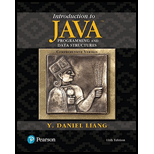
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13, Problem 13.15PE
Program Plan Intro
BigInteger.Java
Program Plan:
- Include a class name named “Exercise_13_15”.
- Declare package.
- Declare main method.
- Declare variables to create and initialize two rational numbers “r1” and “r2” using BigInteger() method.
- Print addition of two rational numbers.
- Print subtraction of two rational numbers.
- Print multiply of two rational numbers.
- Print division of two rational numbers.
- Print double value of rational number.
- Close the main method.
- Close the class.
- Include a class name named “Rational”.
- Declare package.
- Declare main method.
- Create Data fields for numerator and denominator.
- Create a rational constructor with default properties.
- Create a rational constructor and assign numerator and denominator.
- Declare variable gcd.
- Compare the denominator with 0 and use of ternary operator.
- Define a method to find GCD of two numbers.
- Declare variable n1 and n2.
- Compare n1 and n2 using the comapreTo() method.
- return the gcd of n1 and n2.
- Define a method to return numerator.
- Define a method to return denominator.
- Define a method to add a rational number to this rational.
- Add rational number.
- Declare a method to subtract a rational number from this rational.
- Declare a method to multiply a rational number by this rational.
- Declare a method to divide a rational number by this rational.
- Declare an override method to implement the abstract intValue() method in Number.
- Declare an override method to implement the abstract floatValue() method in Number.
- Declare an override method to implement the doubleValue() method in number.
- Declare an override method to implement the abstract longValue() method in number.
- Declare an override method to implement the compareTo method in Comparable
- Close the main method.
- Close the class.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
X15: inOrder
Write a function in Java that implements the following logic: Given three ints, a, b , and c,return true if b is greater than a, and
c is greater than b.However, with the exception that if bok is true, b does not need to be greater than a.
Part 2 - OddOrEven ClassIn bluej. Write a program that prompts the user to enter an integer. The program should display “The input is odd" to the screen if the input is odd and displays “The input is even" to the screen if the input is even. Hint: Consider using the mod (%) operator.
Do not use regular expressions in your solution for this exercise. Write a program that checks to see if an input password is valid or not. In this exercise, a valid password has the following characteristics:
It contains at least eight characters.
It contains at most 12 characters.
It contains only alphabetic characters, digits and the underscore (‘_’) character.
It does not start with a digit.
It has a mix of upper-case and lower-case characters.
Your class must contain the following method: public static String checkPassword(String input)
This method receives a possible password as input and returns only the String“OK” if the password is valid. If the password is not valid then you can return the reason(s) for that as the return value. This is not a part of the requirements, however, and you don’t need to do that. You can simply return null for an invalid password.
Chapter 13 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 13.2 - Prob. 13.2.1CPCh. 13.2 - The getArea() and getPerimeter() methods may be...Ch. 13.2 - True or false? a.An abstract class can be used...Ch. 13.3 - Prob. 13.3.1CPCh. 13.3 - Prob. 13.3.2CPCh. 13.3 - Prob. 13.3.3CPCh. 13.3 - What is wrong in the following code? (Note the...Ch. 13.3 - What is wrong in the following code? public class...Ch. 13.4 - Can you create a Calendar object using the...Ch. 13.4 - Prob. 13.4.2CP
Ch. 13.4 - How do you create a Calendar object for the...Ch. 13.4 - For a Calendar object c, how do you get its year,...Ch. 13.5 - Prob. 13.5.1CPCh. 13.5 - Prob. 13.5.2CPCh. 13.5 - Prob. 13.5.3CPCh. 13.5 - Prob. 13.5.4CPCh. 13.6 - Prob. 13.6.1CPCh. 13.6 - Prob. 13.6.2CPCh. 13.6 - Can the following code be compiled? Why? Integer...Ch. 13.6 - Prob. 13.6.4CPCh. 13.6 - What is wrong in the following code? public class...Ch. 13.6 - Prob. 13.6.6CPCh. 13.6 - Listing 13.5 has an error. If you add list.add...Ch. 13.7 - Can a class invoke the super.clone() when...Ch. 13.7 - Prob. 13.7.2CPCh. 13.7 - Show the output of the following code:...Ch. 13.7 - Prob. 13.7.4CPCh. 13.7 - What is wrong in the following code? public class...Ch. 13.7 - Show the output of the following code: public...Ch. 13.8 - Prob. 13.8.1CPCh. 13.8 - Prob. 13.8.2CPCh. 13.8 - Prob. 13.8.3CPCh. 13.9 - Show the output of the following code: Rational r1...Ch. 13.9 - Prob. 13.9.2CPCh. 13.9 - Prob. 13.9.3CPCh. 13.9 - Simplify the code in lines 8285 in Listing 13.13...Ch. 13.9 - Prob. 13.9.5CPCh. 13.9 - The preceding question shows a bug in the toString...Ch. 13.10 - Describe class-design guidelines.Ch. 13 - (Triangle class) Design a new Triangle class that...Ch. 13 - (Shuffle ArrayList) Write the following method...Ch. 13 - (Sort ArrayList) Write the following method that...Ch. 13 - (Display calendars) Rewrite the PrintCalendar...Ch. 13 - (Enable GeometricObject comparable) Modify the...Ch. 13 - Prob. 13.6PECh. 13 - (The Colorable interface) Design an interface...Ch. 13 - (Revise the MyStack class) Rewrite the MyStack...Ch. 13 - Prob. 13.9PECh. 13 - Prob. 13.10PECh. 13 - (The Octagon class) Write a class named Octagon...Ch. 13 - Prob. 13.12PECh. 13 - Prob. 13.13PECh. 13 - (Demonstrate the benefits of encapsulation)...Ch. 13 - Prob. 13.15PECh. 13 - (Math: The Complex class) A complex number is a...Ch. 13 - (Use the Rational class) Write a program that...Ch. 13 - (Convert decimals to fractious) Write a program...Ch. 13 - (Algebra: solve quadratic equations) Rewrite...Ch. 13 - (Algebra: vertex form equations) The equation of a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- X3: inlTo10 Write a function in Java that implements the following logic: Given a number n, return true if n is in the range 1..10, inclusive. Unless "outsideMode" is true, in which case return true if the number is less or equal to 1, or greater or equal to 10.arrow_forward:Write some statements that display a list of integers from 10 to 20 inclusive ,each with its square root next to it. Write a single statement to find and display the sum of the successive even integers 2, 4, ..., 200. (Answer: 10 100) Ten students in a class write a test. The marks are out of 10. All the marks are entered in a MATLAB vector marks. Write a statement to find and display the average mark. Try it on the following marks: 580 10 3 85794 (Answer: 5.9)arrow_forwardPart 2 - OddOrEven ClassWrite a program that prompts the user to enter an integer. The program should display “The input is odd" to the screen if the input is odd and displays “The input is even" to the screen if the input is even. Hint: Consider using the mod (%) operatorarrow_forward
- Please write the following Code..arrow_forwardQ3: (Tax Calculator) Develop a Java program that determines the total tax for each of four citizens. The tax rate is 10% for earnings up to 50,000 RM earned by each citizen and 15% for all earnings in excess of that ceiling. You are given a list with the citizens’ names and their earnings in a given year. Your program should input this information for each citizen, then determine and display the citizen’s total tax. Use class Scanner to input the data.arrow_forward(language: Intellij-Java) Part One: Create a method which takes two int parameters and swaps them. Print the values before and after swapping. (Example: ‘before swap: x = 5, y = 10; after swap: x=10, y=5). Part2: Write a method which gets a value from the user and convert that value from Celsius to Fahrenheit: - For Celsius to Fahrenheit conversion use: F = 9*C/5 + 32 - Print the result of the conversion Part3: Create a method which takes an integer array input from the user and reverses the elements in the array. Print the reversed array. - For example, the expected “reversed” result for an array [5,33,0,8,2] is [2,8,0,33,5] - Don't use any inbuilt/existing array reverse method. Try to write the reversing logicarrow_forward
- Please help me with this , I am stuck ! PLEASE WRITE IT IN C++ Thanks 1) bagUnion: The union of two bags is a new bag containing the combined contents of the original two bags. Design and specify a method union for the ArrayBag that returns as a new bag the union of the bag receiving the call to the method and the bag that is the method's parameter. The method signature (which should appear in the .h file and be implemented in the .cpp file is: ArrayBag<T> bagUnion(const ArrayBag<T> &otherBag) const; This method would be called in main() with: ArrayBag<int> bag1u2 = bag1.bagUnion(bag2); Note that the union of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the union of these bags contains x seven times. The union does not affect the contents of the original bags. Here is the main file: #include <cstdlib>#include <iostream>#include "ArrayBag.h"using namespace std; template…arrow_forwardRun the following code. Write its output and also explain the outputarrow_forwardQ1) Write a method that checks whether two words are anagrams. Two words are anagrams if they contain the same letters in any order. For example, "silent" and "listen" are anagrams. Write a test program that prompts the user to enter two strings and, if they are anagrams, displays "anagram", otherwise displays "not anagram". Note: The header of the method is as follows: public static boolean isAnagram(String s1, String s2) Sample Input #1: Enter two strings: Silent listen Sample Output #1: The string “Silent” and “listen” are anagrams. Sample Input #1: Enter two strings: teach peach Sample Output #1: The string “teach” and “peach” aren’t anagrams.arrow_forward
- (Check password) Some websites impose certain rules for passwords. Write a method that checks whether a string is a valid password. Suppose the password rules are as follows: A password must have at least eight characters. A password consists of only letters and digits. A password must contain at least two digits. Write a program that prompts the user to enter a password and displays Valid Password if the rules are followed or Invalid Password otherwise.arrow_forward(In Java) In the game Clacker, the numbers 1 through 12 are initially displayed. The player throws two dice and may cover the number representing the total or the two numbers on the dice. For example, for a throw of 3 and 5, the player may cover the 3 and the 5 or just the 8. Play continues until all the numbers are covered. The goal is to cover all numbers in the fewest rolls. Create a Clacker application that displays 12 buttons numbered 1 through 12. Each of these buttons can be clicked to ‘cover’ it. A covered button displays nothing. Another button labelled Roll can be clicked to roll the dice. Include labels to display the appropriate die images for each roll. Another label displays the number of rolls taken. A New Game button can be clicked to clear the labels and uncover the buttons for a new game.arrow_forward//Todo write test cases for SimpleCalculator Class // No need to implement the actual Calculator class just write Test cases as per TDD. // you need to just write test cases no mocking // test should cover all methods from calculator and all scenarios, so a minimum of 5 test // 1 for add, 1 for subtract, 1 for multiply, 2 for divide (1 for normal division, 1 for division by 0) // make sure all these test cases fail public class CalculatorTest { //Declare variable here private Calculator calculator; //Add before each here //write test cases here }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
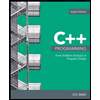
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning