HW2_SUMMER
.py
keyboard_arrow_up
School
Pennsylvania State University *
*We aren’t endorsed by this school
Course
132
Subject
Computer Science
Date
Feb 20, 2024
Type
py
Pages
10
Uploaded by MateWasp883 on coursehero.com
import random
class Course:
'''
>>> c1 = Course('CMPSC132', 'Programming in Python II', 3)
>>> c2 = Course('CMPSC360', 'Discrete Mathematics', 3)
>>> c1 == c2
False
>>> c3 = Course('CMPSC132', 'Programming in Python II', 3)
>>> c1 == c3
True
>>> c1
CMPSC132(3): Programming in Python II
>>> c2
CMPSC360(3): Discrete Mathematics
>>> c3
CMPSC132(3): Programming in Python II
>>> c1 == None
False
>>> print(c1)
CMPSC132(3): Programming in Python II
'''
#Constructor
def __init__(self, cid, cname, credits):
self.cid = cid
self.cname = cname
self.credits = credits
pass
# Method to return string display
def __str__(self):
return f"{self.cid}({self.credits}): {self.cname}"
pass
__repr__ = __str__
#To see for equality
def __eq__(self, other):
if(other is None):
return False
if(self.cid==other.cid):
return True
else:
return False
def isValid(self):
if(type(self.cid)==str and type(self.cname)==str and type(self.credits)==int):
return True
else:
return False
class Catalog:
''' >>> C = Catalog()
>>> C.courseOfferings
{}
>>> C._loadCatalog("cmpsc_catalog_small.csv")
>>> C.courseOfferings
{'CMPSC 132': CMPSC 132(3): Programming and Computation II, 'MATH 230':
MATH 230(4): Calculus and Vector Analysis, 'PHYS 213': PHYS 213(2): General Physics, 'CMPEN 270': CMPEN 270(4): Digital Design, 'CMPSC 311': CMPSC 311(3): Introduction to Systems Programming, 'CMPSC 360': CMPSC 360(3): Discrete Mathematics for Computer Science}
>>> C.removeCourse('CMPSC 360')
'Course removed successfully'
>>> C.courseOfferings
{'CMPSC 132': CMPSC 132(3): Programming and Computation II, 'MATH 230': MATH 230(4): Calculus and Vector Analysis, 'PHYS 213': PHYS 213(2): General Physics, 'CMPEN 270': CMPEN 270(4): Digital Design, 'CMPSC 311': CMPSC 311(3): Introduction to Systems Programming}
>>> isinstance(C.courseOfferings['CMPSC 132'], Course)
True
'''
def __init__(self):
self.courseOfferings = {}
pass
def addCourse(self, cid, cname, credits):
if cid not in self.courseOfferings:
self.courseOfferings[cid] = (Course(cid,cname,credits))
return "Course added successfully"
else:
return "Course already added"
pass
def removeCourse(self, cid):
if cid not in self.courseOfferings:
return "Course not found"
else:
del self.courseOfferings[cid]
return "Course removed successfully"
pass
def _loadCatalog(self, file):
with open(file, "r") as f:
course_info = f.read()
lst=course_info.split("\n")
for i in lst:
i = i.split(',')
self.cid = i[0]
self.cname = i[1]
self.credits = i[2]
self.courseOfferings[i[0]] = (Course(self.cid,self.cname,self.credits))
class Semester:
'''
>>> cmpsc131 = Course('CMPSC 131', 'Programming in Python I', 3)
>>> cmpsc132 = Course('CMPSC 132', 'Programming in Python II', 3)
>>> math230 = Course("MATH 230", 'Calculus', 4)
>>> phys213 = Course("PHYS 213", 'General Physics', 2)
>>> econ102 = Course("ECON 102", 'Intro to Economics', 3)
>>> phil119 = Course("PHIL 119", 'Ethical Leadership', 3)
>>> spr22 = Semester()
>>> spr22
No courses
>>> spr22.addCourse(cmpsc132)
>>> isinstance(spr22.courses['CMPSC 132'], Course)
True
>>> spr22.addCourse(math230)
>>> spr22
CMPSC 132; MATH 230
>>> spr22.isFullTime
False
>>> spr22.totalCredits
7
>>> spr22.addCourse(phys213)
>>> spr22.addCourse(econ102)
