.c, which implements the functions in the arrayOptn.h You are already provided with the main() function. Do not edit anything there in the main.c file.
Array Processing.
In this problem, you have two tasks:
- To create the header file, arrayOptn.h, and add the function declarations of the following functions listed below.
- To create the file, arrayOptn.c, which implements the functions in the arrayOptn.h
You are already provided with the main() function. Do not edit anything there in the main.c file.
-
-
-
List of functions to be implemented:
-
1 - void createArray(int arr[], int count, int maxCapacity)
-
Parameters:
- int arr[] - a reference to an array
- int count - the number of elements to be inputted by the user and to be stored in the array
- int maxCapacity - the max capacity of the array
-
Description:
- Asks the user for the elements of the array. The prompt message is: "Enter element at index N: " where N is the current index position being scanned.
- Each inputted integer must be unique. If an element already exists in the array, you prompt the user the message "Inputted element already exists, try another number"
- If the count is less than the maxCapacity, set the values of all the remaining indices of the array to 0.
-
-
2 - void printArray(int arr[], int count)
-
Parameters:
- int arr[] - a reference to the array whose elements are to be printed
- int count - the number of elements in the array
-
Description:
- Prints all the elements of the array starting from index 0 up to index count - 1
- The format of the message is: "Elements: el1 el2 el3 elx" where e1, el2, el3, elx are the current elements of the array.
-
-
3 - int locateIndex(int arr[], int count, int elem)
-
Parameters:
- int arr[] - a reference to the array where we will search the element
- int count - the number of elements in the array
- int elem - the element to be searched
-
Description:
- Finds the index of the element in the array
-
Return Value:
- If the element is found, this returns the index of the element. Otherwise, this returns -1.
-
-
4 - int locateElement(int arr[], int count, int elem)
-
Parameters:
- int arr[] - a reference to the array where we will search the element
- int count - the number of elements in the array
- int elem - the element to be searched
-
Description:
- Checks if the element is in the array
-
Return Value:
- If the element is found, this returns 1 to represent true. Otherwise, this returns 0 to represent false.
-
-
-
-
main.c:
#include<stdio.h>
#include "arrayOptn.h"
#define MAX_SIZE 100
int main(void) {
int array[MAX_SIZE];
int count = 0;
int numberOfCommands;
printf("Enter number of commands: ");
scanf("%d", &numberOfCommands);
printf("Enter count of elements: ");
scanf("%d", &count);
printf("\n");
int command;
int elem, k;
for(int c = 1; c <= numberOfCommands; c++) {
printf("COMMAND #: ");
scanf("%d", &command);
switch(command) {
case 1:
createArray(array, count, MAX_SIZE);
printf("\n");
break;
case 2:
printArray(array, count);
printf("\n\n");
break;
case 3:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", locateIndex(array, count, elem));
printf("\n\n");
break;
case 4:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", locateElement(array, count, elem));
printf("\n\n");
break;
case 5:
printElementsInAscending(array, count);
printf("\n\n");
break;
case 6:
printElementsInDescending(array, count);
printf("\n\n");
break;
case 7:
printf("Enter element: ");
scanf("%d", &elem);
printf("Enter position: ");
scanf("%d", &k);
printf("Return Value: %d", insertAtPos(array, &count, elem, k));
printf("\n\n");
break;
case 8:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", insertFront(array, &count, elem));
printf("\n\n");
break;
case 9:
printf("Enter position: ");
scanf("%d", &k);
printf("Return Value: %d", removeAtPos(array, &count, k));
printf("\n\n");
break;
case 10:
printf("Enter element: ");
scanf("%d", &elem);
printf("Return Value: %d", removeElement(array, &count, elem));
printf("\n\n");
break;
case 11:
printf("Return Value: %d", removeFront(array, &count));
printf("\n\n");
}
}
return 0;
}

Step by step
Solved in 5 steps with 1 images

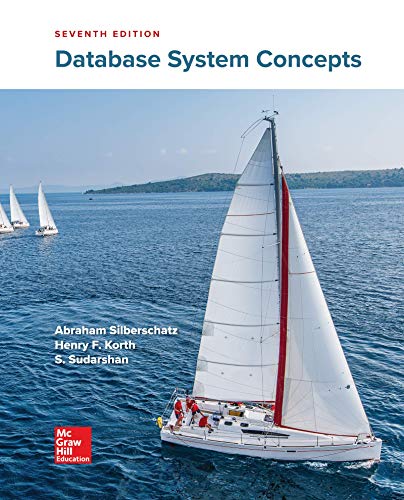
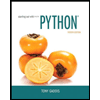
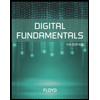
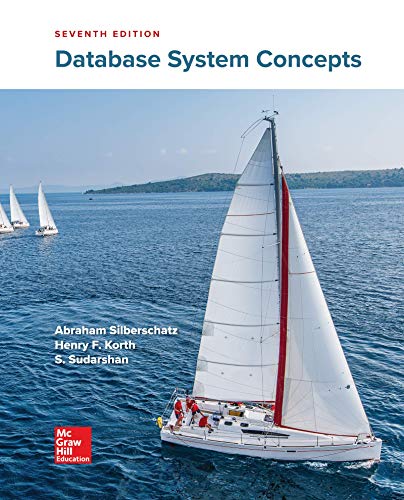
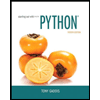
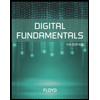
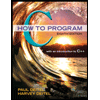
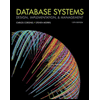
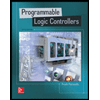