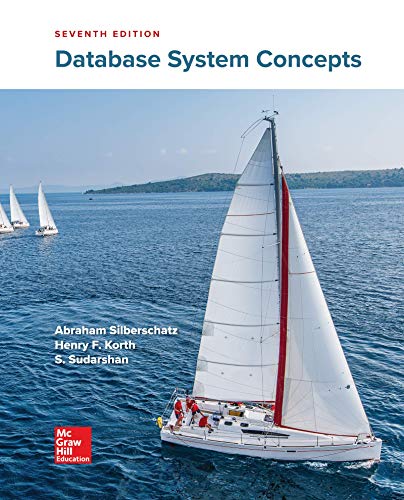
Create a program for Smalltown Regional Airport Flights that accepts either an integer flight number or string airport code from the options in Figure 8-33.
Pass the user’s entry to one of two overloaded GetFlightInfo() methods, and then display a returned string with all the flight details. For example, if 201 was input, the output would be: Flight #201 AUS Austin Scheduled at: 0710 (note that there should be two spaces between 'Austin' and 'Scheduled').
The method version that accepts an integer looks up the airport code, name, and time of flight; the version that accepts a string description looks up the flight number, airport name, and time.
The methods return a message if the flight is not found. For example, if 100 was input, the output should be Flight #100 was not found.
If no flights were scheduled for the airport code entered, for example MCO, the message displayed should be Flight to MCO was not found.
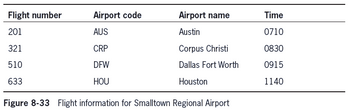

First, establish a structured repository to efficiently manage flight-related data. This repository should comprehensively catalog flight numbers, their associated airport codes, corresponding airport names, and the scheduled departure times for each flight.
Create a specialized function named get_flight_info. This function is tailored to receive a flight number as input and perform the following actions:
- Conduct an internal search within the data repository to ascertain if the specified flight number exists.
- In case of a successful match, retrieve and extract the relevant data elements, including the airport code, airport name, and the scheduled departure time.
- Present this information in an intelligible manner, encapsulating the flight number, airport code, airport name, and the designated departure time.
- Should no matching entry be identified, craft a response to communicate that the provided flight number does not correspond to any existing flight.
Complementing the previous function, create another specialized function called get_flight_info_by_code. This function is designed to accept an airport code as input and execute the following steps:
- Traverse the data repository systematically, seeking a flight associated with the provided airport code.
- Upon discovery of a matching flight, retrieve and format the pertinent details, which encompass the flight number, airport name, and the scheduled departure time.
- In scenarios where no corresponding flight data is found, generate a message to inform the user that no flights are scheduled to the specified airport code.
Instantiate an instance of the SmalltownRegionalAirport class. This instance will facilitate seamless access to the flight data within the system.
Enable user interaction by requesting input from the user, which can manifest as either a flight number or an airport code.
Deploy a systematic approach to interpret the user's input. Specifically, examine whether the provided input can be interpreted as an integer. The outcome of this examination determines whether the input represents a flight number or an airport code.
If the user input is conclusively identified as a flight number, initiate the get_flight_info function, supplying the flight number as an argument. Capture and retain the resultant data.
Conversely, if the user input aligns with an airport code, trigger the get_flight_info_by_code function with the airport code as input. Preserve the ensuing output for subsequent presentation.
Deliver the acquired result, which originates either from the execution of step 7 or step 8, in a user-friendly format. This presentation encapsulates exhaustive flight details or an apt notice if the entered input lacks a corresponding match in the data repository.
Conclude the algorithm, emphasizing its role in furnishing an efficient and user-centric mechanism for accessing flight information pertinent to Smalltown Regional Airport.
Step by stepSolved in 4 steps with 5 images

- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardIn this assignment you will demonstrate your knowledge of debugging by fixing the errors you find in the program below. Fix the code, and paste it in this document, along with the list of the problems you fixed.This example allows the user to display the string for the day of the week. For example, if the user passed the integer 1, the method will return the string Sunday. If the user passed the integer 2, the method will return Monday. This code has both syntax errors and logic errors. Hint: There are two logic errors to find and fix (in addition to the syntax errors).Inport daysAndDates.DaysOfWeek;public class TestDaysOfWeek {public static void main(String[] args) {System.out.println("Days Of week: ");for (int i = 0;i < 8;i++) {System.out.println("Number: " + i + "\tDay Of Week: " + DaysOfWeek.DayOfWeekStr(i) )}}}package daysAndDatespublic class DaysOfWeek {public static String DayOfWeekStr(int NumberOfDay) {String dayStr = ""switch (NumberOfDay) {case 1:dayStr =…arrow_forwardIn python Add the following four methods to your Crew class: move(self, location): This takes in a location as a string, along with self, and attempts to move the crew member to the specified location. If location is one of the five valid location options ("Bridge", "Medbay", "Engine", "Lasers", or "Sleep Pods"), then this should change self.location to that new value. Otherwise, the function should print out the message: Not a valid location. repair(self, ship): first_aid(self, ship): fire_lasers(self, ship, target_ship, target_location): The above three methods represent tasks that a basic Crew member is not capable of (but one of its derived classes will be able to accomplish). So each of them should simply print out a message of the form: <Name> doesn't know how to do that. Examples: Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. if __name__ == '__main__': crew1…arrow_forward
- Using C# create a console app doing the following Write a method that takes two integers and displays their sum. Write a method that takes five doubles and returns their average. Write a method that returns the sum of two randomly generated integers. Write a method that takes three integers and returns true if their sum is divisible by 3, false otherwise. Write a method that takes two strings and displays the string that has fewer characters. Write a method that takes an array of doubles and returns the largest value in the array. Write a method that generates and returns an array of fifty integer values. Write a method that takes two bool variables and returns true if they have the same value, false otherwise. Write a method that takes an int and a double and returns their product. Write a method that takes a two-dimensional array of integers and returns the average of the entries.arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardstrobogrammatic number is a number that looks the same when rotated 180 degrees (looked at upside down). Find all strobogrammatic numbers that are of length = n. For example, Given n = 2, return ["11","69","88","96"]. def gen_strobogrammatic(n): Given n, generate all strobogrammatic numbers of length n. :type n: int :rtype: List[str] return helper(n, n) def helper(n, length): if n == 0: return [™"] if n == 1: return ["1", "0", "8"] middles = helper(n-2, length) result = [] for middle in middles: if n != length: result.append("0" + middle + "0") result.append("8" + middle + "8") result.append("1" + middle + "1") result.append("9" + middle + "6") result.append("6" + middle + "9") return result def strobogrammatic_in_range(low, high): :type low: str :type high: str :rtype: int res = count = 0 low_len = len(low) high_len = len(high) for i in range(low_len, high_len + 1): res.extend(helper2(i, i)) for perm in res: if len(perm) == low_len and int(perm) int(high): continue count += 1 return…arrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT #2 so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #8. 8. Sum of Numbers in a String (page 610) Write a program that asks the user to enter a series of numbers separated by commas. Here are two examples of valid input: 7,9,10,2,18,6 8.777,10.9,11,2.2,-18,6 The program should calculate and display the sum of all the numbers. Input Validation 1. Make sure the string only contains numbers and commas (valid symbols are '0'-'9', '-', '.', and ','). 2. If the string is empty, a messages should be displayed, saying that the string is empty. Test Case 1 Enter a series of numbers separated only by commas or type QUIT to exit:\n7,9,10,2,18,6ENTERThe sum of those numbers is 52.00\nEnter a series of numbers separated only by commas or type QUIT to exit:\nENTEREmpty string.\nEnter a series of numbers separated only by…arrow_forwardProgram63.javaWrite a program that estimates the cost of carpet for one or more rooms with rectangular floors. Begin by prompting for the price of carpet per square yard and the number of rooms needing this carpet. Use a loop to prompt for the floor dimensions of each room in feet. In this loop, call a value-returning method with the dimensions and carpet price as arguments. The method should return the carpet cost for each room to main, where it will be printed and accumulated. After all rooms have been processed, the program should display the total cost of the job.Sample Output (image below) Program64.javaWrite a program that demonstrates method overloading by defining and calling methods that return the area of a triangle, a rectangle, or a square.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
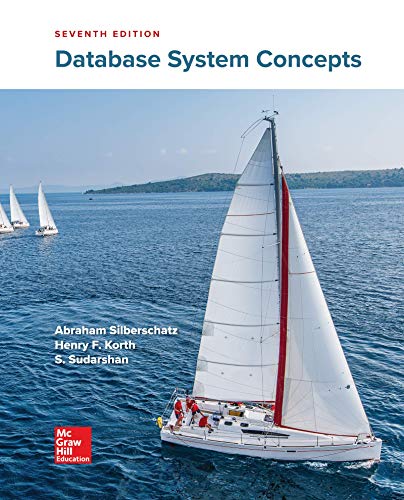
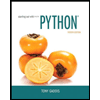
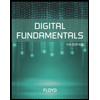
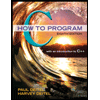
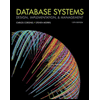
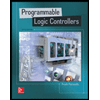