1. One Python function per room. 2. It should include the all the print out of the room description as well as the code for the basic command processing. 3. The function should not take any parameters. 4. Modify the command processing so that until we go to another room, we continue to ask the use for what they want to do in the room. 5. When we exit the room the function should return the room we are going to to the main program. 6. Make sure there is only a single return in your function. We also want to start creating our main program. This should be a loop until the game ends. For now we can make it an infinite loop. Here are the requirements of the main program. 1. It should initialize a variable to the starting room number. 2. Start a loop that runs until game end. For now it can be an infinite loop. 3. In the loop call the appropriate room function based on the room we are in. Remember that the room function will return the value of the room we move to.
1. One Python function per room. 2. It should include the all the print out of the room description as well as the code for the basic command processing. 3. The function should not take any parameters. 4. Modify the command processing so that until we go to another room, we continue to ask the use for what they want to do in the room. 5. When we exit the room the function should return the room we are going to to the main program. 6. Make sure there is only a single return in your function. We also want to start creating our main program. This should be a loop until the game ends. For now we can make it an infinite loop. Here are the requirements of the main program. 1. It should initialize a variable to the starting room number. 2. Start a loop that runs until game end. For now it can be an infinite loop. 3. In the loop call the appropriate room function based on the room we are in. Remember that the room function will return the value of the room we move to.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
1. One Python function per room.
2. It should include the all the print out of the room description as well as the code for the basic command processing.
3. The function should not take any parameters.
4. Modify the command processing so that until we go to another room, we continue to ask the use for what they want to do in the room.
5. When we exit the room the function should return the room we are going to to the main program.
6. Make sure there is only a single return in your function.
We also want to start creating our main program. This should be a loop until the game ends. For now we can make it an infinite loop. Here are the requirements of the main program.
1. It should initialize a variable to the starting room number.
2. Start a loop that runs until game end. For now it can be an infinite loop.
3. In the loop call the appropriate room function based on the room we are in. Remember that the room function will return the value of the room we move to.

Transcribed Image Text:def townSquare():
print("You're in Town Square.")
print("Type 'Northeast' or 'NE' to go to Town Hall")
print("Type 'South' or 'S' to go to the Town Archives")
print("type 'Southeast' or 'SE' to go to the Trading Post")
userInput = input("Player choice: ")
="Northeast":
if userInput:
townHall()
if userInput =="NE":
town Hall (
elif userInput: ="South":
townArchives()
elif userInput == "S":
townArchives()
elif userInput == "Southeast":
tradingPost
elif userInput == "SE":
tradingPost
else:
print('Command unrecognize')
townSquare() #whenever invaild code is put in, the user gets to try again.
#programing the trading post
def tradingPost():
print("Welcome! You're in the Trading Post,") #printing out the options
print("You found a key.")
print("type 'Northwest' or 'NW' to go to Car Ride.")
userInput = input("Player choice: ") #creating user input
if userInput: =="Northwest":
townSquare() #destination
if userInput: ="NW":
townSquare #destination
else:
print(Command unrecognize')
tradingPost()
#programing the Secret Room
def secretRoom():
print("Welcome! You're in the Secret Room.") #printing out the options
print("Go to the Study and type Pull Book.")
print("type 'North' or 'N' to go to the Study.")
userInput = input("Player choice: ") #creating user input
if userInput =="North":
study #destination
if userInput =="N":
==
study #destination
else:
print(Command unrecognize')
secretRoom()
#programing the town archives
def townArchives():
print("Welcome! You're in the Town Archives.") #printing out the options
print("type 'South' or 'S' to go to Study.")
userInput = input("Player choice: ") #creating user input
if userInput: ="South":
study) #destination
if userInput:
else:
study) #destination
print(Command unrecognize')
townArchives()
#programing the garden
def garden():
print("Welcome! You're in the Garden,") #printing out the options
print("type 'West' or 'W' to go to the Dead End and return to Car Ride or 'Southeast' or 'SE' to return to Town
Square")
userInput = input("Player choice: ") #creating user input
if userInput =="West":
carRide #destination
if userInput =="W":
carRide() #destination
elif userInput:
else:
="S":
townSquare)
elif userInput == "SE":
townSquare)
garden()
print(Command unrecognize')
else:
#programing the end room
def endRoom():
print("Winner!") #the player wins
#programing the cult chambers
def cultChambers():
print("Welcome! You're in the Cult Chambers,") #printing out the options
print("type 'East' or 'E' to go to the Dead End or 'South' or 'S' to End Game.")
userInput = input("Player choice: ")
if userInput: ="East":
#creating user input
print("Dead End")
if userInput:
="E":
print("Dead End")
elif userInput
=="South":
endRoom() #destination
elif userInput == "S":
endRoom()
else:
=="Southeast":
print(Command unrecognize')
cultChambers
#programing the under ground cave
def underGroundCave():
print("Welcome! You're in the Under Ground Cave,")
print("type 'South' or 'S' to go to the Cult Chambers") #printing out the options
userInput = input("Player choice: ") #creating user input
if userInput == "South":
cultChambers #destination
if userInput == "S":
cultChambers
else:
#adding abbreviations throughout the code.
print(Command unrecognize')
underGroundCave()
#programing the study
def study:
print("Welcome! You're in the Study,")
print("type 'Pull Book' or 'PB' to go to the Underground Cave") #printing out the options
userInput = input("Player choice: ") #creating user input
if userInput:
="Pull Book":
underGroundCave() #destination
if userInput =="PB":
underGroundCave #destination
carRide #destination
else:
#programing the mansion
def mansion():
print("Welcome! You're in Mansion,")
print("type 'West' or 'W' to go to the Garden") #printing out the options
print("type 'North' or 'N' to go to the Study")
print("type 'Key' or 'K' to enter the Secret Room.")
userInput = input("Player choice: ") #creating user input
if userInput =="West":
garden()
if userInput:
garden()
elif userInput:
="North":
#destination
study)
elif userInput: :"N":
study) #destination
elif userInput =="Key":
secretRoom0 #destination
elif userInput =="K":
secretRoom() #destination
else:
="W":
==
#destination
print('Command unrecognize')
mansion()
#programing the town hall
def town Hall ():
print("Welcome! You're in the Town Hall,") #printing out the options
print("type 'West' or 'W' to go to the Mansion.")
userInput
#destination
if userInput:
mansion()
if userInput =="W":#destination
mansion()
= input("Player choice: ") #creating user input
=="West":#destination
else:
print('Command unrecognize')
town Hall (
#programing the car ride
print("You're in a car. The town of Loveheaven is ahead. Can
you escape?")
def carRide():
print("Type 'West' or 'W' to go to Town Square else South/S, North/N/ or West/W for random location.")
userInput = input("Player choice:") #creating user input
=="West": #destination
if userInput:
townSquare)
if userInput =="W":
townSquare)
if userInput =="South":
tradingPost
carRide()
if userInput == "S":
tradingPost()
if userInput =="North":
garden()
if userInput: ="N":
garden()
if userInput =="West":
underGroundCave()
if userInput =="W":
underGroundCave()
print(Command unrecognize')
carRide #calling for the function
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
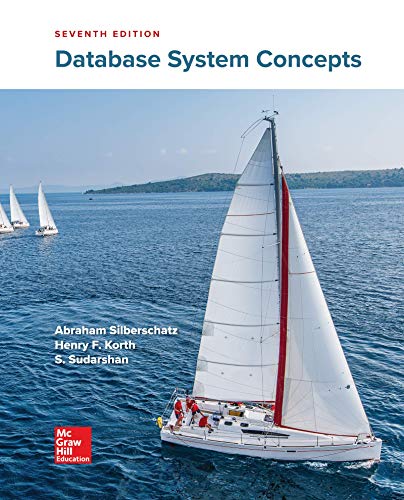
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
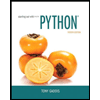
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
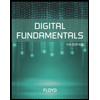
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
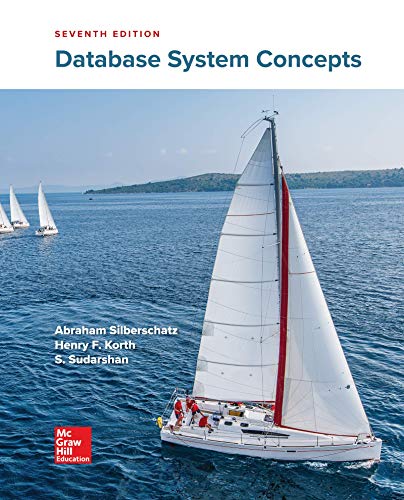
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
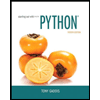
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
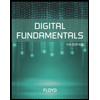
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
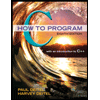
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
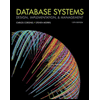
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
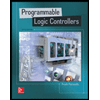
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education