1. Create a GUI that has two check boxes which allow a user to select between a car and a truck and also create a GUI that pops up ONLY if the car is selected.
1. Create a GUI that has two check boxes which allow a user to select between a car and a truck and also create a GUI that pops up ONLY if the car is selected. This new window should contain JLabels with JTextFields that allow the user to enter in all the car's relevant information - e.g. Make, Model, Year, Number of Doors, Fuel Tank Capacity, and Driving Range. Use the same inputs from Main.java in the Appendix. The new window should also include a calculate MPG button which computes and outputs the miles per gallon when pressed. In this case, the MPG is 24.0.
Using the appendix code below:
Appendix
// Traits.java
public interface Traits{
public String getMake();
public String getModel();
}
// Vehicle.java
public class Vehicle implements Traits{
private String make;
private String model;
private int year;
private int number_of_doors;
private double fuel_tank_capacity;
private int driving_range;
public Vehicle(String vehicle_make, String vehicle_model, int vehicle_year, int
vehicle_number_of_doors, double fuel_capacity, int range){
make = vehicle_make;
model = vehicle_model;
year = vehicle_year;
number_of_doors = vehicle_number_of_doors;
fuel_tank_capacity = fuel_capacity;
driving_range = range;
}
//get methods
public String getMake(){
return make;
}
public String getModel(){
return model;
}
public int getYear(){
return year;
}
public int getNumberOfDoors(){
return number_of_doors;
}
public double getFuelCapacity (){
return fuel_tank_capacity;
}
public int getRange (){
return driving_range;
}
public double calcMPG(){
return driving_range / fuel_tank_capacity;
}
}
// Truck.java
public class Truck extends Vehicle{
private int towing_capacity;
private int payload_capacity;
public Truck (String make, String model, int year, int number_of_doors, double
fuel_capacity, int range, int towing, int payload){
super(make, model, year, number_of_doors, fuel_capacity, range);
towing_capacity = towing;
payload_capacity = payload;
}
public int getTowingCapacity(){
return towing_capacity;
}
public int getPayloadCapacity(){
return payload_capacity;
}
}
// Main.java
class Main {
public static void main(String[] args) {
Vehicle car = new Vehicle("Ford","Mustang",2022,2,15.5,372);
Truck truck = new Truck("Ford","F150",2022,4,23,483,8200,2629);
System.out.println("Make: " + car.getMake());
System.out.println("Model: " + car.getModel());
System.out.println("Year: " + car.getYear());
System.out.println("Number of Doors: " + car.getNumberOfDoors());
System.out.println("Fuel Tank Capacity: " + car.getFuelCapacity());
System.out.println("Driving Range: " + car.getRange());
System.out.println("MPG: " + car.calcMPG());
System.out.println("\n");
System.out.println("Make: " + truck.getMake());
System.out.println("Model: " + truck.getModel());
System.out.println("Year: " + truck.getYear());
System.out.println("Number of Doors: " + truck.getNumberOfDoors());
System.out.println("Fuel Tank Capacity: " + truck.getFuelCapacity());
System.out.println("Driving Range: " + truck.getRange());
System.out.println("Towing Capacity: " + truck.getTowingCapacity());
System.out.println("Payload Capacity: " + truck.getPayloadCapacity());
System.out.println("MPG: " + truck.calcMPG());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

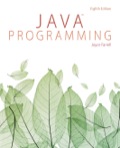
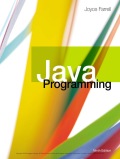
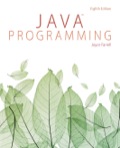
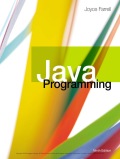