Put the class definition in House.h and the implementation of the constructors and functions in House.cpp 1. Define a class called "House", that represents the information of a house. A House is defined with these attributes: age (int), type (string) (for example Detached, Semi-Attached, Attached) and build_cost (double). Functions of the House class must perform the following operations: ✓ A default constructor which sets all the numeric instance variables to zero and the String instance variables to empty String. ✓ A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed. ✓ Implement a getter function for each of the 3 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable type must be called getType(). ✓ Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable type must be called setType(). ✓ An Estimate Price function: This function estimates the price of a house based on the build_cost and age. The house price is increased by 1% of the build_cost yearly. For example if the build_cost for a house is 100000 and the age of this house is 2, the EstimatPrice() function returns 102000 (100000+2* (0.01*100000)) ✓ A Similar function: This function has one input parameter which is a pointer to a House object. This function compares two houses and it returns true if both of them have the same age and type. ✔ A ComparePrice function: This This function has one input parameter which is a pointer to a House object. This function compares the price of two houses (using the Estimate Price function) and returns a string based on the comparison results as follows: - If the price of the first house is higher, ComparePric function returns this string: House 1 is more expensive" - If the price of the second house is higher, ComparePric function returns this string: House 2 is more expensive"
Put the class definition in House.h and the implementation of the constructors and functions in House.cpp 1. Define a class called "House", that represents the information of a house. A House is defined with these attributes: age (int), type (string) (for example Detached, Semi-Attached, Attached) and build_cost (double). Functions of the House class must perform the following operations: ✓ A default constructor which sets all the numeric instance variables to zero and the String instance variables to empty String. ✓ A constructor with 3 parameters which sets the 3 instance variables to the corresponding values passed. ✓ Implement a getter function for each of the 3 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable type must be called getType(). ✓ Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable type must be called setType(). ✓ An Estimate Price function: This function estimates the price of a house based on the build_cost and age. The house price is increased by 1% of the build_cost yearly. For example if the build_cost for a house is 100000 and the age of this house is 2, the EstimatPrice() function returns 102000 (100000+2* (0.01*100000)) ✓ A Similar function: This function has one input parameter which is a pointer to a House object. This function compares two houses and it returns true if both of them have the same age and type. ✔ A ComparePrice function: This This function has one input parameter which is a pointer to a House object. This function compares the price of two houses (using the Estimate Price function) and returns a string based on the comparison results as follows: - If the price of the first house is higher, ComparePric function returns this string: House 1 is more expensive" - If the price of the second house is higher, ComparePric function returns this string: House 2 is more expensive"
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 7SA: Assume the definition of class foodType as given in Exercise 6. Answer the following questions? (1,...
Related questions
Question
100%

Transcribed Image Text:Put the class definition in House.h and the implementation of the
constructors and functions in House.cpp
1. Define a class called "House", that represents the information of a house. A House is defined with these
attributes: age (int), type (string) (for example Detached, Semi-Attached, Attached) and build_cost (double).
Functions of the House class must perform the following operations:
✓ A default constructor which sets all the numeric instance
variables to zero and the String instance variables to empty
String.
✓ A constructor with 3 parameters which sets the 3 instance
variables to the corresponding values passed.
✓ Implement a getter function for each of the 3 instance variables
that will return the value of the instance variable. For example,
the getX() function for the instance variable type must be called
getType().
✔ Implement a setter function for each instance variable that will
assign to the instance variable to the value passed. For example,
the setX() function for the instance variable type must be called
setType().
✔ An EstimatePrice function: This function estimates the price of
a house based on the build_cost and age. The house price is
increased by 1% of the build_cost yearly. For example if the
build_cost for a house is 100000 and the age of this house is 2,
the EstimatPrice() function returns 102000 (100000+2*
(0.01*100000))
✓ A Similar function: This function has one input parameter which
is a pointer to a House object. This function compares two
houses and it returns true if both of them have the same age and
type.
✓ A ComparePrice function: This This function has one input
parameter which is a pointer to a House object. This function
compares the price of two houses (using the Estimate Price
function) and returns a string based on the comparison results as
follows:
- If the price of the first house is higher, ComparePric
function returns this string: House 1 is more expensive"
- If the price of the second house is higher, ComparePric
function returns this string: House 2 is more expensive"

Transcribed Image Text:function returns this string: House 1 is more expensive"
- If the price of the second house is higher, ComparePric
function returns this string: House 2 is more expensive"
Driver Class (House_driver.cpp)
Create a file House_driver.cpp with the main function that asks the
user to enter the information of two Houses and it dynamically
creates two objects of type House. Then using EstimatePrice
function, it shows the price for both houses. Afterward by using
the Similar and ComparePrice functions, the following results are
displayed: (Text in green is user input)
What is the build cost of first house? 350000
Note
1: You
are to
expect What is the age? 2
a
perfect
user
who
will
What is the type? Semi-Attached
What the build cost of second house? 400000
Where is the type? Semi-Attached
always
enter What is the age? 2į
valid
values;
that is, The estimated price for both houses are: ¿
do not
verify
357000. ¿
408000
What is the build cost of first house? 100000
What is the type? Semi-Attached
What is the age? 2į
What the build cost of second house? 200000
What is the type? Attached ¿
What is the age? 3 į
The estimated price for both houses are: ¿
102000.
206000 ¿
validity of user input.
the
Note 2: Please note that you are supposed to use the pointers at different parts of the lab question. If you
usonififiagent methods, even if your program works you do not get any mark.
House 2 is more expensive
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
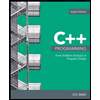
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
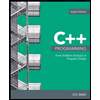
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning