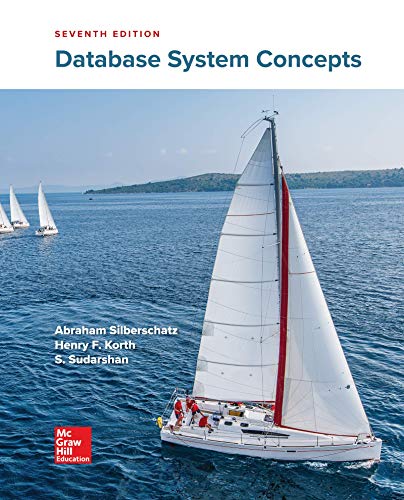
Concept explainers
1) Through BlueJ, the class must define a method called isSpace that allows the book club staff to determine whether there is enough capacity for a group to attend. This method must take a single integer parameter representing the size of the group, and return a boolean result. The method must work as follows: If the value of the parameter is less-than or equal-to 0 then the method must return false. This case has priority over those following. If the value of the parameter is less-than or equal-to the space left in the book club (use the capacity and occupancy values in the to work this out) then the method must return true. Otherwise (i.e., if there is not space in the book club for the whole group) then the method must return false.
2) This method must not change the state of the BookClub object. In other words, both the current number of occupants and the capacity of the club must be exactly the same after it is called as it was before (Note that the return type of this method must be boolean. The method has to return either true or false and that does not mean that it should print or return the strings "true" or "false)
3) The class must define a method called printDetails that prints the current details (using System.out.println) of the book club in the following format: The Ven: 50 shoppers. Capacity 150. Where "The Ven" is the book club's name; 50 shoppers are currently present and the club’s capacity is 150. Of course, different BookClub objects will have different names, number of clubbers and different capacities. (Take note of the spacing between the different parts, the punctuation, and the order of the things to be printed) Write a method called about that has a void return type and takes no parameter. When called, this must print: BookClub written by login where you must replace login with for instance: BookClub written by djb247

- Start.
- Create a class named
BookClub
with private instance variablesname
,capacity
, andoccupancy
. - Define a constructor for
BookClub
that takesname
andcapacity
as parameters and initializes thename
,capacity
, andoccupancy
. - Create a method
isSpace
that checks if there is space for a group of a given size. If the group size is non-positive, it returnsfalse
. Otherwise, it calculates the space left in the book club and returnstrue
if the group can fit, orfalse
if there's not enough space. - Define a
printDetails
method that prints the name of the book club, the current occupancy, and the capacity. - Implement an
about
method that prints a message about the book club's author (assuming "The Ven" is the author). - In the
main
method:- Create an instance of the
BookClub
class with the name "The Ven" and a capacity of 150. - Check if there's space for a group of size 50 and print the result.
- Check if there's space for a group of size -10 and print the result.
- Print the details of the book club.
- Print information about the author.
- Create an instance of the
- End.
Step by stepSolved in 5 steps with 3 images

- In python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forwardWhich of the following statements is false? a. A class can contain only one constructor. b. An example of a behavior is the SetTime method in a Time class. c. An object created from a class is referred to as an instance of the class. d. An instance of a class is considered an object.arrow_forward1) Write a class on BlueJ, which must be called BookClub. The class must have exactly three fields: two integer fields called capacity and occupancy and one String field called name. The capacity field will store the maximum number of shoppers allowed and occupancy will store the current number of shoppers. (There must be no additional fields defined in the class, even if you think more are required. Consider using local variables inside methods if you think you need extra fields.) 2) The initial values of the book club name, and capacity must be received as parameters to the class's constructor, while the occupancy must always be set to zero when a BookClub object is created. 3) The class must define getter methods for all of the fields. These must be called getName, getCapacity and getOccupancy. You must not call them anything different. You must not write setter methods for the fields. The class must define a method called addOne that takes no parameters. If the current number of…arrow_forward
- The class provided represents a item. Information about a item includes the name of the item and the count of items in inventory (inventory count). When ordering items they are ordered in descending order by the inventory count. If items have the same inventory count then they are ordered in ascending alphabetical order by their name (a-z). Here is an example set of items: 7 Camera 5 Bicycle 5 Lego 5 Robot 3 Tricycle The Item class is missing a required method. Write the missing method in the space provided below. public class Item implements Comparable { private String name; private int count; public Item (String nameIn, int count In) { name name In; count countIn; }arrow_forward2please write in C#arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
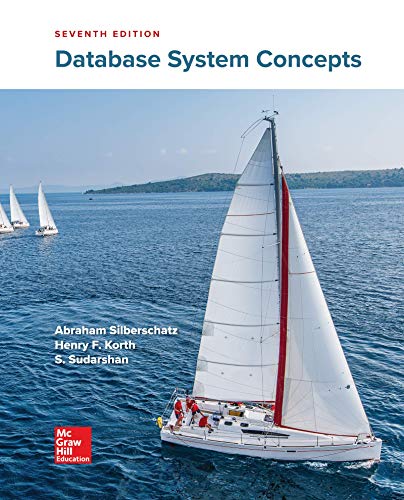
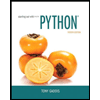
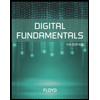
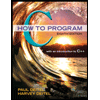
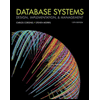
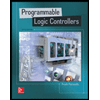