In this lab and subsequent assignments, you will develop a web application for Hurricanes Soccer Academy to help them manage their players and teams. 1.1 Create a Spring Boot project as described in the lecture Introduction to Spring Boot and MVC Architecture ✓. Add two model classes, Player and Team, to the project. The Player class represents a player, and it should have at least the following properties: id, name, gender, birthYear, team (or teamId), as well as a read-only property age which is calculated from the player's birth year. The Team class represents a team, and it should have at least the following properties: id, uniformColor, gender (whether it's a boys or girls team), minAge and maxAge (age limit of the players who can be assigned to the team). 2. Use a singleton bean to store a List and a List. Add at least two Player objects to the player list and at least one Team object to the team list. 3. Use the Hurricanes Soccer Academy home page you created in Week 4 Lab as the home page of the application (i.e., the page shown at the root path of the application). Change the navbar menu so that it has three menu items: Home, Players, Teams. 4. When a user clicks on Players in the navbar menu, the application should show the players in a table with the following columns: Id, Name, Age, and Team (which shows team id). 5. The Players page should have a New Player button (or a link styled as a button). Clicking on this button should take the user to a page where the information of a new player can be entered into a form. The form should have at least the following fields: 6. • A text field for the players name. • A dropdown list from which the birth year of the player can be selected. The academy accepts kids of age 4 to 12, which means that the dropdown list should include years from (current year - 12) to (current year - 4). • Two radio buttons for male and female gender. • A Cancel button that takes the user back to the Players page. • An Add button that submits the form. After a new player is added, the application should redirect the user to the players page. All pages should have consistent styling using Bootstrap. In particular, all pages should have the same header and footer, and UI components such as tables, forms, and buttons should be properly styled with Bootstrap. You may see a video of what the application should look like here.
In this lab and subsequent assignments, you will develop a web application for Hurricanes Soccer Academy to help them manage their players and teams. 1.1 Create a Spring Boot project as described in the lecture Introduction to Spring Boot and MVC Architecture ✓. Add two model classes, Player and Team, to the project. The Player class represents a player, and it should have at least the following properties: id, name, gender, birthYear, team (or teamId), as well as a read-only property age which is calculated from the player's birth year. The Team class represents a team, and it should have at least the following properties: id, uniformColor, gender (whether it's a boys or girls team), minAge and maxAge (age limit of the players who can be assigned to the team). 2. Use a singleton bean to store a List and a List. Add at least two Player objects to the player list and at least one Team object to the team list. 3. Use the Hurricanes Soccer Academy home page you created in Week 4 Lab as the home page of the application (i.e., the page shown at the root path of the application). Change the navbar menu so that it has three menu items: Home, Players, Teams. 4. When a user clicks on Players in the navbar menu, the application should show the players in a table with the following columns: Id, Name, Age, and Team (which shows team id). 5. The Players page should have a New Player button (or a link styled as a button). Clicking on this button should take the user to a page where the information of a new player can be entered into a form. The form should have at least the following fields: 6. • A text field for the players name. • A dropdown list from which the birth year of the player can be selected. The academy accepts kids of age 4 to 12, which means that the dropdown list should include years from (current year - 12) to (current year - 4). • Two radio buttons for male and female gender. • A Cancel button that takes the user back to the Players page. • An Add button that submits the form. After a new player is added, the application should redirect the user to the players page. All pages should have consistent styling using Bootstrap. In particular, all pages should have the same header and footer, and UI components such as tables, forms, and buttons should be properly styled with Bootstrap. You may see a video of what the application should look like here.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Using Intellij IDEA (Using Spring intializer, language: java, type: Maven)

Transcribed Image Text:In this lab and subsequent assignments, you will develop a web application for Hurricanes Soccer Academy to help
them manage their players and teams.
1.1
Create a Spring Boot project as described in the lecture Introduction to Spring Boot and MVC Architecture
✓. Add two model classes, Player and Team, to the project. The Player class represents a player, and it should have at
least the following properties: id, name, gender, birthYear, team (or teamId), as well as a read-only property age
which is calculated from the player's birth year. The Team class represents a team, and it should have at least the
following properties: id, uniformColor, gender (whether it's a boys or girls team), minAge and maxAge (age limit of the
players who can be assigned to the team).
2.
Use a singleton bean to store a List<Player> and a List<Team>. Add at least two Player objects to the player
list and at least one Team object to the team list.
3.
Use the Hurricanes Soccer Academy home page you created in Week 4 Lab as the home page of the
application (i.e., the page shown at the root path of the application). Change the navbar menu so that it has three
menu items: Home, Players, Teams.
4.
When a user clicks on Players in the navbar menu, the application should show the players in a table with the
following columns: Id, Name, Age, and Team (which shows team id).
5.
The Players page should have a New Player button (or a link styled as a button). Clicking on this button
should take the user to a page where the information of a new player can be entered into a form. The form should
have at least the following fields:
6.
• A text field for the players name.
• A dropdown list from which the birth year of the player can be selected. The academy accepts kids of age 4 to 12,
which means that the dropdown list should include years from (current year - 12) to (current year - 4).
• Two radio buttons for male and female gender.
• A Cancel button that takes the user back to the Players page.
• An Add button that submits the form. After a new player is added, the application should redirect the user to the
players page.
All pages should have consistent styling using Bootstrap. In particular, all pages should have the same header
and footer, and UI components such as tables, forms, and buttons should be properly styled with Bootstrap. You may
see a video of what the application should look like here.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 1 steps

Recommended textbooks for you
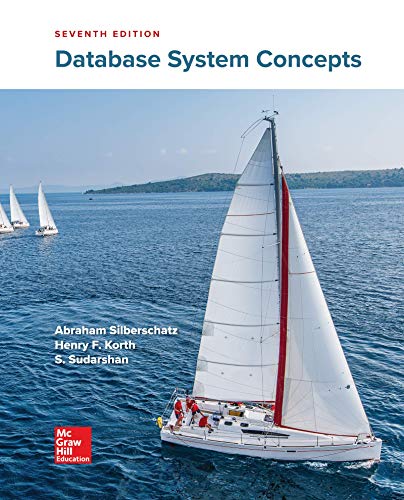
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
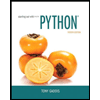
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
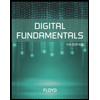
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
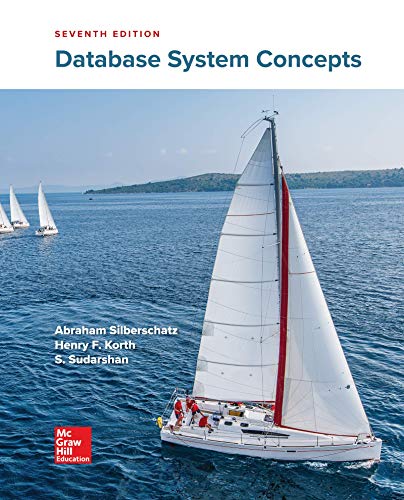
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
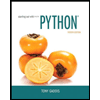
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
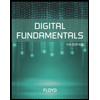
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
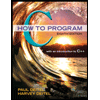
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
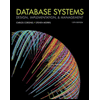
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
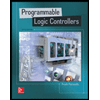
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education