Functions for Organizing Your Code. You will create a new version of the program below . This new version will define the following functions. A function to read and validate the user option. A function to read and validate a yes/no input, if using the user-confirmation approach. A function that prints the menu A function that reads the order and returns the sub-total (which is the sum of the prices of the items ordered). A function that takes the sub-total as a parameter, and calculates and return the tax, the final total, and the tip. A function that takes the sub-tota, tax, total, and tip, and prints a receipt. Defiine function main() to drive the functionality of the program. Your program shall: Define and call the required functions Use meaningful names for the functions. Run and test the program to make sure it works correctly import sys menu = "Y" continueLoop ="y" #Enter number for each dish and price for users while continueLoop =="y": DishNumber= int(input("Enter numbers between 1 and 10:")) if DishNumber == 1: print("Cajun Calamari price is $9.00") elif DishNumber== 2: print("Shrimp Cocktail price is $9.00") elif DishNumber== 3: print:("Spicy Garlic Mussels price is $9.00") elif DishNumber== 4: print("Crispy Ahi price is $10.50") elif DishNumber== 5: print("Spinach Artichoke Dip price is $8.00") elif DishNumber== 6: print("Steamed Clams price is $10.00") elif DishNumber== 7: print("Chips and Dip price is $6.50") elif DishNumber== 8: print:("Beer Battered Ribs price is $6.00") elif DishNumber== 9: print("St.Louis Ribs price is $8.00") elif DishNumber== 10: print("Mozzarella Sticks price is $7.00") else: print("You have selected an invalid input.") continueLoop = input("Enter y to Continue or n to quit:") #Calculate tax, total, tip subtotal = prices + sales_tax sales_tax = subtotal*0.085 grand_total = subtotal + tip tip = subtotal*0.15 #Display information print("Subtotal: ',subtotal,'$") print("Sales tax : ','sales_tax,'$") print("Grand total : ',grand_total,'$") print("Suggested tip : ',tip,'$")
Functions for Organizing Your Code.
You will create a new version of the program below . This new version will define the following functions.
- A function to read and validate the user option.
- A function to read and validate a yes/no input, if using the user-confirmation approach.
- A function that prints the menu
- A function that reads the order and returns the sub-total (which is the sum of the prices of the items ordered).
- A function that takes the sub-total as a parameter, and calculates and return the tax, the final total, and the tip.
- A function that takes the sub-tota, tax, total, and tip, and prints a receipt.
- Defiine function main() to drive the functionality of the program.
Your program shall:
- Define and call the required functions
- Use meaningful names for the functions.
- Run and test the program to make sure it works correctly
import sys
menu = "Y"
continueLoop ="y"
#Enter number for each dish and price for users
while continueLoop =="y":
DishNumber= int(input("Enter numbers between 1 and 10:"))
if DishNumber == 1:
print("Cajun Calamari price is $9.00")
elif DishNumber== 2:
print("Shrimp Cocktail price is $9.00")
elif DishNumber== 3:
print:("Spicy Garlic Mussels price is $9.00")
elif DishNumber== 4:
print("Crispy Ahi price is $10.50")
elif DishNumber== 5:
print("Spinach Artichoke Dip price is $8.00")
elif DishNumber== 6:
print("Steamed Clams price is $10.00")
elif DishNumber== 7:
print("Chips and Dip price is $6.50")
elif DishNumber== 8:
print:("Beer Battered Ribs price is $6.00")
elif DishNumber== 9:
print("St.Louis Ribs price is $8.00")
elif DishNumber== 10:
print("Mozzarella Sticks price is $7.00")
else:
print("You have selected an invalid input.")
continueLoop = input("Enter y to Continue or n to quit:")
#Calculate tax, total, tip
subtotal = prices + sales_tax
sales_tax = subtotal*0.085
grand_total = subtotal + tip
tip = subtotal*0.15
#Display information
print("Subtotal: ',subtotal,'$")
print("Sales tax : ','sales_tax,'$")
print("Grand total : ',grand_total,'$")
print("Suggested tip : ',tip,'$")

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

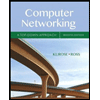
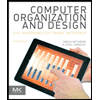
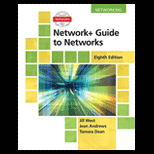
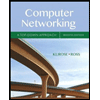
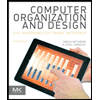
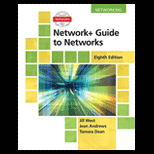
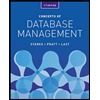
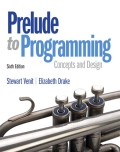
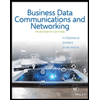