CPSC_1160_Practice_Final_Exam
.docx
keyboard_arrow_up
School
Langara College *
*We aren’t endorsed by this school
Course
1160
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
2
Uploaded by BailiffWildcat2224
CPSC 1160 Algorithms and Data Structures I: Practice Final Exam
Part 1: Multiple Choice Questions
1.
Algorithm Complexity: What is the time complexity of a binary search algorithm in the worst case?
A) O(n)
B) O(log n)
C) O(n log n)
D) O(n^2)
2.
Data Structures: Which data structure uses LIFO (Last In, First Out) principle?
A) Queue
B) Stack
C) Linked List
D) Array
3.
C++ Syntax: Which of the following is the correct way to declare a dynamic array in C++?
A) int* arr = new int[10];
B) int arr[10];
C) int* arr = int[10];
D) int arr = new int[10];
Part 2: Short Answer Questions
4.
Recursion: Write a recursive function in C++ that calculates the factorial of a given number.
5.
Algorithm Analysis: Explain the difference between time complexity and space complexity in algorithms.
Part 3: Coding Problems
6.
Sorting Algorithm: Implement the Merge Sort algorithm in C++.
7.
Data Structure Implementation: Write a C++ program to implement a singly linked list with functions to add and delete nodes.
8.
Advanced Coding Problem: Write a C++ program that uses a recursive function to find all the combinations of a given string.
Part 4: Theory Questions
9.
Q1: Explain the concept of a binary tree and its applications.
10. Q2: Describe the process of memory management in C++ and the role of constructors and destructors.
Instructions for the Exam
Allocate time wisely to each section of the exam.
For coding problems, ensure that your code compiles and runs as expected.
Provide clear and concise answers for theory and short answer questions.
Check your work for errors before submitting the exam.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
In data structures and algorithms,what is the disadvantage in using a circular linked list?
arrow_forward
... ...
Multiple choice:
Which data structure can be used to code a fast and efficient Dijkstra’s algorithm implementation?
Select all that applies (you may choose many):
a. Queue
b. Binary Search Tree
c. Priority Queue
d. Stack
arrow_forward
Examples include the pros and cons of sequential and binary search approaches.
arrow_forward
General computer science question
arrow_forward
convert it to java code
arrow_forward
Q2 please show your work
arrow_forward
Answer with True or False
Linked List is a nonlinear data
structure
Select one:
O
True
O False
arrow_forward
Computer science question 1
arrow_forward
Show step by step approach to evaluate the following prefix expression by applying an
algorithm using “Queue".
(ii)
** 8 9 - 5 8
arrow_forward
As compared to alternative data structures like a linked list or an array, the advantages of a binary search tree become immediately obvious.
arrow_forward
Data structure
arrow_forward
1. Imagine there are 50 nodes in a queue and the head and the tail are pointing to the one and the same node. What is this type of queue called?
2. Can a binary search algorithm be written by recursion?
arrow_forward
The best data structures in terms of asymptotic time complexities for performing the following operations respectively are
CONCATENATION (A, B): Concatenate all elements of A and B together
Insert(T, key): Insert key to T
Delete (T, key): Delete key from T if present
Search (T, key): Returns true if key is present in T
Stack, Binary Search Tree, Sorted Array, Linked List
Linked List, Stack, Binary Search Tree, Sorted Array
Linked List, Stack, Sorted Array, Binary Search Tree
Stack, Linked List, Sorted Array, Binary Search Tree
arrow_forward
Determine the time complexity of the following algorithms:
arrow_forward
Depth-First Search is implemented in recursion with FILO data structure.
Select one:
True
False
arrow_forward
Circular Lists
A linear list is being maintained circularly in an array c[0…n-1] with front, and rear set up as for circular queues.
a)Calculate the time complexity of your algorithms for b) and c).
b)Write an algorithm to delete the k-th element in the list.
c)Write an algorithm to insert an element y immediately after the k-th element.
arrow_forward
General Computer science questions
arrow_forward
Determine the time complexity of the following algorithms:
arrow_forward
What data structure will you most likely see in a
non-recursive implementation of a recursive
algorithm?
A A stack .
B An array
C A record
D Queue
arrow_forward
Q 10
Please explain your answers
arrow_forward
When compared to alternative data structures, such as a linked
list or an array, the advantages of a binary search tree are clear.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
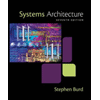
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Related Questions
- In data structures and algorithms,what is the disadvantage in using a circular linked list?arrow_forward... ... Multiple choice: Which data structure can be used to code a fast and efficient Dijkstra’s algorithm implementation? Select all that applies (you may choose many): a. Queue b. Binary Search Tree c. Priority Queue d. Stackarrow_forwardExamples include the pros and cons of sequential and binary search approaches.arrow_forward
- As compared to alternative data structures like a linked list or an array, the advantages of a binary search tree become immediately obvious.arrow_forwardData structurearrow_forward1. Imagine there are 50 nodes in a queue and the head and the tail are pointing to the one and the same node. What is this type of queue called? 2. Can a binary search algorithm be written by recursion?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
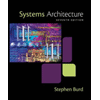
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning