I am trying to get this code to run and but the program calls an syntax error on the else if I remove the 'except EOFError:' code which I do not need. Is there another way I should be writing the loop? class Wages: #Declare Methods def __init__(self, name, hours, wages): self.name = name self.hours = hours self.wages = wages #Determine Name def getName(self): self.name = str(input("Enter employee's name: ")) return self.name #Validate Weekly Hours def getHours(self): while True: try: self.hours = int(input("Enter number of hours worked: ")) if self.hours <= 0: print("Value must be a number greater than 0!") continue except (EOFError, ValueError): print("Value must be a number and greater than 0!") continue else: return self.hours #Validte Hourly Wage def getWage(self): while True: try: self.wages = float(input("Enter your hourly wage: ")) if self.wages <= 0: print("Value must be a number greater than 0!") continue except EOFError: print("Value must be a number greater than 0!") continue else: return self.wages #Determine Weekly Pay def payForWeek(self): pay = float(wage) * int(hours) if int(hours) > 40: overtime = 40 - int(hours) pay += 0.5 * overtime return pay return pay cont = 'Y' while cont == 'Y': weekPay = Wages('john',45,100) print("This Program will create a class that stores an employees name and calculates weekly pay.") name = str(weekPay.getName()) hours = str(weekPay.getHours()) wage = str(weekPay.getWage()) weekpay = str(weekPay.payForWeek()) print("You entered that " + name + " worked " + hours + " hour(s) this week.") print("You entered that " + name + " earns $" + wage + " per hour.") print("Pay for " + name + " is $" + weekpay) print("Do you want to run the program again? ('Y' for yes, anything else to quit)") cont = input()
I am trying to get this code to run and but the program calls an syntax error on the else if I remove the 'except EOFError:' code which I do not need.
Is there another way I should be writing the loop?
class Wages:
#Declare Methods
def __init__(self, name, hours, wages):
self.name = name
self.hours = hours
self.wages = wages
#Determine Name
def getName(self):
self.name = str(input("Enter employee's name: "))
return self.name
#Validate Weekly Hours
def getHours(self):
while True:
try:
self.hours = int(input("Enter number of hours worked: "))
if self.hours <= 0:
print("Value must be a number greater than 0!")
continue
except (EOFError, ValueError):
print("Value must be a number and greater than 0!")
continue
else:
return self.hours
#Validte Hourly Wage
def getWage(self):
while True:
try:
self.wages = float(input("Enter your hourly wage: "))
if self.wages <= 0:
print("Value must be a number greater than 0!")
continue
except EOFError:
print("Value must be a number greater than 0!")
continue
else:
return self.wages
#Determine Weekly Pay
def payForWeek(self):
pay = float(wage) * int(hours)
if int(hours) > 40:
overtime = 40 - int(hours)
pay += 0.5 * overtime
return pay
return pay
cont = 'Y'
while cont == 'Y':
weekPay = Wages('john',45,100)
print("This Program will create a class that stores an employees name and calculates weekly pay.")
name = str(weekPay.getName())
hours = str(weekPay.getHours())
wage = str(weekPay.getWage())
weekpay = str(weekPay.payForWeek())
print("You entered that " + name + " worked " + hours + " hour(s) this week.")
print("You entered that " + name + " earns $" + wage + " per hour.")
print("Pay for " + name + " is $" + weekpay)
print("Do you want to run the program again? ('Y' for yes, anything else to quit)")
cont = input()

Step by step
Solved in 2 steps with 3 images

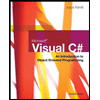
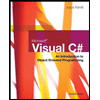