Consider the algorithm below, which sorts a list of n elements by using a Priority Queue (the algorithm does not actually sort the list; rather just displays the sorted contents of the list.) public static void sort(List ts) { PriorityQueue pq = new PriorityQueue(); for (Integer t1 : ts) pq. insert(t1); while (!pq.empty()) { Integer t2 = pq. deleteMin(); System.out.println(t2); } Assume n is the size of the input list, and assume that the printing out (displaying) of each of the elements takes a constant time, 0O (1). What is the worst-case time complexity of this algorithm in terms of Big-O, if the Priority Queue is implemented using: i) An unsorted linked list. ii) A sorted array. iii) A binary search tree. iv) An AVL tree. > Important: Notice that we are not only interested in the answer; but also, and most importantly, on how did you come to that conclusion. You must provide the full details on how your reached that decision. B.
Consider the algorithm below, which sorts a list of n elements by using a Priority Queue (the algorithm does not actually sort the list; rather just displays the sorted contents of the list.) public static void sort(List ts) { PriorityQueue pq = new PriorityQueue(); for (Integer t1 : ts) pq. insert(t1); while (!pq.empty()) { Integer t2 = pq. deleteMin(); System.out.println(t2); } Assume n is the size of the input list, and assume that the printing out (displaying) of each of the elements takes a constant time, 0O (1). What is the worst-case time complexity of this algorithm in terms of Big-O, if the Priority Queue is implemented using: i) An unsorted linked list. ii) A sorted array. iii) A binary search tree. iv) An AVL tree. > Important: Notice that we are not only interested in the answer; but also, and most importantly, on how did you come to that conclusion. You must provide the full details on how your reached that decision. B.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Data structure

Transcribed Image Text:Consider the algorithm below, which sorts a list of n elements by using a Priority Queue (the
algorithm does not actually sort the list; rather just displays the sorted contents of the list.)
public static void sort(List<Integer> ts) {
PriorityQueue<Integer> pq = new PriorityQueue<Integer>();
for (Integer t1 : ts)
pq. insert(t1);
while (!pq.empty()) {
Integer t2 =
pq. deleteMin();
System.out.println(t2);
}
Assume n is the size of the input list, and assume that the printing out (displaying) of each of the
elements takes a constant time, 0O (1). What is the worst-case time complexity of this algorithm in
terms of Big-O, if the Priority Queue is implemented using:
i) An unsorted linked list.
ii) A sorted array.
iii) A binary search tree.
iv) An AVL tree.
> Important: Notice that we are not only interested in the answer; but also, and most
importantly, on how did you come to that conclusion. You must provide the full details on
how your reached that decision.
B.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
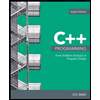
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
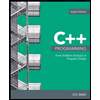
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning