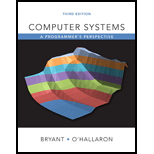
You are given the task of writing a function that will copy an integer val into a buffer buf, but it should do so only if enough space is available in the buffer.
Here is the code you write:
/* Copy integer into buffer if space is available */
/* WARNING: The following code is buggy */
void copy_int(int val; void *buf, int maxbytes) {
if (maxbytes-sizeof (val) >= 0)
memcpy(buf, (void *) &val, sizeof(val));
}
This code makes use of the library function memcpy. Although its use is a bit artificial here, where we simply want to copy an int, it illustrates an approach commonly used to copy larger data structures.
You carefully test the code and discover that it always copies the value to the buffer, even when maxbytes is too small.
- A. Explain why the conditional test in the code always succeeds. Hint: The sizeof operator returns a value of type size_t.
- B. Show how you can rewrite the conditional test to make it work properly.

Trending nowThis is a popular solution!
Learn your wayIncludes step-by-step video

Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
Digital Fundamentals (11th Edition)
Concepts Of Programming Languages
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Modern Database Management
Modern Database Management (12th Edition)
Absolute Java (6th Edition)
- 4. The following program loads and displays an image. Complete the modify function to change the brightness of the image. The parameter m is a multiplication factor. modify will will multiply each pixel by m - that means you need to multiply the red, green, and blue channels by m, create a new pixel with new red, green, and blue values, and replace the old pixel with the new pixel. Be sure none of the red, green, and blue values exceed 255 (if a value is greater than 255, set it to 255). Your program should work for images of any size. A good example is the sepia tone exercise. Do not change the main function, except to try different values for the multiplication factor. Save & Run Load History 1 import image 3 def modify(pic, m): '''Multiply each pixel by m to darken or brighten an image''' pass #You can leave this or remove it #Your code here to change the brightness of the image 4 7 8 def main(): 9. '''Controls the program'" fname…arrow_forwardIn C++, how is the readfile function written for this program?arrow_forwardWrite a function that reads all the numbers from a file into an array. The file is already open and there is no bad data in the file. The data is one number per line and the file has an unknown number of lines of data. Assume that the array is large enough to hold all the data. The data should be stored in the array starting at the first element. Prototype void readData( ifstream& iFile, double numbers[ ], int& count); iFile - already open ifstream numbers[ ] - array to store data count - the number of values read and must be set by the function before returning.arrow_forward
- : A monochrome screen is stored as a single array of bytes, allowing eight consecutivepixels to be stored in one byte. The screen has width w, where w is divisible by 8 (that is, no byte willbe split across rows). The height of the screen, of course, can be derived from the length of the arrayand the width. Implement a function that draws a horizontal line from (xl, y) to (x2, y).The method signature should look something like:drawLine(byte[] screen, int width, int xl, int x2, int y)arrow_forwardProblem 3. This problem is about the same file foo as in the previous problem, but before it is modified. A program opens it for reading and gets back the file descriptor number fd. The program has a character array char buf [30]. The program now does the following: if ((rv = read (fd, buf,20)) < 0) { ...handle error... } if ((rv = read(fd,buf,20)) < 0) { ...handle error...} a. What does each call return (assuming no errors)? b. What do the first 20 bytes of the character array buf contain after the second read call? c. What do the last 10 bytes of buf contain?arrow_forwardTo do term Program 5: Improve the file copy program from Lab 9 so that it will make sure the user enters a file that exists. If a file doesn’t exist, the open function will raise the FileNotFoundError. You do not need to check if the destination exists since a file doesn’t need to exist to write it. Instead of copying the content of the source as exact, it only copies non-empty lines and it also prepend each line with a line number. A line is empty if the length of the line stripped of white space from both end is 0. The code segment below shows how to strip white space and getting the length of the string. If the length of the line is zero, the line is a blank line. line1 = " " line2 = " aa " print(len(line1.strip())) # 0 print(len(line2.strip())) # 2 In the sample run, “add.py” is the source file and “add-copy.py” is the destination file. Note that “add-copy.py” contains only non-blank lines from “add.py” and each line in “add-copy.py” is labelled with a line number. Sample run:…arrow_forward
- with python do whis: Edit or delete a user profileWhen the user chooses 2, the first thing that it should do is to check whether the user information is loaded in the program (i.e., check if the user information is passed to the function that generates recipe recommendations). If the user information is passed to the function (i.e., the user chose option 1 before choosing option 2), the program should show the user the following menu:Hello (user name)You can perform one of the following operations:1) Delete your profile2) Edit your profilea. If the user chooses 1, perform the following subtasks to delete a user profile:1- Search for the user profile in the file userInformation.txt using the user name in read mode; once you find the user profile (i.e., the line that contains all the user information), pass it to a function that deletes the user information.2- The function should create a temporary file called temp.txt in write mode and search the file userInformation.txt in read mode…arrow_forwardPlease design the mapper and reducer for the below WordCount problem. WordCount problem: Given an input text file, count the frequency of each word in the file. Please design the input and output pairs for the Mapper and Reducer, use below table for reference. Input Output We get the "WordCount.jar" file by using the above table. It only contains Mapper and Reducer. There is no Combiner and no Partitioner in this program. Please illustrate how the WordCount.jar program works on the below dataset. This text file only contains one line. Dataset: the sound sounds sound. it is the right right, right? Suppose we run the WordCount.jar program on this file. Suppose that the space symbol, and "?" are all used for separating the words. The outputs of the Mapper are Mapper The outputs of the reducer are Reducer Answer: Are the results sorted alphabetically? When was it sorted? 2 27 20 35arrow_forwardWrite a python program that will open a file and place each line of text from the file into a Python list. This program will calculate total number of lines, words and characters in the file. Write a python function counter to calculate and return total number of lines, words and characters in a text (.txt) file. The count function needs to return total number of lines, words and characters from the function. Also the function will take two parameters: • fileName is the name of the file that need to be opened to calculate the file stats (# of lines, # of words and # of characters). • lineList – this is python list that contains that will add each line (strip of new line feed in the line) to the python list. #function count will open the file fileName and counts how many lines, words and chars # in the file, and place each line of the file in the lineList parameter. # The function must RETURN numOfLine, numOfWords, numOfChars as return value, # and lineList as parameter # # numOfLine:…arrow_forward
- Please take your time and read the question very carefully also take a good look at my code in the link so that you can understand what you're working with. Like to full code for reference will you will need it Questions / what I need help with below link https://onlinegdb.com/jYuB3gpCxarrow_forwardcan you do it pythonarrow_forwardhow do i do this? pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
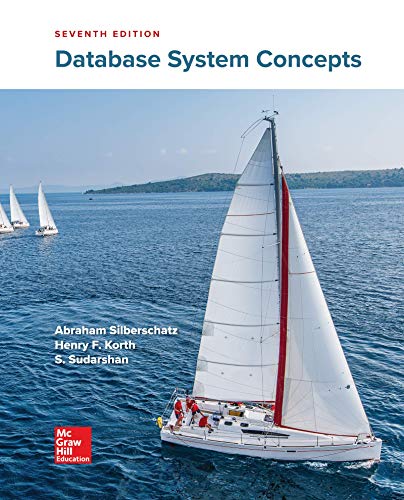
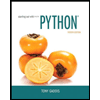
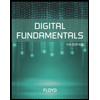
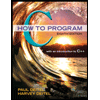
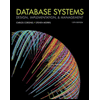
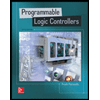