1. Problem Description: In this project, you will implement a simplified multi-cycle CPU using Verilog or systemverilog. The multi-cycle CPU should be able to perform arithmetic, memory, and control-related instructions. By completing this project, you will gain hands-on experience in building a fundamental component of modern computer systems. 2. Instruction Set Architecture Memory Instructions: 31-29 opcode 2.1. 2.2. 28-24 reg_addr_0 23-16 15-0 Addr Iw: Loads a 32-bit value from memory and writes it in register regFile[reg_addr_0] = memory[Addr] sw: Stored a 32-bit value from register file and writes it to memory memory(Addr] = regFile[reg_addr_0] Control Instructions: 31-29 opcode 28-24 reg_addr_0 23-19 18-16 14-0 reg_addr_1 Addr 2.3. 2.4. beq: Changes program counter (PC) when register values are equal if(regFile/reg_addr_0] == regFile[reg_addr_1]): PC = Addr blt: Changes PC when a register value is less than the other if(regFile/reg_addr_0] < regFile(reg_addr_1]): PC = Addr Arithmetic Instructions: 31-29 opcode 28-24 reg_addr_0 23-19 reg_addr 1 18-14 reg_addr_2 13-0 31-29 opcode 28-24 reg_addr_0 23-19 reg_addr_1 18-14 13-0 reg_addr_2 2.5. 2.6. 2.7. 2.8. add: Perform addition operation regFile[reg_addr_2] = regFile[reg_addr_0] + regFile[reg_addr_1] sub: Perform subtraction operation regFile[reg_addr_2] = regFile[reg_addr_0] - regFile[reg_addr_1] and: Perform bitwise AND operation regFile[reg_addr_2] = regFile[reg_addr_0] & regFile[reg_addr_1] or: Perform bitwise OR operation regFile[reg_addr_2] = regFile[reg_addr_0] | regFile[reg_addr_1] 3. CPU Components: Write a verilog module for each of the CPU components. Implement the decoder and ALU with combination logic. Implement the memory and register file using sequential logic. 3.1. 3.2. 3.3. 3.4. Memory (Instruction, Data): The memory module implements a 256x32 bits single read/write port RAM. The module takes in a 16-bit read address, 32-bit write data and a write enable signal as input. It also produces 32-bit read data as output. Register File: The register file implements a 16x32 bit dual read/single write port RAM. The module takes in two 16-bit read addresses, one 16-bit write address, and a write enable signal as input. It produces two 32-bit read data as output. Decoder: The decoder modules take the 32-bit instruction as an input and splits the instruction into its constituent signals based on the ISA. ALU: The ALU module performs arithmetic operations based on the opcode. It takes in two 32-bit input operands and produces a single 32-bit output. 4. CPU execution stages: Write a CPU top module instantiating all the CPU components. You will write a state machine that goes through each of the 4 stages of CPU execution. 4.1. 4.2. Fetch: During the fetch stage, a new instruction is read from the instruction memory and the program counter is incremented. Decode: In the decode stage, the instruction is decoded, and signals are established to assist its execution. At the same time, the register file is read to
1. Problem Description: In this project, you will implement a simplified multi-cycle CPU using Verilog or systemverilog. The multi-cycle CPU should be able to perform arithmetic, memory, and control-related instructions. By completing this project, you will gain hands-on experience in building a fundamental component of modern computer systems. 2. Instruction Set Architecture Memory Instructions: 31-29 opcode 2.1. 2.2. 28-24 reg_addr_0 23-16 15-0 Addr Iw: Loads a 32-bit value from memory and writes it in register regFile[reg_addr_0] = memory[Addr] sw: Stored a 32-bit value from register file and writes it to memory memory(Addr] = regFile[reg_addr_0] Control Instructions: 31-29 opcode 28-24 reg_addr_0 23-19 18-16 14-0 reg_addr_1 Addr 2.3. 2.4. beq: Changes program counter (PC) when register values are equal if(regFile/reg_addr_0] == regFile[reg_addr_1]): PC = Addr blt: Changes PC when a register value is less than the other if(regFile/reg_addr_0] < regFile(reg_addr_1]): PC = Addr Arithmetic Instructions: 31-29 opcode 28-24 reg_addr_0 23-19 reg_addr 1 18-14 reg_addr_2 13-0 31-29 opcode 28-24 reg_addr_0 23-19 reg_addr_1 18-14 13-0 reg_addr_2 2.5. 2.6. 2.7. 2.8. add: Perform addition operation regFile[reg_addr_2] = regFile[reg_addr_0] + regFile[reg_addr_1] sub: Perform subtraction operation regFile[reg_addr_2] = regFile[reg_addr_0] - regFile[reg_addr_1] and: Perform bitwise AND operation regFile[reg_addr_2] = regFile[reg_addr_0] & regFile[reg_addr_1] or: Perform bitwise OR operation regFile[reg_addr_2] = regFile[reg_addr_0] | regFile[reg_addr_1] 3. CPU Components: Write a verilog module for each of the CPU components. Implement the decoder and ALU with combination logic. Implement the memory and register file using sequential logic. 3.1. 3.2. 3.3. 3.4. Memory (Instruction, Data): The memory module implements a 256x32 bits single read/write port RAM. The module takes in a 16-bit read address, 32-bit write data and a write enable signal as input. It also produces 32-bit read data as output. Register File: The register file implements a 16x32 bit dual read/single write port RAM. The module takes in two 16-bit read addresses, one 16-bit write address, and a write enable signal as input. It produces two 32-bit read data as output. Decoder: The decoder modules take the 32-bit instruction as an input and splits the instruction into its constituent signals based on the ISA. ALU: The ALU module performs arithmetic operations based on the opcode. It takes in two 32-bit input operands and produces a single 32-bit output. 4. CPU execution stages: Write a CPU top module instantiating all the CPU components. You will write a state machine that goes through each of the 4 stages of CPU execution. 4.1. 4.2. Fetch: During the fetch stage, a new instruction is read from the instruction memory and the program counter is incremented. Decode: In the decode stage, the instruction is decoded, and signals are established to assist its execution. At the same time, the register file is read to
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Asap please
![1. Problem Description:
In this project, you will implement a simplified multi-cycle CPU using Verilog or
systemverilog. The multi-cycle CPU should be able to perform arithmetic, memory, and
control-related instructions. By completing this project, you will gain hands-on
experience in building a fundamental component of modern computer systems.
2. Instruction Set Architecture
Memory Instructions:
31-29
opcode
2.1.
2.2.
28-24
reg_addr_0
23-16
15-0
Addr
Iw: Loads a 32-bit value from memory and writes it in register
regFile[reg_addr_0] = memory[Addr]
sw: Stored a 32-bit value from register file and writes it to memory
memory(Addr] = regFile[reg_addr_0]
Control Instructions:
31-29
opcode
28-24
reg_addr_0
23-19
18-16
14-0
reg_addr_1
Addr
2.3.
2.4.
beq: Changes program counter (PC) when register values are equal
if(regFile/reg_addr_0] == regFile[reg_addr_1]):
PC = Addr
blt: Changes PC when a register value is less than the other
if(regFile/reg_addr_0] < regFile(reg_addr_1]):
PC = Addr
Arithmetic Instructions:
31-29
opcode
28-24
reg_addr_0
23-19
reg_addr 1
18-14
reg_addr_2
13-0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0a997a6f-a222-4716-adb9-ab223c542cab%2Fba6d920a-593b-4772-aec8-e1634cee362d%2Feuzhu1_processed.jpeg&w=3840&q=75)
Transcribed Image Text:1. Problem Description:
In this project, you will implement a simplified multi-cycle CPU using Verilog or
systemverilog. The multi-cycle CPU should be able to perform arithmetic, memory, and
control-related instructions. By completing this project, you will gain hands-on
experience in building a fundamental component of modern computer systems.
2. Instruction Set Architecture
Memory Instructions:
31-29
opcode
2.1.
2.2.
28-24
reg_addr_0
23-16
15-0
Addr
Iw: Loads a 32-bit value from memory and writes it in register
regFile[reg_addr_0] = memory[Addr]
sw: Stored a 32-bit value from register file and writes it to memory
memory(Addr] = regFile[reg_addr_0]
Control Instructions:
31-29
opcode
28-24
reg_addr_0
23-19
18-16
14-0
reg_addr_1
Addr
2.3.
2.4.
beq: Changes program counter (PC) when register values are equal
if(regFile/reg_addr_0] == regFile[reg_addr_1]):
PC = Addr
blt: Changes PC when a register value is less than the other
if(regFile/reg_addr_0] < regFile(reg_addr_1]):
PC = Addr
Arithmetic Instructions:
31-29
opcode
28-24
reg_addr_0
23-19
reg_addr 1
18-14
reg_addr_2
13-0
![31-29
opcode
28-24
reg_addr_0
23-19
reg_addr_1
18-14
13-0
reg_addr_2
2.5.
2.6.
2.7.
2.8.
add: Perform addition operation
regFile[reg_addr_2] = regFile[reg_addr_0] + regFile[reg_addr_1]
sub: Perform subtraction operation
regFile[reg_addr_2] = regFile[reg_addr_0] - regFile[reg_addr_1]
and: Perform bitwise AND operation
regFile[reg_addr_2] = regFile[reg_addr_0] & regFile[reg_addr_1]
or: Perform bitwise OR operation
regFile[reg_addr_2] = regFile[reg_addr_0] | regFile[reg_addr_1]
3. CPU Components:
Write a verilog module for each of the CPU components. Implement the decoder and
ALU with combination logic. Implement the memory and register file using sequential
logic.
3.1.
3.2.
3.3.
3.4.
Memory (Instruction, Data):
The memory module implements a 256x32 bits single read/write port RAM. The
module takes in a 16-bit read address, 32-bit write data and a write enable signal
as input. It also produces 32-bit read data as output.
Register File:
The register file implements a 16x32 bit dual read/single write port RAM. The
module takes in two 16-bit read addresses, one 16-bit write address, and a write
enable signal as input. It produces two 32-bit read data as output.
Decoder:
The decoder modules take the 32-bit instruction as an input and splits the
instruction into its constituent signals based on the ISA.
ALU:
The ALU module performs arithmetic operations based on the opcode. It takes in
two 32-bit input operands and produces a single 32-bit output.
4. CPU execution stages:
Write a CPU top module instantiating all the CPU components. You will write a state
machine that goes through each of the 4 stages of CPU execution.
4.1.
4.2.
Fetch: During the fetch stage, a new instruction is read from the
instruction memory and the program counter is incremented.
Decode: In the decode stage, the instruction is decoded, and signals are
established to assist its execution. At the same time, the register file is read to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0a997a6f-a222-4716-adb9-ab223c542cab%2Fba6d920a-593b-4772-aec8-e1634cee362d%2Fxd8fadg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:31-29
opcode
28-24
reg_addr_0
23-19
reg_addr_1
18-14
13-0
reg_addr_2
2.5.
2.6.
2.7.
2.8.
add: Perform addition operation
regFile[reg_addr_2] = regFile[reg_addr_0] + regFile[reg_addr_1]
sub: Perform subtraction operation
regFile[reg_addr_2] = regFile[reg_addr_0] - regFile[reg_addr_1]
and: Perform bitwise AND operation
regFile[reg_addr_2] = regFile[reg_addr_0] & regFile[reg_addr_1]
or: Perform bitwise OR operation
regFile[reg_addr_2] = regFile[reg_addr_0] | regFile[reg_addr_1]
3. CPU Components:
Write a verilog module for each of the CPU components. Implement the decoder and
ALU with combination logic. Implement the memory and register file using sequential
logic.
3.1.
3.2.
3.3.
3.4.
Memory (Instruction, Data):
The memory module implements a 256x32 bits single read/write port RAM. The
module takes in a 16-bit read address, 32-bit write data and a write enable signal
as input. It also produces 32-bit read data as output.
Register File:
The register file implements a 16x32 bit dual read/single write port RAM. The
module takes in two 16-bit read addresses, one 16-bit write address, and a write
enable signal as input. It produces two 32-bit read data as output.
Decoder:
The decoder modules take the 32-bit instruction as an input and splits the
instruction into its constituent signals based on the ISA.
ALU:
The ALU module performs arithmetic operations based on the opcode. It takes in
two 32-bit input operands and produces a single 32-bit output.
4. CPU execution stages:
Write a CPU top module instantiating all the CPU components. You will write a state
machine that goes through each of the 4 stages of CPU execution.
4.1.
4.2.
Fetch: During the fetch stage, a new instruction is read from the
instruction memory and the program counter is incremented.
Decode: In the decode stage, the instruction is decoded, and signals are
established to assist its execution. At the same time, the register file is read to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
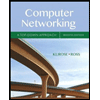
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
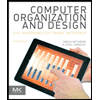
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
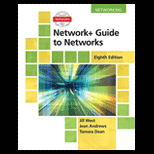
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
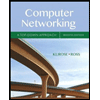
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
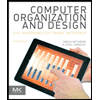
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
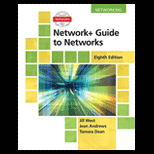
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
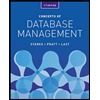
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
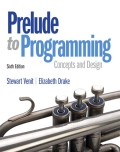
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
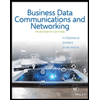
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY