This is the question I am struggling with - Create a class in JobApplicant.java that holds data about a job applicant. Include a name, a phone number, and four Boolean fields that represent whether the applicant is skilled in each of the following areas: word processing, spreadsheets, databases, and graphics. Include a constructor that accepts values for each of the fields. Also include a get method for each field. The get method should be the field name prefixed with 'get'. For example, the get method for name should be called getName. Create an application in TestJobApplicants.java that instantiates several job applicant objects and pass each in turn to a Boolean method named isQualified that determines whether each applicant is qualified for an interview. Then, in the main() method, display an appropriate method for each applicant. A qualified applicant has at least three of the four skills. This is the code it has given - public class JobApplicant { private String name; private String phone; private boolean hasWordSkill; private boolean hasSpreadsheetSkill; private boolean hasDatabaseSkill; private boolean hasGraphicsSkill; public JobApplicant(String name, String phone, boolean w, boolean s, boolean d, boolean g) { } public String getName() { } public String getPhone() { } public boolean getHasWordSkill() { } public boolean getHasSpreadsheetSkill() { } public boolean getHasDatabaseSkill() { } public boolean getHasGraphicsSkill() { } } import java.util.Scanner; public class TestJobApplicants { public static void main(String[] args) { JobApplicant app1 = new JobApplicant("Johnson", "312-345-9875", true, false, true, false); JobApplicant app2 = new JobApplicant("Kermit", "312-543-1275", true, false, false, false); JobApplicant app3 = new JobApplicant("Lawrence", "608-288-09125", true, false, true, true); JobApplicant app4 = new JobApplicant("Mitchell", "815-288-3881", true, true, true, true); String qualifiedMsg = "is qualified for an interview "; String notQualifiedMsg = "is not qualified for an interview at this time "; // Write code here } public static boolean isQualified(JobApplicant app) { // Write code here } public static void display(JobApplicant app, String msg) { System.out.println(app.getName() + " " + msg + " Phone: " + app.getPhone()); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
This is the question I am struggling with -
Create a class in JobApplicant.java that holds data about a job applicant. Include a name, a phone number, and four Boolean fields that represent whether the applicant is skilled in each of the following areas: word processing, spreadsheets,
Create an application in TestJobApplicants.java that instantiates several job applicant objects and pass each in turn to a Boolean method named isQualified that determines whether each applicant is qualified for an interview. Then, in the main() method, display an appropriate method for each applicant. A qualified applicant has at least three of the four skills.
This is the code it has given -

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

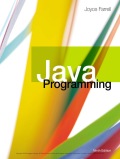
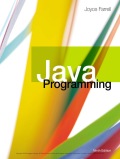