Class Circle A Circle will have a radius. The class will be able to keep track of the number of Circle objects created. It will also hold the total of the circumferences of all the Circle objects created. It will allow a client to create a Circle, passing in a double value for the radius into the Circle constructor. If the value for the radius that is passed in is 0 or negative, then the radius will be set to a value of 999. The constructor should add 1 to the count of the number of Circles created. The constructor should call a method to calculate the circumference and then add the
PLEASE SEE ATTACHED IMAGES FOR CLASS TRIANGLE AND CLASS CIRCLE
CLASS SHAPESDEMO IS THE DRIVER
PLEASE CODE IN JAVA AND SEE ALL SPECIFICATIONS
Problem Statement
Write a class called Triangle that can be used to represent a triangle.
Write a class called Circle that can be used to represent a circle.
Write a class called TriangleDemo that will interface with the Triangle and Circle classes.
Be sure to specify proper visibility for all methods and properties
Note 1:
When given 3 triangle sides:
- to determine if the triangle is right
1) Square all 3 sides.
2) Sum the squares of the 2 shortest sides.
3) Compare this sum to the square of the 3rd side.
4) If equal then we have a right triangle
Note 2:
All methods MUST be well documented including formalized pre-conditions and post-conditions. Refer to document T-1_Design By Contract located in the testing folder in your Project 1 download for guidance.
Note 3:
Your Triangle and Circle classes MUST be written according to these specifications. Do not vary from those specs.
CLASS SHAPESDEMO
- ShapesDemo will drive the application and manage the arrays which will store the Circle and Triangle objects. In main you will declare your Circle and Triangle arrays. You will need one array to store any Circle objects you create and another array to store any Triangle objects you create. You will also need to instantiate any other objects necessary necessary for input and output and declare any variables used in main.
- Review the Test Run to see how the application interfaces with the user.
- Inside a loop in main
- Prompt the user as shown below
- Allow the user to create either a Circle or a Triangle
- If the user selects Triangle then allow the user to type 3 integers on separate lines for each triangle.
- Instantiate each Triangle (refer to the sample dialog below for test data)
- Store each Triangle object in an array of Triangle objects.
- If the user selects Circle then allow the user to type in a double, representing the radius of the Circle.
- Instantiate each Circle (refer to the sample dialog below for test data)
- Store each Circle object in an array of Circle objects.
ï Once you have entered you data, then inside a separate loop
- Use an if statements to test each triangle for its properties and print appropriate output.
- Print the values of the sides of each triangle.
- Print the properties that apply for each triangle. See the sample output.
- Print the Perimeter for each triangle.
- Inside a separate loop
- Test each Circle for its properties and print appropriate output.
- Print the values as shown in the Test Run.
Test Run (Data entered by the user is in boldface)
Do you wish to create a circle or a triangle?
Enter 1 for a circle or 2 for a triangle
1. Circle
2. Triangle
1
Enter the radius for circle 1 as a double:
2.2
Do you wish to process another shape
y
Do you wish to create a circle or a triangle?
Enter 1 for a circle or 2 for a triangle
1. Circle
2. Triangle
1
Enter the radius for circle 2 as a double:
2.2
Do you wish to process another shape
y
Do you wish to create a circle or a triangle?
Enter 1 for a circle or 2 for a triangle
1. Circle
2. Triangle
2
Enter an integer dimension for side a of triangle 1: 3
Enter an integer dimension for side b of triangle 1: 4
Enter an integer dimension for side c of triangle 1: 5
Do you wish to process another shape
y
Properties for all Triangles created
Triangle 1 has sides of:
Side a: 3
Side b: 4
Side c: 5
The perimeter is 12
Triangle properties:
Right Triangle
Scalene
Triangle 2 has sides of:
Side a: 3
Side b: 3
Side c: 3
The perimeter is 9
Triangle properties:
Acute
Equilateral
The total perimeter of all 9 triangles is: 129
My test for equals method
Triangle 4 and 5 are equal
Triangle 2 and 6 are not equal
Properties for all Circles created
Circle 1 has a radius of 2.2
Circle 1 has circumference of 13.82
Circle 1 has an area of 15.21
Circle 2 has a radius of 2.2
Circle 2 has circumference of 13.82
Circle 2 has an area of 15.21
Circles are congruent
These circles are similar



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

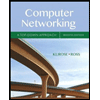
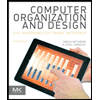
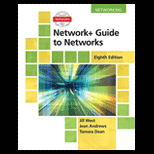
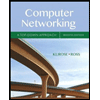
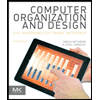
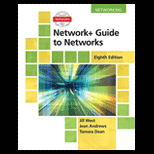
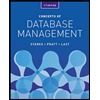
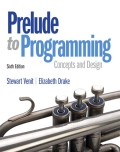
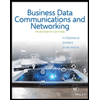