import time def raiseIndexError(): raise IndexError def raiseZeroDivisionErrorWithMessage (message:str): raise ZeroDivisionError(message) def raise This Exception (exception): raise exception def catchAndReturnMessage (message: str, main_function: callable) →> str: try: main_function() except Exception as e: return str(e) else: return message def catchCleanupAnd Throw (main_supplier: callable, index_supplier: callable, zero_supplier: callable, cleanup: callable) -> str: try: result = main_supplier() except IndexError: result = index_supplier() except ZeroDivisionError: result = zero_supplier() finally: cleanup() return result class Timer: definit__(self, time_out:int): self.time_out= time_out self.start_time = None self.end_time = None def get_total_time(self): if self.end_time == None: return -1 return self.end_time- self.start_time def _enter_(self): self.start_time = int(round(time.time()* 1000)) return self def_exit_(self, exc_type, exc_value, traceback): self.end_time = time.time() if self.get_total_time() > self.time_out: raise TimeoutError
import time def raiseIndexError(): raise IndexError def raiseZeroDivisionErrorWithMessage (message:str): raise ZeroDivisionError(message) def raise This Exception (exception): raise exception def catchAndReturnMessage (message: str, main_function: callable) →> str: try: main_function() except Exception as e: return str(e) else: return message def catchCleanupAnd Throw (main_supplier: callable, index_supplier: callable, zero_supplier: callable, cleanup: callable) -> str: try: result = main_supplier() except IndexError: result = index_supplier() except ZeroDivisionError: result = zero_supplier() finally: cleanup() return result class Timer: definit__(self, time_out:int): self.time_out= time_out self.start_time = None self.end_time = None def get_total_time(self): if self.end_time == None: return -1 return self.end_time- self.start_time def _enter_(self): self.start_time = int(round(time.time()* 1000)) return self def_exit_(self, exc_type, exc_value, traceback): self.end_time = time.time() if self.get_total_time() > self.time_out: raise TimeoutError
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi help me fixing the error

Transcribed Image Text:import time
def raiseIndexError():
raise IndexError
def raiseZeroDivisionErrorWithMessage (message: str):
raise ZeroDivisionError(message)
def raise This Exception (exception):
raise exception
def catchAndReturnMessage (message:str, main_function: callable) →> str:
try:
main_function()
except Exception as e:
return str(e)
else:
return message
def catchCleanupAnd Throw (main_supplier:callable, index_supplier: callable, zero_supplier:callable, cleanup: callable) -> str:
try:
result = main_supplier()
except IndexError:
result = index_supplier()
except ZeroDivisionError:
result = zero_supplier()
finally:
cleanup()
return result
class Timer:
def __init__(self, time_out:int):
self.time_out = time_out
self.start_time = None
self.end_time = None
def get_total_time(self):
if self.end_time == None:
return -1
return self.end_time self.start_time
def _enter_(self):
self.start_time = int(round(time.time()* 1000))
return self
def _exit_(self, exc_type, exc_value, traceback):
self.end_time = time.time()
if self.get_total_time() > self.time_out:
raise TimeoutError

Transcribed Image Text:Test Timer
✪ Error
AssertionError
True is not false : The Timer context manager failed to raised a TimeoutError even
though the function completed after the time-out limit. The code appoximatly took 943
ms to exicute and the timeout time was 487 ms.
Traceback (most recent call last):
File "/var/gt_test_envs/7hDNGjWK9CdSx3ak/wrk/homeworkTest.py",
line 192, in test_Timer
self.assertFalse (timed_out, 'The Timer context manager failed to raised a TimeoutError
even though the function completed after the time-out limit. The code appoximatly took
%s ms to exicute and the timeout time was %s ms. %(sleep, time_out))
AssertionError: True is not false : The Timer context manager failed to raised a
TimeoutError even though the function completed after the time-out limit. The code
appoximatly took 943 ms to exicute and the timeout time was 487 ms.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
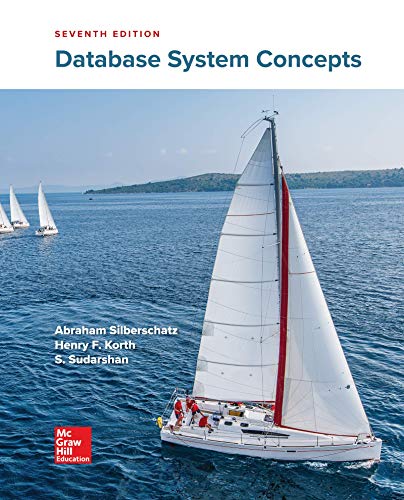
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
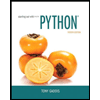
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
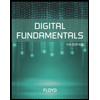
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
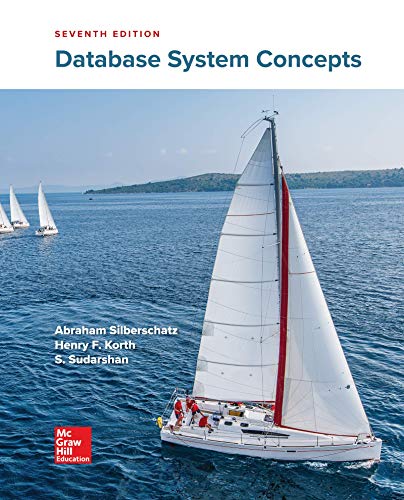
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
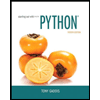
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
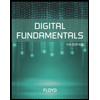
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
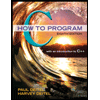
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
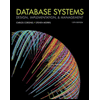
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
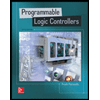
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education