## MP (Machine Problem) Details The bulls and cows game is a code-breaking game designed for two or more players. Each player chooses a secret code of 4 digits from 0 – 9. The digits must be all different. The goal of the game is for each player to guess the other player's secret code. The players in turn present their guesses to the opponents. The opponents respond by telling the players: 1. The number of bulls, i.e. the number of matching digits in their right positions, and 2. The number of cows, i.e. the number of matching digits but in different positions. ### Requirements The main goal of this assignment is to develop the bulls and cows game that allows a single player to play interactively against the computer. The game stores two secret codes, one from the player and one from the computer. The player and the computer will try to guess each other’s secret code. Both the player and the computer only have seven attempts for guessing the secret code. If the player enters invalid input, the game should ask the player to try again. The game also lets the player choose the difficulty level to play against the computer. There are three levels: easy, medium, and hard. The details of the difficulty levels will be described in later sections. In addition, the game can read from a text file that contains multiple guesses from the player, and save the results to another text file. You don’t have to do this now, you will do this after the discussion on IO and File IO. For this module assignment, you'll complete a series of tasks as you work your way towards a fully functional implementation. As you complete each task, it might be a good idea to save a working version of the program at that point. ## Task One: Design and Feedback For this assignment, there is very little which is already given to you. Through this assignment, you'll gain experience in designing and building a complex program from scratch. Before starting to code, don't forget to design your FLOWCHART. You should apply the concepts you have learned so far in the course. Try to promote code reuse as much as possible by using methods. Using either pen & paper or any diagramming tool of your choice, I recommend that you use draw.io to create your FLOWCHART. Save this diagram (as a PNG or JPEG) within the module assignment folder. ## Task Two: The Beginning Implement the first part of the game allowing the player to guess the computer's secret code. The computer randomly generates the secret code at the beginning of the game, which it then lets the player guess. Remember that when generating the computer's secret code, each of the four digits must be different. Note that the player only has seven attempts to guess the secret code. The prompt for player input results for each guess and the final outcome (i.e. whether the player has won the game or not) should be displayed appropriately on the console. ## Task Three: Easy AI Save your Task 2 code on a different package / folder. Modify your code so the player can now also enter a secret code when the game begins, which the computer must guess. Remember to verify that the player has chosen a valid secret code. The player and computer each take turn guessing the other's code. The game ends when either side successfully guesses the other's code (resulting in a win for that side) or when each side has made seven incorrect guesses (resulting in a draw). For this task, have the player play against an easy AI. When the AI makes a guess, it will simply generate a random (valid) guess. ## Task Four: Medium AI Save your Task 3 code on a different package / folder. Modify your code so that at the beginning of the game (before the player enters their own secret code), they will be asked to select either an easy or medium AI opponent to play against. If the player chooses to play against an easy AI, the game should proceed in exactly the same manner as in Task Three. However, if a medium AI is selected, the AI should keep track of guesses it has already made. The AI will not make the same guess twice.
## MP (Machine Problem) Details The bulls and cows game is a code-breaking game designed for two or more players. Each player chooses a secret code of 4 digits from 0 – 9. The digits must be all different. The goal of the game is for each player to guess the other player's secret code. The players in turn present their guesses to the opponents. The opponents respond by telling the players: 1. The number of bulls, i.e. the number of matching digits in their right positions, and 2. The number of cows, i.e. the number of matching digits but in different positions. ### Requirements The main goal of this assignment is to develop the bulls and cows game that allows a single player to play interactively against the computer. The game stores two secret codes, one from the player and one from the computer. The player and the computer will try to guess each other’s secret code. Both the player and the computer only have seven attempts for guessing the secret code. If the player enters invalid input, the game should ask the player to try again. The game also lets the player choose the difficulty level to play against the computer. There are three levels: easy, medium, and hard. The details of the difficulty levels will be described in later sections. In addition, the game can read from a text file that contains multiple guesses from the player, and save the results to another text file. You don’t have to do this now, you will do this after the discussion on IO and File IO. For this module assignment, you'll complete a series of tasks as you work your way towards a fully functional implementation. As you complete each task, it might be a good idea to save a working version of the program at that point. ## Task One: Design and Feedback For this assignment, there is very little which is already given to you. Through this assignment, you'll gain experience in designing and building a complex program from scratch. Before starting to code, don't forget to design your FLOWCHART. You should apply the concepts you have learned so far in the course. Try to promote code reuse as much as possible by using methods. Using either pen & paper or any diagramming tool of your choice, I recommend that you use draw.io to create your FLOWCHART. Save this diagram (as a PNG or JPEG) within the module assignment folder. ## Task Two: The Beginning Implement the first part of the game allowing the player to guess the computer's secret code. The computer randomly generates the secret code at the beginning of the game, which it then lets the player guess. Remember that when generating the computer's secret code, each of the four digits must be different. Note that the player only has seven attempts to guess the secret code. The prompt for player input results for each guess and the final outcome (i.e. whether the player has won the game or not) should be displayed appropriately on the console. ## Task Three: Easy AI Save your Task 2 code on a different package / folder. Modify your code so the player can now also enter a secret code when the game begins, which the computer must guess. Remember to verify that the player has chosen a valid secret code. The player and computer each take turn guessing the other's code. The game ends when either side successfully guesses the other's code (resulting in a win for that side) or when each side has made seven incorrect guesses (resulting in a draw). For this task, have the player play against an easy AI. When the AI makes a guess, it will simply generate a random (valid) guess. ## Task Four: Medium AI Save your Task 3 code on a different package / folder. Modify your code so that at the beginning of the game (before the player enters their own secret code), they will be asked to select either an easy or medium AI opponent to play against. If the player chooses to play against an easy AI, the game should proceed in exactly the same manner as in Task Three. However, if a medium AI is selected, the AI should keep track of guesses it has already made. The AI will not make the same guess twice.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section: Chapter Questions
Problem 14PP
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
## MP (Machine Problem) Details
The bulls and cows game is a code-breaking game designed for two or more players. Each
player chooses a secret code of 4 digits from 0 – 9. The digits must be all different. The goal of
the game is for each player to guess the other player's secret code.
The players in turn present their guesses to the opponents. The opponents respond by telling
the players:
1. The number of bulls, i.e. the number of matching digits in their right positions, and
2. The number of cows, i.e. the number of matching digits but in different positions.
### Requirements
The main goal of this assignment is to develop the bulls and cows game that allows a single
player to play interactively against the computer. The game stores two secret codes, one from
the player and one from the computer. The player and the computer will try to guess each
other’s secret code. Both the player and the computer only have seven attempts for guessing
the secret code. If the player enters invalid input, the game should ask the player to try again.
The game also lets the player choose the difficulty level to play against the computer.
There are three levels: easy, medium, and hard. The details of the difficulty levels will be
described in later sections.
In addition, the game can read from a text file that contains multiple guesses from the player,
and save the results to another text file. You don’t have to do this now, you will do this after the
discussion on IO and File IO.
For this module assignment, you'll complete a series of tasks as you work your way towards a
fully functional implementation. As you complete each task, it might be a good idea to save a
working version of the program at that point.
## Task One: Design and Feedback
For this assignment, there is very little which is already given to you. Through this assignment,
you'll gain experience in designing and building a complex program from scratch. Before starting to code, don't forget to design your FLOWCHART. You should apply the concepts you
have learned so far in the course. Try to promote code reuse as much as possible by using
methods.
Using either pen & paper or any diagramming tool of your choice, I recommend that you use
draw.io to create your FLOWCHART. Save this
diagram (as a PNG or JPEG) within the module assignment folder.
## Task Two: The Beginning
Implement the first part of the game allowing the player to guess the computer's secret code.
The computer randomly generates the secret code at the beginning of the game, which it then
lets the player guess. Remember that when generating the computer's secret code, each of the
four digits must be different. Note that the player only has seven attempts to guess the secret
code. The prompt for player input results for each guess and the final outcome (i.e. whether the
player has won the game or not) should be displayed appropriately on the console.
## Task Three: Easy AI
Save your Task 2 code on a different package / folder.
Modify your code so the player can now also enter a secret code when the game begins, which
the computer must guess. Remember to verify that the player has chosen a valid secret code.
The player and computer each take turn guessing the other's code. The game ends when either
side successfully guesses the other's code (resulting in a win for that side) or when each side
has made seven incorrect guesses (resulting in a draw).
For this task, have the player play against an easy AI. When the AI makes a guess, it will simply
generate a random (valid) guess.
## Task Four: Medium AI
Save your Task 3 code on a different package / folder.
Modify your code so that at the beginning of the game (before the player enters their own
secret code), they will be asked to select either an easy or medium AI opponent to play against.
If the player chooses to play against an easy AI, the game should proceed in exactly the same
manner as in Task Three. However, if a medium AI is selected, the AI should keep track of
guesses it has already made. The AI will not make the same guess twice.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
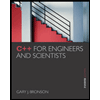
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
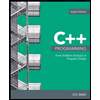
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
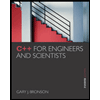
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
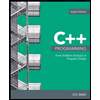
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning