forward
- Project: Two Formulas in Separate Class Write two formulas your choice in separate file and you call it in some manner from your from main program. In Java the pic for examplearrow_forwardmy assignment wants me to Convert the MileConversions program to an interactive application. Instead of assigning a value to the miles variable, accept it from the user as input. Something is wrong with my code My code is : { public static void main(String[] args) { Scanner inputDevice = new Scanner(System.in); final double INCHES_IN_MILE = 63360; final double FEET_IN_MILE = 5280; final double YARDS_IN_MILE = 1760; double miles = 4; double in, ft, yds; in = miles * INCHES_IN_MILE; ft = miles * FEET_IN_MILE; yds = miles * YARDS_IN_MILE; System.out.println(miles + " miles is " + in + " inches, or " + ft + " feet, or " + yds + " yards"); } }arrow_forwardC++. Please do not change the existing code. The instructions are in the image that is provided. Please zoom in or you can download the png file. Thank you! Time.cpp #include "Time.h" //Default Constructor //Constructor with parameters int Time::getHour() const { return hour; }int Time::getMinute() const { return minute; }int Time::getSecond() const { return second; }void Time::setHour(int h) { hour = h; }void Time::setMinute(int m) { minute = m; }void Time::setSecond(int s) { second = s; } int Time::timeToSeconds() const{ return (getSecond() + getMinute() * 60 + getHour() * 3600);} const Time Time::secondsToTime(int s) const{ int resultS = s % 60; s /= 60; int resultM = s % 60; s /= 60; int resultH = s % 24; return Time(resultH, resultM, resultS);} //toString // + // - // < // > // == ------ Time.h #ifndef TIME#define TIME #include <string> using namespace std; class Time{private: int hour; int minute; int second; int timeToSeconds() const;…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
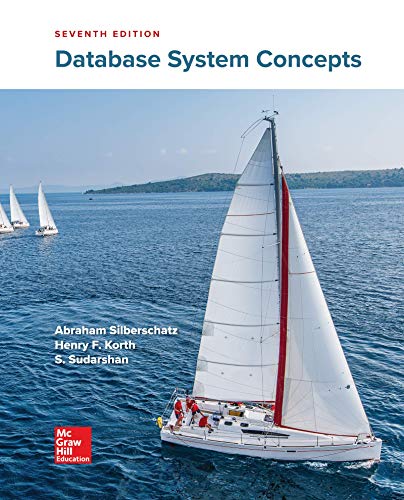
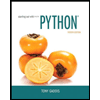
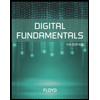
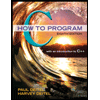
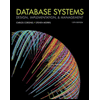
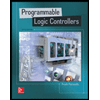