>>> spr22.addCourse(econ102)
'Course already added'
>>> spr22.addCourse(phil119)
>>> spr22.isFullTime
True
>>> spr22.dropCourse(phil119)
>>> spr22.addCourse(Course("JAPNS 001", 'Japanese I', 4))
>>> spr22.totalCredits
16
>>> spr22.dropCourse(cmpsc131)
'No such course'
>>> spr22.courses
{'CMPSC 132': CMPSC 132(3): Programming in Python II, 'MATH 230': MATH 230(4): Calculus, 'PHYS 213': PHYS 213(2): General Physics, 'ECON 102': ECON 102(3): Intro to Economics, 'JAPNS 001': JAPNS 001(4): Japanese I}
'''
def __init__(self):
self.courses = {}
pass
#To convert into string
def __str__(self):
if len(self.courses)!=0:
self.l1=list(self.courses.keys())
return ('; '.join(self.l1))
else:
return "No courses"
pass
__repr__ = __str__
def addCourse(self, course):
if course.cid not in self.courses:
self.courses[course.cid]=(course)
else:
return "Course already added"
pass
def dropCourse(self, course):
if course.cid in self.courses:
self.courses.pop(course.cid)
else:
return "No such course"
pass
@property
def totalCredits(self):
totalcredits = 0
for course in self.courses:
totalcredits += self.courses[course].credits
return totalcredits
pass
@property
def isFullTime(self):
return (self.totalCredits>=12)
pass
class Loan:
'''
>>> import random
>>> random.seed(2) # Setting seed to a fixed value, so you can predict what numbers the random module will generate
>>> first_loan = Loan(4000)
>>> first_loan
Balance: $4000
>>> first_loan.loan_id
17412
>>> second_loan = Loan(6000)
>>> second_loan.amount
6000
>>> second_loan.loan_id
22004
>>> third_loan = Loan(1000)
>>> third_loan.loan_id
21124
'''
def __init__(self, amount):
self.amount = amount
self.loan_id = self.__getloanID
pass
def __str__(self):
return f"Balance: ${self.amount}"
pass
__repr__ = __str__
@property
def __getloanID(self):
return random.randint(10000, 99999)
pass
class Person:
'''
>>> p1 = Person('Jason Lee', '204-99-2890')
>>> p2 = Person('Karen Lee', '247-01-2670')
>>> p1
Person(Jason Lee, ***-**-2890)
>>> p2
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
26. Assume the declaration of Exercise 24.
A. Write the statements that derive
the class dummyClass from class base as a private inheritance. (2
lines are { and }.)
"A1 is {
"A2 is
"A3 is ))
B. Determine which members of class base are private, protected,
and public in class dummyClass.
"B1 is dummyClass is a de
arrow_forward
class Vehicle
{
private:
int wheels;
protected :
int passenger:
public :
void inputdata(int, int);
void outputdata();
};
class Heavyvehicle : protected Vehicle
{
int diesel_petrol;
protected :
int load;
public:
void readdata(int, int);
void writedata();
};
class Bus : private Heavyvehicle
char make[20];
public :
void fetchdata(char);
void displaydata();
(1) Name the base class and derived class of the class Heavyvehicle.
(ii) Name the data member(s) that can be accessed from function displaydata().
(iii) Name the data member's that can be accessed by an object of Bus class.
(iv) Is the member function outputdata() accessible to the objects of Heavyvehicle class.
arrow_forward
Implement all the classes using Java programming language from the given UML Class diagram.
Note: This problem requires you to submit just two classes: Customer.java, Account.java.
Do NOT include "public static void main()" method inside all of these classes. Graders will be testing your classes, using the unit-testing framework JUnit 4.
Customer
- ID:int
-name:String
-gender:char
'm' or 'f'
+Customer(ID:int,name:String,
discount:int)
+getID():int
+getName ():String
+getGender ():char
+toString():String
"name (ID)"
The Customer class models, customer design as shown in the class diagram. Write the codes for the Customer class and a test driver to test all the public
methods.
Account
arrow_forward
IN C++
Lab #6: Shapes
Create a class named Point.
private attributes x and y of integer type.
Create a class named Shape.
private attributes:
Point points[6]
int howManyPoints;
Create a Main Menu:
Add a Triangle shape
Add a Rectangle shape
Add a Pentagon shape
Add a Hexagon shape
Exit
All class functions should be well defined in the scope of this lab.
Use operator overloading for the array in Shape class.
Once you ask the points of any shape it will display in the terminal the points added.
arrow_forward
class Test { char paper[20]; int marks; public: Test () // Function 1 { strcpy (paper, "Computer"); marks = 0; } Test (char p[]) // Function 2 { strcpy(paper, p); marks = 0; } Test (int m) // Function 3 { strcpy(paper,"Computer"); marks = m; } Test (char p[], int m) // Function 4 { strcpy (paper, p); marks = m; } };
i. Write statements in C++ that would execute Function 1, Function 2, Function 3 and Function 4 of class Test.
ii. Which feature of Object Oriented Programming is demonstrated using Function 1, Function 2, Function 3 and Function 4 together in the above class Test?
arrow_forward
MindTap:
In C#, Write a program named Averages that includes a method named Average that accepts any number of numeric parameters, displays them, and displays their average.
Test your function in your Main(). Tests will be run against Average() to determine that it works correctly when passed one, two, or three numbers, or an array of numbers.
arrow_forward
Question in Python
{
"logs": [
{
"logType": “xxxxx”
},
{
"logType": “43435”
},
{
"logType": “count”
},
{
"logType": “4452”
},
{
"logType": “count”
},
{
"logType": “count”
},
{
"logType": “43435”
},
{
"logType": "count"
},
{
"logType": "count"
}
}
So, please count the total of "logType": “count”
My desired output:
{
"count”: 5
}
arrow_forward
int a = -2, b = 5, c = 1, d = 11, e = -5;
int x = e + d - 2;
a = b - c + x;
b = e * x + 5;
c = d / 3 - x;
d = b + c - 7;
e = a + c - x;
a =
b =
c =
d =
e =
arrow_forward
Trace through the following program and show the output. Show your work for partial credit.
public class Employee {
private static int empID = 1111l;
private String name , position;
double salary;
public Employee(String name) {
empID ++;
this.name 3 пате;
}
public Employee(Employee obj) {
empID = obj.empĪD;
пате %3D оbj.naте;
position = obj.position;
%3D
public void empPosition(String empPosition) {position = empPosition;}
public void empSalary(double empSalary) { salary = empSalary;}
public String toString() {
return name + " "+empID + "
"+ position +" $"+salary;
public void setName(String empName){ name = empName;}
public static void main(String args[]) {
Employee empOne = new Employee("James Smith"), empTwo;
%3D
empOne.empPosition("Senior Software Engineer");
етрOпе.етpSalary(1000);
System.out.println(empOne);
еmpTwo
empTwo.empPosition("CEO");
System.out.println(empOne);
System.out.println(empTwo);
%3D етpОпе
empOne ;
arrow_forward
Use Python Programming Language
(The Stock class) Design a class named Stock to represent a company’s stock that contains:
A private string data field named symbol for the stock’s symbol.
A private string data field named name for the stock’s name.
A private float data field named previousClosingPrice that stores the stock price for the previous day.
A private float data field named currentPrice that stores the stock price for the current time.
A constructor that creates a stock with the specified symbol, name, previous price, and current price.
A get method for returning the stock name.
A get method for returning the stock symbol.
Get and set methods for getting/setting the stock’s previous price.
Get and set methods for getting/setting the stock’s current price.
A method named getChangePercent() that returns the percentage changed from previousClosingPrice to currentPrice.
Write a test program that creates a Stock object with the stock symbol INTC, the name Inte lCorporation, the…
arrow_forward
//Movie.java
import java.util.Arrays;
public class Movie {
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
// default constructorpublic Movie() {
movieName = "Flick";
numMinutes = 0;
isKidFriendly = false;
numCastMembers = 0;
castMembers = new String[10];
}
// overloaded parameterized constructorpublic Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) {
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
//numCastMember is set to array lengththis.numCastMembers = castMembers.length;
this.castMembers = castMembers;
}
public String getMovieName() {
return this.movieName;
}
public int getNumMinutes() {
return this.numMinutes;
}
public boolean getIsKidFriendly() {
return this.isKidFriendly;
}
public boolean isKidFriendly() {
return this.isKidFriendly;
}
public int getNumCastMembers() {
return this.numCastMembers;
}
public…
arrow_forward
Instructions:
In the code editor, you are provided with a treasureChestMagic() function which has the following description:
Return type - void
Name - treasureChestMagic
Parameters - an address of an integer
Description - updates the value of a certain integer randomly
You do not have to worry about how the treasureChestMagic() function works. All you have to do is ask the user for an integer and then call the treasureChestMagic() function, passing the address of that integer you just asked.
Finally, print the updated value of the integer inputted by the user.
Please create a main code for my function that works with this.
This is my code:
#include<stdio.h>#include<math.h>
void treasureChestMagic(int*);
int main(void) { // TODO: Write your code here
return 0;}
void treasureChestMagic(int *n) { int temp = *n; int temp2 = temp;
*n = *n + 5 - 5 * 5 / 5;
temp2 = (int) pow(2, 3);
if(temp % 3 == 0) { *n = temp * 10; } else if(temp %…
arrow_forward
Enter the name of Stock: Oracle Corporation
Enter the symbol of Stock: URCL
Enter the previous closing price: 34.5
Enter the current price: 34.35
Stock name: Oracle Corporation
Stock symbol: ORCL
Stock ID: 59
Price-change percentage: - 0.434782608695648
Challenge Question - Using GregorianCalendar and Date classes:
(Use the GregorianCalendar class) Java API has the Date class and GregorianCalendar class in
the java.util package, which you can use to obtain the current date with specific details like
current year, current month, current day of a date and so on.
Write a program to perform these tasks:
1. Display the current year, month, and day.
2. Display the current date by using the to String() method from the Date class.
3. Display the current time-which is used to display the number of milliseconds- by
using getTime() method from the Date class. We will use it in the step 17.
4. - Display the current year by using get(GregorianCalendar.YEAR) method from to
GregorianCale class.
5-…
arrow_forward
In Python: Write a class named Pet, which should have the following data attributes:
_ _name (for the name of a pet)
_ _animal_type (for the type of animal that a pet is. Example values are 'Dog','Cat', and 'Bird')
_ _age (for the pets age)
The Pet class should have an _ _init_ _ method that creates these attributes. It should also have the following methods:
set_nameThis method assigns a value to the _ _name field
set_animal_typeThis method assigns a value to the _ _animal_type field
set_ageThis method assignsa value to the _ _age field
get_nameThis method assignsa value to the _ _name field
get_animal_typeThis method assignsa value to the _ _animal_type field
get_ageThis method assignsa value to the _ _age field
Once you have written the class, write a program that creates an object of the class and prompts the user to enter the name, type and age of his or her pet. This data should be stored as the objects attributes. Use the objects accessor methods to retrieve the pets…
arrow_forward
Enter the name of Stock: Oracle Corporation
Enter the symbol of Stock: URCL
Enter the previous closing price: 34.5
Enter the current price: 34.35
Stock name: Oracle Corporation
Stock symbol: ORCL
Stock ID: 59
Price-change percentage: - 0.434782608695648
Challenge Question - Using GregorianCalendar and Date classes:
(Use the GregorianCalendar class) Java API has the Date class and GregorianCalendar class in
the java.util package, which you can use to obtain the current date with specific details like
current year, current month, current day of a date and so on.
Write a program to perform these tasks:
1. Display the current year, month, and day.
2. Display the current date by using the to String() method from the Date class.
3. Display the current time-which is used to display the number of milliseconds- by
using getTime() method from the Date class. We will use it in the step 17.
4. - Display the current year by using get(GregorianCalendar.YEAR) method from to
GregorianCale class.
5-…
arrow_forward
Written in Python
It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method.
Docstrings for modules, functions, classes, and methods
arrow_forward
As a starting point, use the files fraction.h, fraction.cpp, testfraction.cpp below:
// simple class for fractions
#ifndef FRACTION_H_#define FRACTION_H_
class Fraction {
private: // internal implementation is hidden int num; // numerator int den; // denominator
int gcd(int a, int b); // calculates the gcd of a and b int lcm(int a, int b);
public: Fraction(); // empty constructor Fraction(int, int = 1); // constructor taking values for fractions and // integers. Denominator by default is 1 void print(); // prints it to the screen};
#endif /* FRACTION_H_ */
#include <iostream>#include "fraction.h"
Fraction::Fraction(){ num = 1; den = 1;}
Fraction::Fraction(int n, int d){ int tmp_gcd = gcd(n, d);
num = n / tmp_gcd; den = d / tmp_gcd;}
int Fraction::gcd(int a, int b){ int tmp_gcd = 1;
// Implement…
arrow_forward
A
return;
else
#include
using namespace std;
void division (int num, int denom);
int main()
{ division (5, -1);
division (10, 2);
return 0; }
void division (int num, int denom)
{if (denom
using namespace std;
int main()
(char List[4][10] = {"kareem", "ahmed", "ali", "mohamed"};
cout<
arrow_forward
True or false ?
Assuming
class Quiz { public: void TakeIt ( ); }; Quiz cs2b_quiz; Quiz * p_quiz = & cs2b_quiz;
The syntax to invoke TakeIt ( ) member function using the pointer is:
p_quiz->TakeIt ( );
arrow_forward
Write program in C++ Language
The other solutions on chegg were incorrect!
Please read carefully!
Write a program that defines and tests a class called COP3014. The class resembles aclassroom. It has the following grading scheme:3 quizzes 20 points each 20%Mid term 100 points 30%Final 100 points 50%Based on the total grade of any student for the course, the letter grade of each student can becomputed as follows:Any grade of 90 or more is an A, any grade of 80 or more (but less than 90) is a B, any grade of70 or more (but less than 80) is a C, any grade of 60 or more (but less than 70) is a D, and anygrade below 60 is an F.This class needs to have some member variables. The first name, last name, the Z-number, thegrades, the total grade and final letter grade are all considered to be private member variablesof this class.The class should also have the following public member functions/procedural attributes that canbring the objects (students of the class) to life and give them some…
arrow_forward
class Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list);
Task:
Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…
arrow_forward
public class LabProgram {
public static void main(String args[]) {
Course course = new Course();
String first; // first name
String last; // last name
double gpa; // grade point average
first = "Henry";
last = "Cabot";
gpa = 3.5;
course.addStudent(new Student(first, last, gpa)); // Add 1st student
first = "Brenda";
last = "Stern";
gpa = 2.0;
course.addStudent(new Student(first, last, gpa)); // Add 2nd student
first = "Jane";
last = "Flynn";
gpa = 3.9;
course.addStudent(new Student(first, last, gpa)); // Add 3rd student
first = "Lynda";
last = "Robison";
gpa = 3.2;
course.addStudent(new Student(first, last, gpa)); // Add 4th student
course.printRoster();
}
}
// Class representing a student
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average…
arrow_forward
dictionaries = []dictionaries.append({"First":"Bob", "Last":"Jones", "Color":"red"})dictionaries.append({"First":"Harpreet", "Last":"Kaur", "Color":"green"})dictionaries.append({"First":"Mohamad", "Last":"Argani", "Color":"blue"})for i in range(0, -1,len(dictionaries)):print(dictionaries[i]['First'])
********************
Please modify the code, so the result will show first, last names and colour separated by a single space
arrow_forward
class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const;
temporary(string = "", double = 0.0, double = 0.0);
private: string description; double first; double second; };
What are the members and functions in this example? I need help identifying how the members and functions work to answer the questions below. I am having troubles understanding this concept. I am learning constructors and deconstructors along with inline and hiding information. I feel lost trying to answer these questions. Can I get direction on how to proceed?
Write the definition of the member function set so that the instance variables are set according to the parameters.
Write the definition of the member function manipulate that returns a decimal number as follows: If the…
arrow_forward
class Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list);
Implement the function Singer Singer::combine(const Singer& rhs):It should create a new Singer object, by calling the constructor with the following values:
For name it should pass a combination of be the name of the calling object followed by a '+' and then…
arrow_forward
class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const;
temporary(string = "", double = 0.0, double = 0.0);
private: string description; double first; double second; };
I need help writing the definition of the member function set so the instance varialbes are set according to the parameters.
I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.
arrow_forward
class Currency {
protected:
int whole;
int fraction;
virtual std::string get_name() = 0;
public:
Currency() {
whole = 0;
fraction = 0;
}
Currency(double value) {
if (value < 0)
throw "Invalid value";
whole = int(value);
fraction = std::round(100 * (value - whole));
}
Currency(const Currency& curr) {
whole = curr.whole;
fraction = curr.fraction;
}
/* This algorithm gets the whole part or fractional part of the currency
Pre: whole, fraction - integer numbers
Post:
Return: whole or fraction
*/
int get_whole() { return whole; }
int get_fraction() { return fraction; }
/* This algorithm adds an object to the same currency
Pre: object (same currency)
Post:
Return:
*/
void set_whole(int w) {
if (w >= 0)
whole = w;
}
void set_fraction(int f) {
if (f >= 0 && f <…
arrow_forward
class Clock {
public: Clock() : hourValue_(0), minuteValue_(0), secondValue_(0) {}; Clock(const int hourValue, const int minuteValue, const int secondValue) : hourValue_(hourValue), minuteValue_(minuteValue), secondValue_(secondValue) {};
virtual std::string ShowTime(void) = 0; virtual void SetTime(int hourValue, int minuteValue, int secondValue) = 0;
int hourValue_; int minuteValue_; int secondValue_;
int GetHour() { return hourValue_; }; int GetMinute() { return minuteValue_; }; int GetSecond() { return secondValue_; };
void SetHour(const int hourValue) { hourValue_ = hourValue; }; void SetMinute(const int minuteValue) { minuteValue_ = minuteValue; }; void SetSecond(const int secondValue) { secondValue_ = secondValue; };};
class DigitalClock final : public Clock {
public: DigitalClock(const int hourValue, const int minuteValue, const int secondValue);
virtual std::string ShowTime(void) override; virtual void SetTime(const int…
arrow_forward
2. The MyInteger Class
Problem Description:
Design a class named MyInteger. The class contains:
n
[]
[]
A private int data field named value that stores the int
value represented by this object.
A constructor that creates a MyInteger object for the
specified int value.
A get method that returns the int value.
Methods isEven () and isOdd () that return true if the value
is even or odd respectively.
Static methods isEven (int) and isOdd (int) that return true
if the specified value is even or odd respectively.
Static methods isEven (MyInteger) and isOdd (MyInteger) that
return true if the specified value is even or odd
respectively.
Methods equals (int) and equals (MyInteger) that return true
if the value in the object is equal to the specified value.
Implement the class. Write a client program that tests all
methods in the class.
arrow_forward
C# languageCreate a class for “Plane” having functionalities (methods) startengine(), fly() and land(). When ever engine starts it should reset the attribute TTK (Total travel kilometer) to zero and attribute Fuel to 100. On fly() it should add 10 kilometers if the Fuel attribute is greater than zero and decrement Fuel by 20.On land() it should print total distance covered. Write the code providing all necessary details. And then show working object of Plane in main().
arrow_forward
Classes, Objects, Pointers and Dynamic Memory
Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name.
The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version).
Your MYString class needs to be written using the .h and .cpp format.
Inside the class we will have the following data members:
Member Data
Description
char * str
pointer to dynamic memory for storing the string
int cap
size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough)
int end
index of the end of the string (the '\0' char)
The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…
arrow_forward
account.json
"1000001": { "accountType": "Chequing", "accountBalance": 0 }, "1000002": { "accountType": "Savings", "accountBalance": 0 }, "1000011": { "accountType": "Chequing", "accountBalance": 0 }, "1000022": { "accountType": "Savings", "accountBalance": 0 }, "1000031": { "accountType": "Chequing", "accountBalance": 0 }, "1000032": { "accountType": "Savings", "accountBalance": 0 }, "1000051": { "accountType": "Chequing", "accountBalance": 13.699999999999989 }, "1000052": { "accountType": "Savings", "accountBalance": 0 }, "1000071": { "accountType": "Chequing", "accountBalance": 0 }, "1000081": { "accountType": "Savings", "accountBalance": 0 }, "1000091": { "accountType": "Chequing", "accountBalance": 0 }, "lastID": "1000091"}
-------
var express =…
arrow_forward
8- Create an instance of the following class and call its methods.
class Exam{
int grade;
public:
void seta (int b) (grade=b; }
int geta () {return grade; }
9- When the word const is put before the variable definition, what does that mean?
10- How to concatenate two strings in C++ language?
C++
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
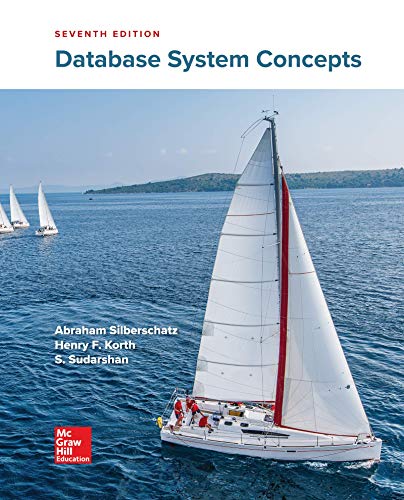
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
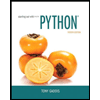
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
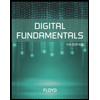
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
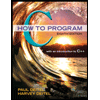
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
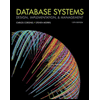
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
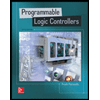
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- 26. Assume the declaration of Exercise 24. A. Write the statements that derive the class dummyClass from class base as a private inheritance. (2 lines are { and }.) "A1 is { "A2 is "A3 is )) B. Determine which members of class base are private, protected, and public in class dummyClass. "B1 is dummyClass is a dearrow_forwardclass Vehicle { private: int wheels; protected : int passenger: public : void inputdata(int, int); void outputdata(); }; class Heavyvehicle : protected Vehicle { int diesel_petrol; protected : int load; public: void readdata(int, int); void writedata(); }; class Bus : private Heavyvehicle char make[20]; public : void fetchdata(char); void displaydata(); (1) Name the base class and derived class of the class Heavyvehicle. (ii) Name the data member(s) that can be accessed from function displaydata(). (iii) Name the data member's that can be accessed by an object of Bus class. (iv) Is the member function outputdata() accessible to the objects of Heavyvehicle class.arrow_forwardImplement all the classes using Java programming language from the given UML Class diagram. Note: This problem requires you to submit just two classes: Customer.java, Account.java. Do NOT include "public static void main()" method inside all of these classes. Graders will be testing your classes, using the unit-testing framework JUnit 4. Customer - ID:int -name:String -gender:char 'm' or 'f' +Customer(ID:int,name:String, discount:int) +getID():int +getName ():String +getGender ():char +toString():String "name (ID)" The Customer class models, customer design as shown in the class diagram. Write the codes for the Customer class and a test driver to test all the public methods. Accountarrow_forward
- IN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forwardclass Test { char paper[20]; int marks; public: Test () // Function 1 { strcpy (paper, "Computer"); marks = 0; } Test (char p[]) // Function 2 { strcpy(paper, p); marks = 0; } Test (int m) // Function 3 { strcpy(paper,"Computer"); marks = m; } Test (char p[], int m) // Function 4 { strcpy (paper, p); marks = m; } }; i. Write statements in C++ that would execute Function 1, Function 2, Function 3 and Function 4 of class Test. ii. Which feature of Object Oriented Programming is demonstrated using Function 1, Function 2, Function 3 and Function 4 together in the above class Test?arrow_forwardMindTap: In C#, Write a program named Averages that includes a method named Average that accepts any number of numeric parameters, displays them, and displays their average. Test your function in your Main(). Tests will be run against Average() to determine that it works correctly when passed one, two, or three numbers, or an array of numbers.arrow_forward
- Question in Python { "logs": [ { "logType": “xxxxx” }, { "logType": “43435” }, { "logType": “count” }, { "logType": “4452” }, { "logType": “count” }, { "logType": “count” }, { "logType": “43435” }, { "logType": "count" }, { "logType": "count" } } So, please count the total of "logType": “count” My desired output: { "count”: 5 }arrow_forwardint a = -2, b = 5, c = 1, d = 11, e = -5; int x = e + d - 2; a = b - c + x; b = e * x + 5; c = d / 3 - x; d = b + c - 7; e = a + c - x; a = b = c = d = e =arrow_forwardTrace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forward
- Use Python Programming Language (The Stock class) Design a class named Stock to represent a company’s stock that contains: A private string data field named symbol for the stock’s symbol. A private string data field named name for the stock’s name. A private float data field named previousClosingPrice that stores the stock price for the previous day. A private float data field named currentPrice that stores the stock price for the current time. A constructor that creates a stock with the specified symbol, name, previous price, and current price. A get method for returning the stock name. A get method for returning the stock symbol. Get and set methods for getting/setting the stock’s previous price. Get and set methods for getting/setting the stock’s current price. A method named getChangePercent() that returns the percentage changed from previousClosingPrice to currentPrice. Write a test program that creates a Stock object with the stock symbol INTC, the name Inte lCorporation, the…arrow_forward//Movie.java import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructorpublic Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } // overloaded parameterized constructorpublic Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; //numCastMember is set to array lengththis.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() { return this.isKidFriendly; } public int getNumCastMembers() { return this.numCastMembers; } public…arrow_forwardInstructions: In the code editor, you are provided with a treasureChestMagic() function which has the following description: Return type - void Name - treasureChestMagic Parameters - an address of an integer Description - updates the value of a certain integer randomly You do not have to worry about how the treasureChestMagic() function works. All you have to do is ask the user for an integer and then call the treasureChestMagic() function, passing the address of that integer you just asked. Finally, print the updated value of the integer inputted by the user. Please create a main code for my function that works with this. This is my code: #include<stdio.h>#include<math.h> void treasureChestMagic(int*); int main(void) { // TODO: Write your code here return 0;} void treasureChestMagic(int *n) { int temp = *n; int temp2 = temp; *n = *n + 5 - 5 * 5 / 5; temp2 = (int) pow(2, 3); if(temp % 3 == 0) { *n = temp * 10; } else if(temp %…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
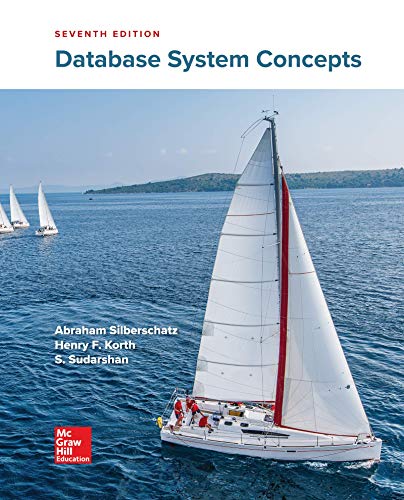
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
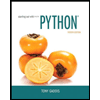
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
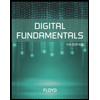
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
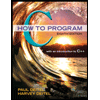
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
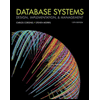
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
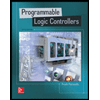
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education