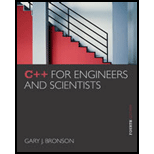
C++ for Engineers and Scientists
4th Edition
ISBN: 9781133187844
Author: Bronson, Gary J.
Publisher: Course Technology Ptr
expand_more
expand_more
format_list_bulleted
Question
Chapter 11, Problem 1PP
a)
Program Plan Intro
To construct the class Pol_coord along with the member functions convToPolar() and showdata().
a)
Expert Solution

Explanation of Solution
//class definition classPol_coord { //two floating point data members dist and mathematical private: floatdist; float theta; //constructor and function prototypes public: Pol_coord(); voidshowdata(); voidconvToPolar(float,float); }; //constructor function Pol_coord::Pol_coord() { //setting the values to 0 dist=0; theta=0; } //function for finding the polar coordinates voidPol_coord::convToPolar(float x,float y) { dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416)); } //function for showing the data voidPol_coord::showdata() { cout<<"The polar coordinates are "; cout<<dist<<", "<<theta<<endl; return; }
Explanation:
Create the class Pol_coordwith two floating data members for distance and angle. The constructor is used for assigning these two values.The function convToPolar() is used for converting them into polar coordinates using the following formula:
dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416));
b)
Program Plan Intro
- Include the class declaration and implementation in the program.
- Create the objects of the class Pol_coordin the main function.
- Calculate the polar coordinates using the method convToPolar.
- Display the data member values using the function showValues.
Program Description: The purpose of the program is to find the polar coordinate values.
b)
Expert Solution

Explanation of Solution
Program:
#include<iostream> //including the header file for mathematical functions #include<cmath> usingnamespacestd; //class definition classPol_coord { //two floating point data members dist and mathematical private: floatdist; float theta; //constructor and function prototypes public: Pol_coord(); voidshowdata(); voidconvToPolar(float,float); }; //constructor function Pol_coord::Pol_coord() { //setting the values to 0 dist=0; theta=0; } //function for finding the polar coordinates voidPol_coord::convToPolar(float x,float y) { dist=sqrt(pow(x,2)+pow(y,2)); theta=(atan(y/x)*(180/3.1416)); } //function for showing the data voidPol_coord::showdata() { cout<<"The polar coordinates are "; cout<<dist<<", "<<theta<<endl; return; } intmain() { Pol_coord a; a.convToPolar(9.09326,5.25001); a.showdata(); return0; }
Explanation:
The object of the class Pol_coord is created and the function convToPolar ( float, float ) is called with float values and then function showdata() is called for displaying the polar coordinates in the output screen.
Output Screenshot:
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
(C++) 23. True/False: function getGallons() is an inline member function
24. Design a class called Date.
The class should store a date in three private integers: month, day, year.
There should be a member function to print out the date in the month/day/year format, e.g. 12/25/2015, 4/1/1970
There should be a constructor which takes three parameters to initialize the date. It should not accept values for day greater than 31 or less than 1, or values for month greater than 12 or less than 1. If the constructor is called with these values an error message should be printed out and the field set to 0. Any value for year is acceptable.
There do not need to be any other member functions.
Provide the class declaration and also the implementation of the member function and constructor.
(C++ programming language)
Create a class Student with following data members- name, roll no and marks as private data member. Create array of objects for three students, compare their marks using operator overloading, and display the records of the student who is getting highest score.
(C++)
A designer in 3D graphics company wants to design a matrix as a two-dimensional array. The size of 2D array could be the last two digit of arid number. Initially he creates a class matrix that provides the member function to check that no array index is out of bounds. Make the member data in the matrix class a 10-by-10 array. A constructor should allow the programmer to specify the actual dimensions of the matrix (provided they’re less than 10 by 10). The member functions that access data in the matrix will now need two index numbers: one for each dimension of the array. Here’s what a fragment of a main() program that operates on such a class might look like:
If my Arid Number is 20-Arid-254 then: // in case of zero consider next digit
matrix m1(5, 4); // define a matrix object
int temp = 12345; // define an int value
m1.putel(7, 4, temp); // insert value of temp into matrix at 7,4
temp = m1.getel(7, 4); // obtain value from matrix at 7,4
Chapter 11 Solutions
C++ for Engineers and Scientists
Knowledge Booster
Similar questions
- (Hospital Class) Write a c++ class called 'Hospital' with Data Members: hospital name -string type- //store the name of the hospital Number of beds - integer type- //store number of beds in the hospital number of patient - integer type- // number of patients, consider each patient need one bed, if the hospital has 15 beds then no more than 15 patients Member Functions: Hospital(name, beds, patients) // Constructor to initilize data members addPatient() //to add one patient to number of patients currently in the hospital, just increment number of patient by 1 removePatient() //to remove one patient from number of patients currently in the hospital, just subtract one from number of patients displayInfo() // which just show information about the hospital: hospital name, number of beds, number of patients. Create a program that creates at least three Hospital objects or array of Hospital objects and tests the member functions of…arrow_forward(C++) Create a class Cylinder with attributes base-radius and height, each of which defaults to 1 .Provide member functions that calculate the surface area ( = 2πrh) and the volume (= πr2h) of the cylinder. Also, provide set and get functions for the base radius and height attributes. The set functions should verify that base radius and height are each floating- point numbers larger than 0.0 and less than 20.0. Use M PI from < cmath > header for π.arrow_forward(Polymorphic Banking Program Using Account Hierarchy) Develop a polymorphic banking program using the Account hierarchy created in Exercise 19.10. Create a vector of Accountpointers to SavingsAccount and CheckingAccount objects. For each Account in the vector, allowthe user to specify an amount of money to withdraw from the Account using member function debit and an amount of money to deposit into the Account using member function credit. As youprocess each Account, determine its type. If an Account is a SavingsAccount, calculate the amountof interest owed to the Account using member function calculateInterest, then add the interestto the account balance using member function credit. After processing an Account, print the updated account balance obtained by invoking base-class member function getBalance.arrow_forward
- (Python) (The Fan class) Design a class named Fan to represent a fan. The class contains: Three constants named SLOW, MEDIUM, and FAST with the values 1, 2, and 3 to denote the fan speed. A private int data field named speed that specifies the speed of the fan. A private bool data field named on that specifies whether the fan is on (the default is False). A private float data field named radius that specifies the radius of the fan. A private string data field named color that specifies the color of the fan. The accessor and mutator methods for all four data fields. A constructor that creates a fan with the specified speed (default SLOW), radius (default 5), color (default blue), and on (default False). Draw the UML diagram for the class and then implement the class. Write a test program that creates two Fan objects. For the first object, assign the maximum speed, radius 10, color yellow, and turn it on. Assign medium speed, radius 5, color blue, and turn it off for the…arrow_forwardC programing (condition using pointers) Q1) Write a function called letter grade that has a type int input parameter called points andreturns through an output parameters gradepLetter and gradepNumber. The appropriate lettergrade matching is given in the table below. Return through a second output parameter(just_missedp) an indication of whether the student just missed the next higher grade (true for89, 79, 64 and so on).Prototype: void letter_grade(int points, char *gradepLetter, char*gradepNumber, char *just_missedp);arrow_forward(Write C++ Code) 1. Monitor class header file: Monitor.h Instructions:1. Code the class specification file and name it as Monitor.h. You can code the constructor initialization steps in-line. The rest of the member functions should just be prototypes. The class specification should define the prototypes of each functions. Class name: MonitorPrivate Member Attributes: string brand string model int width (px) int height(px) int ppi double refreshRate (hz) char speakers (Y/N) int ports (no. of ports) string portType (list of port types separated by commas) Public Member Inline Functions Default Constructor with no parameters will initialize all numeric variables to 0. Constructor that accepts 3 int parameters for width, height, ppi. This constructor defaults the brand to "Generic", model to "Basic", the refreshRate to 60, speakers to 'N', ports to 1, and portType to "HDMI". Destructor (empty destructor) Public Member Functions as Prototypes: Prototype for…arrow_forward
- (Rectangle Class) Create a class Rectangle with attributes length and width, each of whichdefaults to 1. Provide member functions that calculate the perimeter and the area of the rectangle.Also, provide set and get functions for the length and width attributes. The set functions should verify that length and width are each floating-point numbers larger than 0.0 and less than 20.0.arrow_forward(C PROGRAMMING ONLY) 3. From Person to Peopleby CodeChum Admin Now that we have created a Person, it's time to create more Person and this tech universe shall be filled with people! Instructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main() function, there's a pre-created array of 5 Persons.Your task is to ask the user for the values of the age and gender of these Persons.Then, once you've set their ages and genders, call the displayPerson() function and pass them one by one.Input 1. A series of ages and genders of the 5 Persons Output Person #1Enter Person's age: 24Enter Person's gender: M Person #2Enter Person's·age: 21Enter Person's gender: F Person #3Enter Person's age: 22Enter Person's gender: F Person #4Enter Person's age: 60Enter…arrow_forward(phyton) create a vehicle claas with max_speed and mileage. define a function that changes the max speed with the setMax_speed(new speed) function. Create an object of this class change the max_speed value of this object to 200 with the help of the function you definedarrow_forward
- (C++) True/False: a class may have more than one destructor. True/False: assume a class has a default constructor implemented. Any time an object of this class is instantiated, a constructor is called. Public members of an object may be accessed using the following operator: a) . b) > c) - d) None of these A header file (.h file) of a class typically contains: a) The code to implement the class b) a program using the class c) The class declaration d) None of the abovearrow_forward(CP6) Write a function that is passed an array of structs and performs calculations. The function should locate the highest gpa from a group of students. The function is passed the array of structs, the first element to be searched, and the last element to be searched. The return value of the function is the highest gpa in the search range. struct data{ string name; double gpa;}; double highestGpa( data [ ], int, int ); data [ ] - array of structs to search int - first element to be searched int - last element to be searched return - highest gpa from grouparrow_forward(Dynamic Binding vs. Static Binding) Distinguish between static binding and dynamicbinding. Explain the use of virtual functions and the vtable in dynamic bindingarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
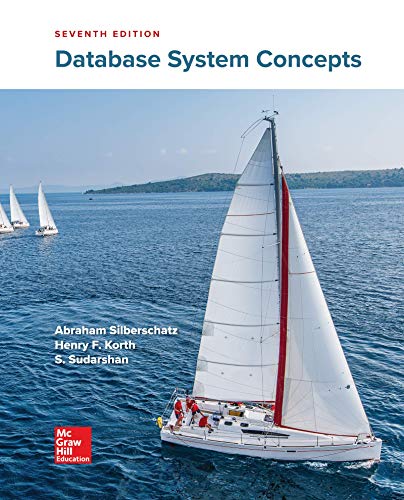
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
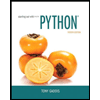
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
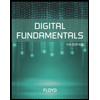
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
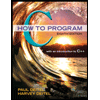
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
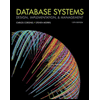
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
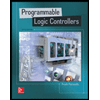
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education