Add a static variable to the top of your Person class: private static int numPersons = 0; Next, add two static methods to access and update the value of the numPersons variable: Write a static access method as follows: This accessor method is named getNumPersons It is a static method It returns an int The body of the method returns the value of the numPersons variable Write a static mutator method as follows: This mutator method is named updateNumPersons It is a static method It returns nothing The body of the method increments the value of numPersons by 1 Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file): /** * PersonTest.java, Activity 10.1 * @author * CIS 36B */
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
(Java)
Check to see if you get the correct final output!
You will use the following code from Person.java class:
import java.util.Scanner;
import java.io.*;
class Person {
publicStringname;
publicintage;
publicStringgender;
// add additional member
privateAddressaddress;
// default constructor
publicPerson(){
name="unkonwn";
age=0;
gender="NA";
address=newAddress();
}
// argument constructor
publicPerson(Stringname,intage,Stringgender,Addressaddress){
this.name=name;
this.age=age;
this.gender=gender;
this.address=address;
}
publicvoidgreeting(){
System.out.println("Hi, my name is " + name + "!");
}
publicvoidgreeting(StringotherName){
System.out.println("Hi, " + otherName + "!");
}
publicvoidsetName(Stringname){
this.name=name;
}
publicvoidsetAge(intage){
this.age=age;
}
publicvoidsetGender(Stringgender){
this.gender=gender;
}
publicStringgetName(){
returnthis.name;
}
publicintgetAge(){
returnthis.age;
}
publicStringgetGender(){
returnthis.gender;
}
publicAddressgetAddress(){
// fill in method body here
returnaddress;
}
publicvoidsetAddress(Addressa){
// fill in method body here
address=a;
}
@Override
publicStringtoString(){
return"Name: "+name+"\nAge: "+age+"\nGender: "+gender+"\nAddress: "+address;// fill in here!;
}
}
- Add a static variable to the top of your Person class:
- Next, add two static methods to access and update the value of the numPersons variable:
- Write a static access method as follows:
- This accessor method is named getNumPersons
- It is a static method
- It returns an int
- The body of the method returns the value of the numPersons variable
- Write a static mutator method as follows:
- This mutator method is named updateNumPersons
- It is a static method
- It returns nothing
- The body of the method increments the value of numPersons by 1
- Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file):
/**
* PersonTest.java, Activity 10.1
* @author
* CIS 36B
*/
import java.util.Scanner;
public class PersonTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String name, gender, street;
int age, number;
Person person1;
System.out.print("Welcome!\n\nEnter your name: ");
name = input.nextLine();
System.out.print("Enter your age: ");
age = input.nextInt();
System.out.print("Enter your gender: ");
gender = input.next();
System.out.print("Enter the street number of your address: ");
number = input.nextInt();
System.out.print("Enter the name of your street: ");
input.nextLine();
street = input.nextLine();
Address address = new Address(number, street);
person1 = new Person(name, age, gender, address);
Person.updateNumPersons();
System.out.println("\nYour Summary:\n" + person1);
Person person2 = new Person(person1);
person2.setName("C.J. Nguyen");
Address a = new Address(42, "South 3rd St");
person2.setAddress(a);
Person.updateNumPersons();
System.out.println("\nAnother student with your "
+ "same age and gender: \n" + person2);
System.out.println("\nTotal num persons: " + Person.getNumPersons());
input.close();
}
}
- When you get the correct output (as shown below), upload Address.java and Person.java to Canvas
Sample Output (Note that user input may vary):
Welcome!
Enter your name: Xing Li
Enter your age: 39
Enter your gender: M
Enter the street number of your address: 555
Enter the name of your street: W. Harlem Ave
Your Summary:
Name: Xing Li
Age: 39
Gender: M
Address: 555 W. Harlem Ave
Another student with your same age and gender:
Name: C.J. Nguyen
Age: 39
Gender: M
Address: 42 South 3rd St
Total num persons: 2

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

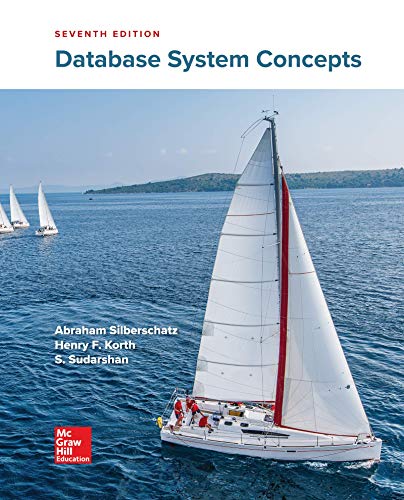
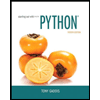
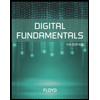
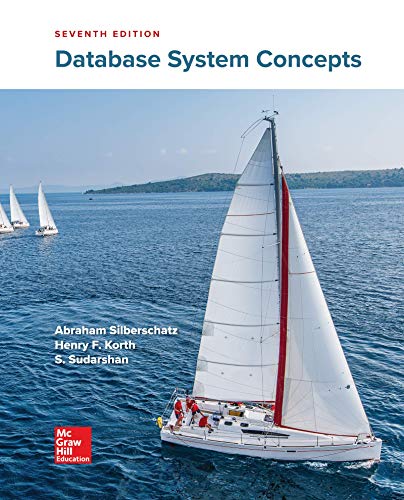
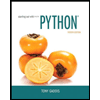
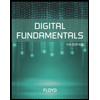
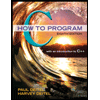
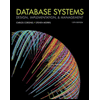
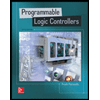