Reference_Notebook_Milestone_1_Classification+FINAL
html
keyboard_arrow_up
School
Rutgers University *
*We aren’t endorsed by this school
Course
700
Subject
Economics
Date
Apr 30, 2024
Type
html
Pages
37
Uploaded by DeanQuetzalPerson1017
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
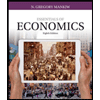
Essentials of Economics (MindTap Course List)
Economics
ISBN:9781337091992
Author:N. Gregory Mankiw
Publisher:Cengage Learning
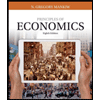
Principles of Economics (MindTap Course List)
Economics
ISBN:9781305585126
Author:N. Gregory Mankiw
Publisher:Cengage Learning
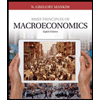
Brief Principles of Macroeconomics (MindTap Cours...
Economics
ISBN:9781337091985
Author:N. Gregory Mankiw
Publisher:Cengage Learning

Principles of Economics, 7th Edition (MindTap Cou...
Economics
ISBN:9781285165875
Author:N. Gregory Mankiw
Publisher:Cengage Learning
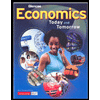
Economics Today and Tomorrow, Student Edition
Economics
ISBN:9780078747663
Author:McGraw-Hill
Publisher:Glencoe/McGraw-Hill School Pub Co
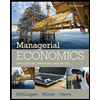
Managerial Economics: Applications, Strategies an...
Economics
ISBN:9781305506381
Author:James R. McGuigan, R. Charles Moyer, Frederick H.deB. Harris
Publisher:Cengage Learning
Recommended textbooks for you
- Essentials of Economics (MindTap Course List)EconomicsISBN:9781337091992Author:N. Gregory MankiwPublisher:Cengage LearningPrinciples of Economics (MindTap Course List)EconomicsISBN:9781305585126Author:N. Gregory MankiwPublisher:Cengage LearningBrief Principles of Macroeconomics (MindTap Cours...EconomicsISBN:9781337091985Author:N. Gregory MankiwPublisher:Cengage Learning
- Principles of Economics, 7th Edition (MindTap Cou...EconomicsISBN:9781285165875Author:N. Gregory MankiwPublisher:Cengage LearningEconomics Today and Tomorrow, Student EditionEconomicsISBN:9780078747663Author:McGraw-HillPublisher:Glencoe/McGraw-Hill School Pub CoManagerial Economics: Applications, Strategies an...EconomicsISBN:9781305506381Author:James R. McGuigan, R. Charles Moyer, Frederick H.deB. HarrisPublisher:Cengage Learning
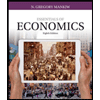
Essentials of Economics (MindTap Course List)
Economics
ISBN:9781337091992
Author:N. Gregory Mankiw
Publisher:Cengage Learning
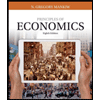
Principles of Economics (MindTap Course List)
Economics
ISBN:9781305585126
Author:N. Gregory Mankiw
Publisher:Cengage Learning
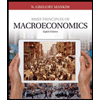
Brief Principles of Macroeconomics (MindTap Cours...
Economics
ISBN:9781337091985
Author:N. Gregory Mankiw
Publisher:Cengage Learning

Principles of Economics, 7th Edition (MindTap Cou...
Economics
ISBN:9781285165875
Author:N. Gregory Mankiw
Publisher:Cengage Learning
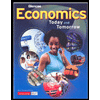
Economics Today and Tomorrow, Student Edition
Economics
ISBN:9780078747663
Author:McGraw-Hill
Publisher:Glencoe/McGraw-Hill School Pub Co
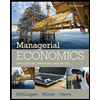
Managerial Economics: Applications, Strategies an...
Economics
ISBN:9781305506381
Author:James R. McGuigan, R. Charles Moyer, Frederick H.deB. Harris
Publisher:Cengage Learning