C- Create the sub class UsedBook that is derived from the super class Book and has the following instance variables, constructor, and functions: title ( String) isbn ( String) authors (String) publisher (String) edition ( int) published_year (int) price_new (double) // the price of the book if it is new age (int) // how old the book is in years Constructor that takes all of the above variables as input parameters. set/get functions calculate_price method // the price is calculated as price_new * (1- 0.10*age) print function that prints all information related to the old book. D- Create the Testing class that has the main method and does the following: aa- Use the constructor to create instance of NewBook class and name it as new. Call compute_price function. bb- Use the constructor to create instance of usedBook class and name it used. Call compute_price function. cc- Print the related information of new and used objects using print functions. Use this following code: class Book: def__init__(self, title, isbn, authors, publisher, edition, published_year): self.title = title self.isbn = isbn self.authors = authors self.publisher = publisher self.edition = edition self.published_year = published_year # Setters defsetTitle(self,title): self.title = title defsetIsbn(self,isbn): self.isbn = isbn defsetAuthor(self,authors): self.authors = authors defsetPublisher(self, publisher): self.publisher = publisher defsetEdtion(self,edition): self.edition = edition defsetPublishedYear(self, published_year): self.published_year = published_year # Getters defgetTitle(self): returnself.title defgetIsbn(self): returnself.isbn defgetAuthor(self): returnself.authors defgetPublisher(self): returnself.publisher defgetEdtion(self): returnself.edition defgetPublishedYear(self): returnself.published_year defprint(self): print("Title: ",self.getTitle()) print("ISBN: ",self.getIsbn()) print("Authors: ",self.getAuthor()) print("Publisher: ",self.getPublisher()) print("Edition: ",self.getEdtion()) print("Published Year: ",self.getPublishedYear()) class NewBook(Book): def__init__(self, title, isbn, authors, publisher, edition, published_year, price): super().__init__(title, isbn, authors, publisher, edition, published_year) self.price = price defsetPrice(self,price): self.price = price defcalculate_price(self): returnself.price defprint(self): super().print() print("Price: ",self.calculate_price())
C- Create the sub class UsedBook that is derived from the super class Book and has the following instance variables, constructor, and functions:
title ( String)
isbn ( String)
authors (String)
publisher (String)
edition ( int)
published_year (int)
price_new (double) // the price of the book if it is new
age (int) // how old the book is in years
Constructor that takes all of the above variables as input parameters.
set/get functions
calculate_price method // the price is calculated as price_new * (1- 0.10*age)
print function that prints all information related to the old book.
D- Create the Testing class that has the main method and does the following:
aa- Use the constructor to create instance of NewBook class and name it as new. Call compute_price function.
bb- Use the constructor to create instance of usedBook class and name it used. Call compute_price function.
cc- Print the related information of new and used objects using print functions.
Use this following code:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

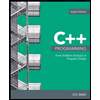
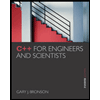
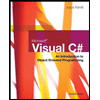
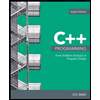
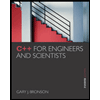
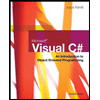
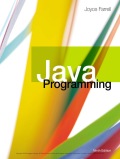