code Connect6State.java according to the following instructions Class Connect6State java.lang.Object Connect6State public class Connect6State extends java.lang.Object Connect6State - A representation of a Connect6 (http://en.wikipedia.org/wiki/Connect6) game state. We assume a square grid board size specified to be a positive integer less than or equal to 26. Size 19 is common. The first and second players place pieces on the board that are black and white, respectively. Each piece is placed in an unoccupied grid position. On the first turn, the first player places one piece. Afterwards, players alternate placing two pieces per turn. Play continues until either one player completes a consecutive line of 6 or more of their pieces or no play is possible. The player with 6 or more pieces in a horizontal, vertical, or diagonal line is the winner. If no legal play is possible, the game is a draw. Field Summary Fields Modifier and Type Field Description static int BLACK a constant int value 1 indicating a black player/piece static int NONE a constant int value 0 indicating no player/piece static int WHITE a constant int value -1 indicating a white player/piece Constructor Summary Constructors Constructor Description Connect6State(int size) Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26. Method Summary All MethodsInstance MethodsConcrete Methods Modifier and Type Method Description int getPiece(int row, int column) Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position. int getPlayer() Returns the current player (BLACK or WHITE). int getSize() Returns the grid size. int getWinner() Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively. boolean isGameOver() Return whether or not the game is over. boolean playPiece(int row, int column) The current player tries to play a piece at the given position. java.lang.String toString() Returns a String representation of the board. Methods inherited from class java.lang.Object clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Field Detail BLACK public static final int BLACK a constant int value 1 indicating a black player/piece See Also:Constant Field Values WHITE public static final int WHITE a constant int value -1 indicating a white player/piece See Also:Constant Field Values NONE public static final int NONE a constant int value 0 indicating no player/piece See Also:Constant Field Values Constructor Detail Connect6State public Connect6State(int size) Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26. Parameters:size - game grid size Method Detail getPiece public int getPiece(int row, int column) Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position. Parameters:row - - valid grid position rowcolumn - - valid grid position columnReturns:the piece (BLACK, WHITE, or NONE) at position (row, column) getPlayer public int getPlayer() Returns the current player (BLACK or WHITE). Returns:the current player (BLACK or WHITE) getSize public int getSize() Returns the grid size. Returns:the grid size getWinner public int getWinner() Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively. A player wins by placing 6 or more pieces consecutively in a line horizontally, vertically, or diagonally. Returns:the winner BLACK, WHITE, or NONE (if there is no winner) playPiece public boolean playPiece(int row, int column) The current player tries to play a piece at the given position. If the move is illegal (e.g. out of bounds, already occupied), false is returned. Otherwise, the current player's piece is placed at the given row and column, the current player changes or doesn't change according to the game rules, and true is returned. The current player changes after the first play, and after every two plays of a player thereafter. Parameters:row - attempted play rowcolumn - attempted play columnReturns:whether or not the attempted play was legal toString public java.lang.String toString() Returns a String representation of the board. A '.' denotes an empty grid position. A '*' denotes a black piece. A 'o' denotes a white piece. Each grid row begins with a 1-based row number from 1 to size, right-justified within a width of 2 characters and followed by space-separated pieces of that row. Rows are printed in decreasing order, so that printed row 1 (internal grid row 0) is printed last. Under the board and aligned with each column, print successive lowercase letters 'a', 'b', 'c', ... until each column (of 26 columns max) is labeled with a letter. Thus, internal grid column number c is labeled by character (char) ('a' + c). Overrides:toString in class java.lang.Object isGameOver public boolean isGameOver() Return whether or not the game is over. Returns:whether or not the game is over
code Connect6State.java according to the following instructions Class Connect6State java.lang.Object Connect6State public class Connect6State extends java.lang.Object Connect6State - A representation of a Connect6 (http://en.wikipedia.org/wiki/Connect6) game state. We assume a square grid board size specified to be a positive integer less than or equal to 26. Size 19 is common. The first and second players place pieces on the board that are black and white, respectively. Each piece is placed in an unoccupied grid position. On the first turn, the first player places one piece. Afterwards, players alternate placing two pieces per turn. Play continues until either one player completes a consecutive line of 6 or more of their pieces or no play is possible. The player with 6 or more pieces in a horizontal, vertical, or diagonal line is the winner. If no legal play is possible, the game is a draw. Field Summary Fields Modifier and Type Field Description static int BLACK a constant int value 1 indicating a black player/piece static int NONE a constant int value 0 indicating no player/piece static int WHITE a constant int value -1 indicating a white player/piece Constructor Summary Constructors Constructor Description Connect6State(int size) Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26. Method Summary All MethodsInstance MethodsConcrete Methods Modifier and Type Method Description int getPiece(int row, int column) Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position. int getPlayer() Returns the current player (BLACK or WHITE). int getSize() Returns the grid size. int getWinner() Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively. boolean isGameOver() Return whether or not the game is over. boolean playPiece(int row, int column) The current player tries to play a piece at the given position. java.lang.String toString() Returns a String representation of the board. Methods inherited from class java.lang.Object clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Field Detail BLACK public static final int BLACK a constant int value 1 indicating a black player/piece See Also:Constant Field Values WHITE public static final int WHITE a constant int value -1 indicating a white player/piece See Also:Constant Field Values NONE public static final int NONE a constant int value 0 indicating no player/piece See Also:Constant Field Values Constructor Detail Connect6State public Connect6State(int size) Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26. Parameters:size - game grid size Method Detail getPiece public int getPiece(int row, int column) Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position. Parameters:row - - valid grid position rowcolumn - - valid grid position columnReturns:the piece (BLACK, WHITE, or NONE) at position (row, column) getPlayer public int getPlayer() Returns the current player (BLACK or WHITE). Returns:the current player (BLACK or WHITE) getSize public int getSize() Returns the grid size. Returns:the grid size getWinner public int getWinner() Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively. A player wins by placing 6 or more pieces consecutively in a line horizontally, vertically, or diagonally. Returns:the winner BLACK, WHITE, or NONE (if there is no winner) playPiece public boolean playPiece(int row, int column) The current player tries to play a piece at the given position. If the move is illegal (e.g. out of bounds, already occupied), false is returned. Otherwise, the current player's piece is placed at the given row and column, the current player changes or doesn't change according to the game rules, and true is returned. The current player changes after the first play, and after every two plays of a player thereafter. Parameters:row - attempted play rowcolumn - attempted play columnReturns:whether or not the attempted play was legal toString public java.lang.String toString() Returns a String representation of the board. A '.' denotes an empty grid position. A '*' denotes a black piece. A 'o' denotes a white piece. Each grid row begins with a 1-based row number from 1 to size, right-justified within a width of 2 characters and followed by space-separated pieces of that row. Rows are printed in decreasing order, so that printed row 1 (internal grid row 0) is printed last. Under the board and aligned with each column, print successive lowercase letters 'a', 'b', 'c', ... until each column (of 26 columns max) is labeled with a letter. Thus, internal grid column number c is labeled by character (char) ('a' + c). Overrides:toString in class java.lang.Object isGameOver public boolean isGameOver() Return whether or not the game is over. Returns:whether or not the game is over
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 10PE
Related questions
Question
code Connect6State.java according to the following instructions
Class Connect6State
- java.lang.Object
-
- Connect6State
- public class Connect6State extends java.lang.Object
Connect6State - A representation of a Connect6 (http://en.wikipedia.org/wiki/Connect6) game state. We assume a square grid board size specified to be a positive integer less than or equal to 26. Size 19 is common. The first and second players place pieces on the board that are black and white, respectively. Each piece is placed in an unoccupied grid position. On the first turn, the first player places one piece. Afterwards, players alternate placing two pieces per turn. Play continues until either one player completes a consecutive line of 6 or more of their pieces or no play is possible. The player with 6 or more pieces in a horizontal, vertical, or diagonal line is the winner. If no legal play is possible, the game is a draw.
-
-
Field Summary
FieldsModifier and Type Field Description static int BLACK a constant int value 1 indicating a black player/piecestatic int NONE a constant int value 0 indicating no player/piecestatic int WHITE a constant int value -1 indicating a white player/piece
-
Constructor Summary
ConstructorsConstructor Description Connect6State(int size) Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26.
-
Method Summary
All MethodsInstance MethodsConcrete MethodsModifier and Type Method Description int getPiece(int row, int column) Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position.int getPlayer() Returns the current player (BLACK or WHITE).int getSize() Returns the grid size.int getWinner() Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively.boolean isGameOver() Return whether or not the game is over.boolean playPiece(int row, int column) The current player tries to play a piece at the given position.java.lang.String toString() Returns a String representation of the board.-
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
-
-
-
-
Field Detail
-
BLACK
public static final int BLACKa constant int value 1 indicating a black player/pieceSee Also:Constant Field Values
-
WHITE
public static final int WHITEa constant int value -1 indicating a white player/pieceSee Also:Constant Field Values
-
NONE
public static final int NONEa constant int value 0 indicating no player/pieceSee Also:Constant Field Values
-
-
Constructor Detail
-
Connect6State
public Connect6State(int size)Creates Connect6State instance with an empty size-by-size grid and black to play, where size is assumed to be positive and less than or equal to 26.Parameters:size - game grid size
-
-
Method Detail
-
getPiece
public int getPiece(int row, int column)Given the row and column, returns the piece (BLACK, WHITE, or NONE) at that grid position.Parameters:row - - valid grid position rowcolumn - - valid grid position columnReturns:the piece (BLACK, WHITE, or NONE) at position (row, column)
-
getPlayer
public int getPlayer()Returns the current player (BLACK or WHITE).Returns:the current player (BLACK or WHITE)
-
getSize
public int getSize()Returns the grid size.Returns:the grid size
-
getWinner
public int getWinner()Returns BLACK, WHITE, or NONE, if black has won, white has won, or no player has won, respectively. A player wins by placing 6 or more pieces consecutively in a line horizontally, vertically, or diagonally.Returns:the winner BLACK, WHITE, or NONE (if there is no winner)
-
playPiece
public boolean playPiece(int row, int column)The current player tries to play a piece at the given position. If the move is illegal (e.g. out of bounds, already occupied), false is returned. Otherwise, the current player's piece is placed at the given row and column, the current player changes or doesn't change according to the game rules, and true is returned. The current player changes after the first play, and after every two plays of a player thereafter.Parameters:row - attempted play rowcolumn - attempted play columnReturns:whether or not the attempted play was legal
-
toString
public java.lang.String toString()Returns a String representation of the board. A '.' denotes an empty grid position. A '*' denotes a black piece. A 'o' denotes a white piece. Each grid row begins with a 1-based row number from 1 to size, right-justified within a width of 2 characters and followed by space-separated pieces of that row. Rows are printed in decreasing order, so that printed row 1 (internal grid row 0) is printed last. Under the board and aligned with each column, print successive lowercase letters 'a', 'b', 'c', ... until each column (of 26 columns max) is labeled with a letter. Thus, internal grid column number c is labeled by character (char) ('a' + c).Overrides:toString in class java.lang.Object
-
isGameOver
public boolean isGameOver()Return whether or not the game is over.Returns:whether or not the game is over
-
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
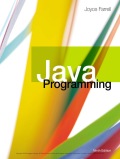
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
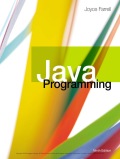
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT