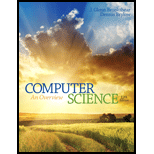
Computer Science: An Overview (12th Edition)
12th Edition
ISBN: 9780133760064
Author: Glenn Brookshear, Dennis Brylow
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 8, Problem 13CRP
Explanation of Solution
Function for reversing the order of a linked list:
//function for reversing the order of a linked list
//void Reverselist(Linkedlist list)
{
//creating pointers to the linked list
nodeType*current,*temp,*first;
//assign the head position to current node
current=head;
//make the first pointer to NULL
first=NULL;
//change the order of the list by using the while loop
while(current!=NULL)
{
first=temp;
current=first;
current=current->link;
}
//assign the head to the first pointer
first=head;
}
Explanation:
- Suppose *current, *first and *temp be any three points that point to the nodes in a list.
- The address of the head node is stored by the current pointer.
current=head;
- The NULL is initialized by the first pointer...
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
C Language
In a linear linked list, write a function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list.
C Language
In a linear linked list, write a function that deletes the element in the middle of the list (free this memory location) (if the list has 100 or 101 elements, it will delete the 50th element). The function will take a list as a parameter and return the updated list.
Write a C code for a doubly linked list with all its functions
Chapter 8 Solutions
Computer Science: An Overview (12th Edition)
Ch. 8.1 - Give examples (outside of computer science) of...Ch. 8.1 - Prob. 2QECh. 8.1 - Prob. 3QECh. 8.1 - Prob. 4QECh. 8.1 - Prob. 5QECh. 8.2 - In what sense are data structures such as arrays,...Ch. 8.2 - Prob. 2QECh. 8.2 - Prob. 3QECh. 8.3 - Prob. 1QECh. 8.3 - Prob. 2QE
Ch. 8.3 - Prob. 3QECh. 8.3 - Prob. 4QECh. 8.3 - Modify the function in Figure 8.19 so that it...Ch. 8.3 - Prob. 7QECh. 8.3 - Prob. 8QECh. 8.3 - Draw a diagram representing how the tree below...Ch. 8.4 - Prob. 1QECh. 8.4 - Prob. 2QECh. 8.4 - Prob. 3QECh. 8.4 - Prob. 4QECh. 8.5 - Prob. 1QECh. 8.5 - Prob. 3QECh. 8.5 - Prob. 4QECh. 8.6 - In what ways are abstract data types and classes...Ch. 8.6 - What is the difference between a class and an...Ch. 8.6 - Prob. 3QECh. 8.7 - Suppose the Vole machine language (Appendix C) has...Ch. 8.7 - Prob. 2QECh. 8.7 - Using the extensions described at the end of this...Ch. 8.7 - In the chapter, we introduced a machine...Ch. 8 - Prob. 1CRPCh. 8 - Prob. 2CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 4CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 6CRPCh. 8 - Prob. 7CRPCh. 8 - Prob. 8CRPCh. 8 - Prob. 9CRPCh. 8 - Prob. 10CRPCh. 8 - Prob. 11CRPCh. 8 - Prob. 12CRPCh. 8 - Prob. 13CRPCh. 8 - Prob. 14CRPCh. 8 - Prob. 15CRPCh. 8 - Prob. 16CRPCh. 8 - Prob. 17CRPCh. 8 - Prob. 18CRPCh. 8 - Design a function to compare the contents of two...Ch. 8 - (Asterisked problems are associated with optional...Ch. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 22CRPCh. 8 - Prob. 23CRPCh. 8 - Prob. 24CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 26CRPCh. 8 - Prob. 27CRPCh. 8 - Prob. 28CRPCh. 8 - Prob. 29CRPCh. 8 - Prob. 30CRPCh. 8 - Design a nonrecursive algorithm to replace the...Ch. 8 - Prob. 32CRPCh. 8 - Prob. 33CRPCh. 8 - Prob. 34CRPCh. 8 - Draw a diagram showing how the binary tree below...Ch. 8 - Prob. 36CRPCh. 8 - Prob. 37CRPCh. 8 - Prob. 38CRPCh. 8 - Prob. 39CRPCh. 8 - Prob. 40CRPCh. 8 - Modify the function in Figure 8.24 print the list...Ch. 8 - Prob. 42CRPCh. 8 - Prob. 43CRPCh. 8 - Prob. 44CRPCh. 8 - Prob. 45CRPCh. 8 - Prob. 46CRPCh. 8 - Using pseudocode similar to the Java class syntax...Ch. 8 - Prob. 48CRPCh. 8 - Identify the data structures and procedures that...Ch. 8 - Prob. 51CRPCh. 8 - In what way is a class more general than a...Ch. 8 - Prob. 53CRPCh. 8 - Prob. 54CRPCh. 8 - Prob. 55CRPCh. 8 - Prob. 1SICh. 8 - Prob. 2SICh. 8 - In many application programs, the size to which a...Ch. 8 - Prob. 4SICh. 8 - Prob. 5SICh. 8 - Prob. 6SICh. 8 - Prob. 7SICh. 8 - Prob. 8SI
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What is the biggest advantage of linked list over array? Group of answer choices Unlike array, linked list can dynamically grow and shrink With linked list, it is faster to access a specific element than with array Linked list is easier to implement than array Unlike array, linked list can only hold a fixed number of elementsarrow_forwardGiven an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list(this is not graded).arrow_forwardİn C language A singly linear list stores integer values in each node and has multiple nodes. Write a function using given prototype below. This function cuts the first node of the list and adds it to the end as last node. It takes beginning address of the list as a parameter and returns the updated list.struct node* cutheadaddlast(struct node* head);struct node { int number; struct node * next; };arrow_forward
- How does insertion of a new element vary between arrays and linked lists?arrow_forwardExplain the differences between a statically allocated array, a dynamically allocated array, and a linked list.arrow_forwardExplain the performance of different operations in Array List and Linked List. in javaarrow_forward
- 1. a function that takes in a list (L), and creates a copy of L. note: The function should return a pointer to the first element in the new L. [iteration and recursion]. 2. a function that takes in 2 sorted linked lists, and merges them into a single sorted list. note: This must be done in-place, and it must run in O(n+m).arrow_forwardIN PYTHON Linked Lists Consider the implementation of the Linked list class, implement the following functions as part of the class: index(item) returns the position of item in the list. It needs the item and returns the index. Assume the item is in the list. pop() removes and returns the last item in the list. It needs nothing and returns an item. Assume the list has at least one item. pop_pos(pos) removes and returns the item at position pos. It needs the position and returns the item. Assume the item is in the list. a function that counts the number of times an item occurs in the linked list a function that would delete the replicate items in the linked list (i.e. leave one occurrence only of each item in the linked list) Your main function should do the following: Generate 15 random integer numbers in the range from 1 to 5. Insert each number (Item in a node) in the appropriate position in a linked list, so you will have a sorted linked list in ascending order. Display the…arrow_forwardC++ ONLY Add the following functions to the linked list. int getSize() -> This function will return the number of elements in the linked-list. This function should work in O(1). For this keep track of a size variable and update it when we insert a new value in the linked-list. int getValue(index) -> This function will return the value present in the input index. If the index is greater or equal to the size of the linked-list return -1. void printReverse() -> This function will print the linked list in reverse order. You don’t need to reverse the linked list. Just need to print it in reverse order. You need to do this recursively. You cannot just take the elements in an array or vector and then print them in reverse order. void swapFirst() -> This function will swap the first two nodes in the linked list. If the linked-list contains less than 2 elements then just do nothing and return. To check your code add the following code in your main function. LinkedList l;…arrow_forward
- Write a C++ Function that returns the data of the middle node in a linked list and in case the linked list contains only one node return the data inside this node then returns the sum of all the nodes in linked list then returns the maximum data value in the linked listarrow_forwardThere are many differences between array and linked list, one of these differences: a. In a linked list insertion and deletion takes more time b. Array supports random access while linked List supports sequential access c. in linked list, elements are stored in contiguous memory location while in array are stored anywhere in the memory d. None of the abovearrow_forwardWrite a C++ Function that returns the data of the middle node in a linked list and in case the linked list contains only one node return the data inside this node.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
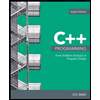
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning