Java programming Project 1 had all the calculations occurring in the main program. For this project, you are to modularize your program. Use functions as much as you can and use Arrays for your menu. be sure that the names you come up with for your modules are verbs and follow case conventions. Please do not create a function for every single input or calculation! Programmers group statements together that accomplish one task, such as getting input from the user and name them appropriately, such as getInput. Make sure every input display cost or money owed. The results of running your program should be identical to the results you obtained in Project 1 (either your original version or a corrected version if there were errors). If the money given is not enough, make sure you loop back or with decision structure to ask for more money. This is project 1: // Project 1 //// This program is designed to take a customers food order and generate a recipt displaying their purchase, the qauntity, amount due, and tax amount, and total cost after tax deducted. //To solve the issue I coded and tested and tested my code multiple times to make sure that there were no syntax or logic errors. //Import to be able to get user input for your receipt import java.util.Scanner; public class Main { publicstaticvoid main(String[] args){ //define your constants finalfloat FRIES = 2.99F; // set the price for your products finalfloat CHEESESTEAK = 5.99F; finalfloat BISCUIT = .99F; finalfloat SALADBOWL = 5.99F; finalfloat DONUTBOX = 10.99F; finalfloat SALESTAX = .06F; //Get input from the user //ask the user questions Scanner sc= new Scanner(System.in); System.out.println("How many Fries would you like to order? (each at: " + FRIES + ")"); //calculate total for the fries int numFRIES = sc.nextInt(); //get second input from the user System.out.println("How many CheeseSteaks would you like to order? (each at: " + CHEESESTEAK + ")"); int numCHEESESTEAK = sc.nextInt(); //Get third input from the user System.out.println("How many Biscuits would you like to order? (each at: " + BISCUIT + ")"); int numBISCUIT = sc.nextInt(); //Get fifth input from the user System.out.println("How many SaladBowls would you like to order? (each at: " + SALADBOWL + ")"); int numSALADBOWL = sc.nextInt(); //Get input for the the last order item System.out.println("How many Donut Boxes would you like to order? (each at: " + DONUTBOX + ")"); int numDONUTBOX = sc.nextInt(); //calculate total price for items based on amount ordered (not including tax deducted) float costFRIES = FRIES * numFRIES; float costCHEESESTEAK = CHEESESTEAK * numCHEESESTEAK; float costBISCUIT = BISCUIT * numBISCUIT; float costSALADBOWL = SALADBOWL * numSALADBOWL; float costDONUTBOX = DONUTBOX * numDONUTBOX; //Calculate the subtotal for all your items combined float SubTotal = costFRIES + costCHEESESTEAK + BISCUIT + SALADBOWL + DONUTBOX; float TAX = SubTotal * SALESTAX; //Calculate the total amount of taxes deducted from a customers order float total = SubTotal + TAX; //Ask user input System.out.println("How much will you be paying ? (Total is " + total + ")"); float tender = sc.nextFloat(); //calculate the tender and change float change = tender - total; //Receipt displayal starts here System.out.println("\t\t\t\t\t\tTop Cuisine");//display the name of your restaurant System.out.println("\t\t\t\t\t\t1267 Loongton st.");//display the address and phone number System.out.println("\t\t\t\t\thyattsville, Maryland 28746"); System.out.println("\t\t\t\t\t\t301-273-378"); System.out.println(numFRIES + " Fries each at: $" + FRIES + "\t\t\t\t\t\t\t\t $" +costFRIES); //Display items quantity ordered, and cost per individual item System.out.println(numCHEESESTEAK + " Cheesesteaks each at: $" + CHEESESTEAK + "\t\t\t\t\t\t $" +costCHEESESTEAK); //Display items quantity ordered, and cost per individual item System.out.println(numBISCUIT + " Biscuits each at: $" + BISCUIT + "\t\t\t\t\t\t\t\t $" +costBISCUIT);//Display items quantity ordered, and cost per individual item System.out.println(numSALADBOWL + " Salad Bowls each at: $" + SALADBOWL + "\t\t\t\t\t\t\t $" +costSALADBOWL);//Display items quantity ordered, and cost per individual item System.out.println(numDONUTBOX + " DONUT BOXES each at: $ " + DONUTBOX + "\t\t\t\t\t\t\t$ " +costDONUTBOX );//Display items quantity ordered, and cost per individual item //Display the subtotal, tax, tender, change and total after tax due System.out.println(" Subtotal :\t\t\t\t\t\t\t\t\t\t\t\t$"+SubTotal ); System.out.println(" Taxes : \t\t\t\t\t\t\t\t\t\t\t\t$" +TAX); System.out.println(" Total :\t\t\t\t\t\t\t\t\t\t\t\t$"+total); System.out.println(" Tender :\t\t\t\t\t\t\t\t\t\t\t\t$" +tender); System.out.println(" Change :\t\t\t\t\t\t\t\t\t\t\t\t$" +change); System.out.println("\t\t\t\t Thank You For Visiting ! Come Again!"); //Program finished } }
Java programming
Project 1 had all the calculations occurring in the main program. For this project, you are to modularize your program. Use functions as much as you can and use Arrays for your menu.
be sure that the names you come up with for your modules are verbs and follow case conventions. Please do not create a function for every single input or calculation! Programmers group statements together that accomplish one task, such as getting input from the user and name them appropriately, such as getInput. Make sure every input display cost or money owed. The results of running your program should be identical to the results you obtained in Project 1 (either your original version or a corrected version if there were errors). If the money given is not enough, make sure you loop back or with decision structure to ask for more money.
This is project 1:

import java.util.Scanner;
public class MainWithModules
{
final static String items[] = new String[]{"FRIES","CHEESESTEAK","BISCUIT","SALADBOWL","DONUTBOX","SALESTAX"};
final static float price[] = new float[]{2.99F,5.99F,.99F,5.99F,10.99F,.06F};
static float subTotal=0, TAX=0, total=0, tender=0,change=0;
public static void getInput(int [] ordered_items)
{
Scanner sc= new Scanner(System.in);
for(int i=0;i<5;i++)
{
System.out.println("How many "+ items[i] +" would you like to order? (each at: " + price[i] + ")");
ordered_items[i] = sc.nextInt();
}
}
public static void billCalculator(int [] ordered_items, float [] item_bill)
{
for(int i=0;i<5;i++)
{
item_bill[i] = ordered_items[i]*price[i];
}
for(int i=0;i<5;i++)
{
subTotal+=item_bill[i];
}
TAX = subTotal * price[5];
total = subTotal + TAX;
}
public static void printBill(int [] ordered_items, float [] item_bill)
{
System.out.println("\t\t\t\t\t\tTop Cuisine");
System.out.println("\t\t\t\t\t\t1267 Loongton st.");
System.out.println("\t\t\t\t\thyattsville, Maryland 28746");
System.out.println("\t\t\t\t\t\t301-273-378");
for(int i=0;i<5;i++)
{
System.out.println(ordered_items[i] + " " + items[i] + " each at: $" + price[i] + "\t\t\t\t\t\t\t\t $" + item_bill[i]);
}
System.out.println(" Subtotal :\t\t\t\t\t\t\t\t\t\t\t\t$"+subTotal );
System.out.println(" Taxes : \t\t\t\t\t\t\t\t\t\t\t\t$" +TAX);
System.out.println(" Total :\t\t\t\t\t\t\t\t\t\t\t\t$"+total);
System.out.println(" Tender :\t\t\t\t\t\t\t\t\t\t\t\t$" +tender);
System.out.println(" Change :\t\t\t\t\t\t\t\t\t\t\t\t$" +change);
System.out.println("\t\t\t\t Thank You For Visiting ! Come Again!");
}
public static void main(String[] args)
{
int ordered_items[] = new int[5];
float item_bill[] = new float[5];
getInput(ordered_items);
billCalculator(ordered_items, item_bill);
System.out.println("How much will you be paying ? (Total is " + total + ")");
Scanner sc= new Scanner(System.in);
while(true)
{
tender = sc.nextFloat();
if(tender<total)
{
System.out.println("You are paying "+(total-tender)+" less than the total bill");
System.out.println("Please re-enter the amount");
}
else
{
break;
}
}
change = tender - total;
printBill(ordered_items, item_bill);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

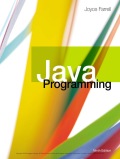
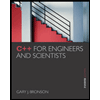
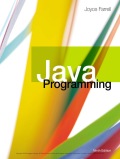
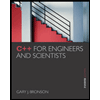
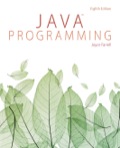
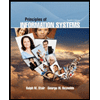
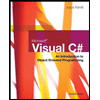